Java Abstract Classes
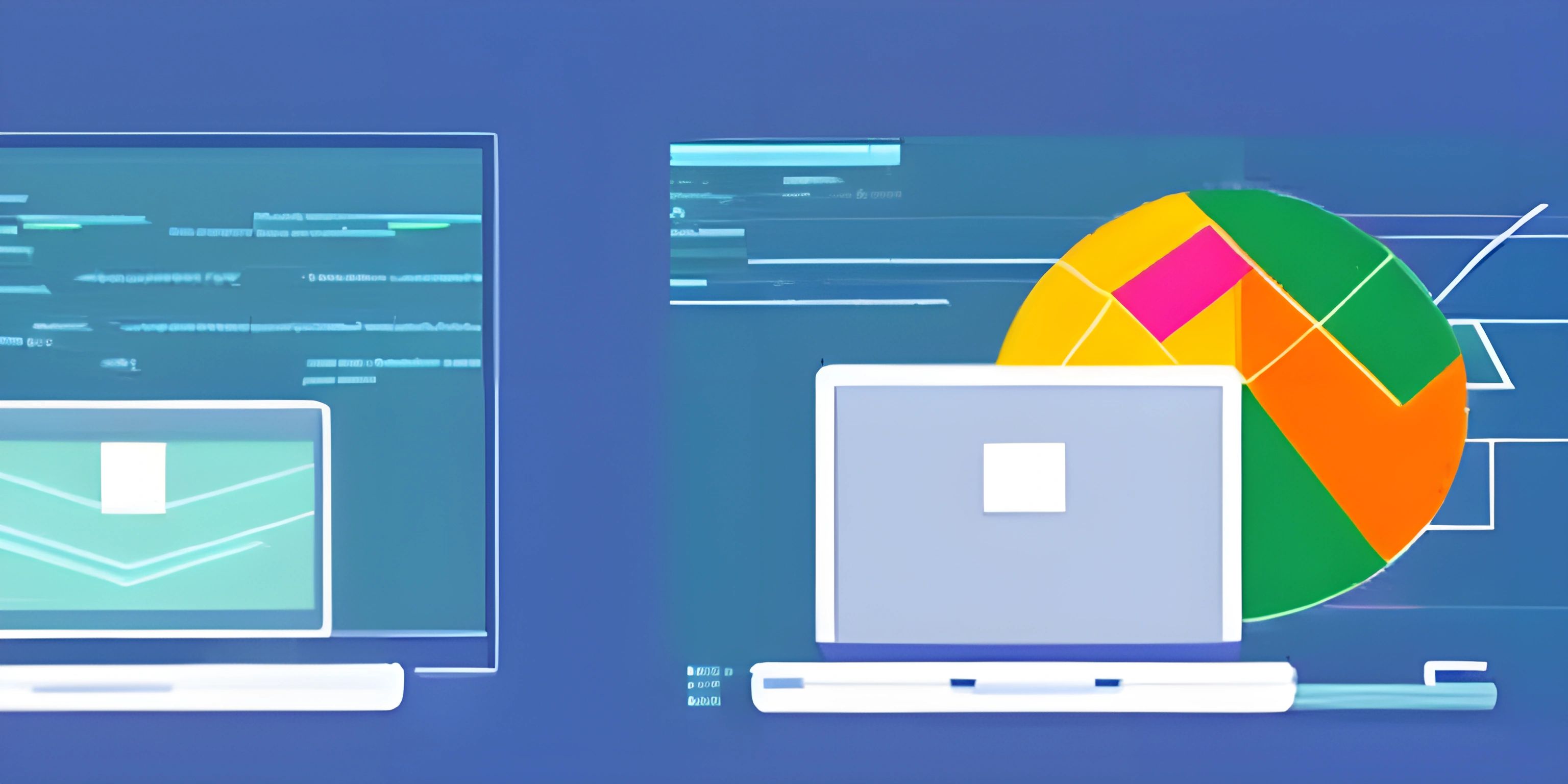
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Abstract classes in Java are essential tools for designing flexible and powerful code structures. They serve as a foundation for other classes to inherit from, allowing you to define common behaviors and properties for a group of related classes. By understanding abstract classes, you'll be better equipped to create clean and maintainable code.
Abstract Classes
An abstract class is a class that cannot be instantiated on its own but can serve as a base for other classes. It's like a blueprint for creating new classes with common attributes and behaviors. Abstract classes can contain both abstract and non-abstract methods.
Declaring Abstract Classes
To declare an abstract class in Java, you need to use the abstract
keyword before the class
keyword:
abstract class Animal { // class body }
Abstract Methods
Abstract methods are methods without any implementation inside the abstract class. They act as placeholders for methods that must be implemented in any non-abstract class that extends the abstract class. To declare an abstract method, use the abstract
keyword before the method signature and end the line with a semicolon:
abstract class Animal { abstract void makeSound(); }
Inheriting from Abstract Classes
To inherit from an abstract class, use the extends
keyword in the class declaration of the subclass:
class Dog extends Animal { // class body }
Implementing Abstract Methods
When a non-abstract class inherits from an abstract class, it must provide implementations for all abstract methods in the superclass:
class Dog extends Animal { @Override void makeSound() { System.out.println("The dog barks"); } }
Why Use Abstract Classes
Abstract classes are useful when you have a group of related classes that share some common behavior. By using an abstract class as a base, you can:
- Enforce a consistent structure: When you define abstract methods in an abstract class, you ensure that all subclasses must implement these methods.
- Promote code reusability: By providing common implementations for non-abstract methods in the abstract class, you can avoid duplicating code across multiple subclasses.
- Enhance maintainability: By centralizing shared behavior in an abstract class, you can more easily make changes that affect all subclasses.
Abstract Classes vs. Interfaces
While abstract classes and interfaces both provide a way to define common behaviors, there are some key differences:
- Abstract classes can have non-abstract methods with implementations, while interfaces can only have abstract methods.
- A class can extend only one abstract class, but it can implement multiple interfaces.
- Abstract classes can have fields (instance variables), while interfaces cannot.
In general, use abstract classes when you want to provide a common base for related classes with shared behavior. Use interfaces when you want to define a contract for unrelated classes that implement a specific set of methods.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is an abstract class?
An abstract class is a class that cannot be instantiated on its own but serves as a base for other classes. Abstract classes can contain both abstract (methods without implementation) and non-abstract methods, and they help enforce a consistent structure, promote code reusability, and enhance maintainability.
How do you declare an abstract class in Java?
To declare an abstract class in Java, use the abstract
keyword before the class
keyword. For example: abstract class Animal { /* class body */ }
.
How do you inherit from an abstract class in Java?
To inherit from an abstract class in Java, use the extends
keyword in the class declaration of the subclass. For example: class Dog extends Animal { /* class body */ }
.
What is the difference between abstract classes and interfaces in Java?
Both abstract classes and interfaces provide a way to define common behaviors, but there are key differences: abstract classes can have non-abstract methods with implementations and fields (instance variables), while interfaces can only have abstract methods and no fields. Also, a class can extend only one abstract class but can implement multiple interfaces.