Understanding Abstraction in Java and How to Implement It
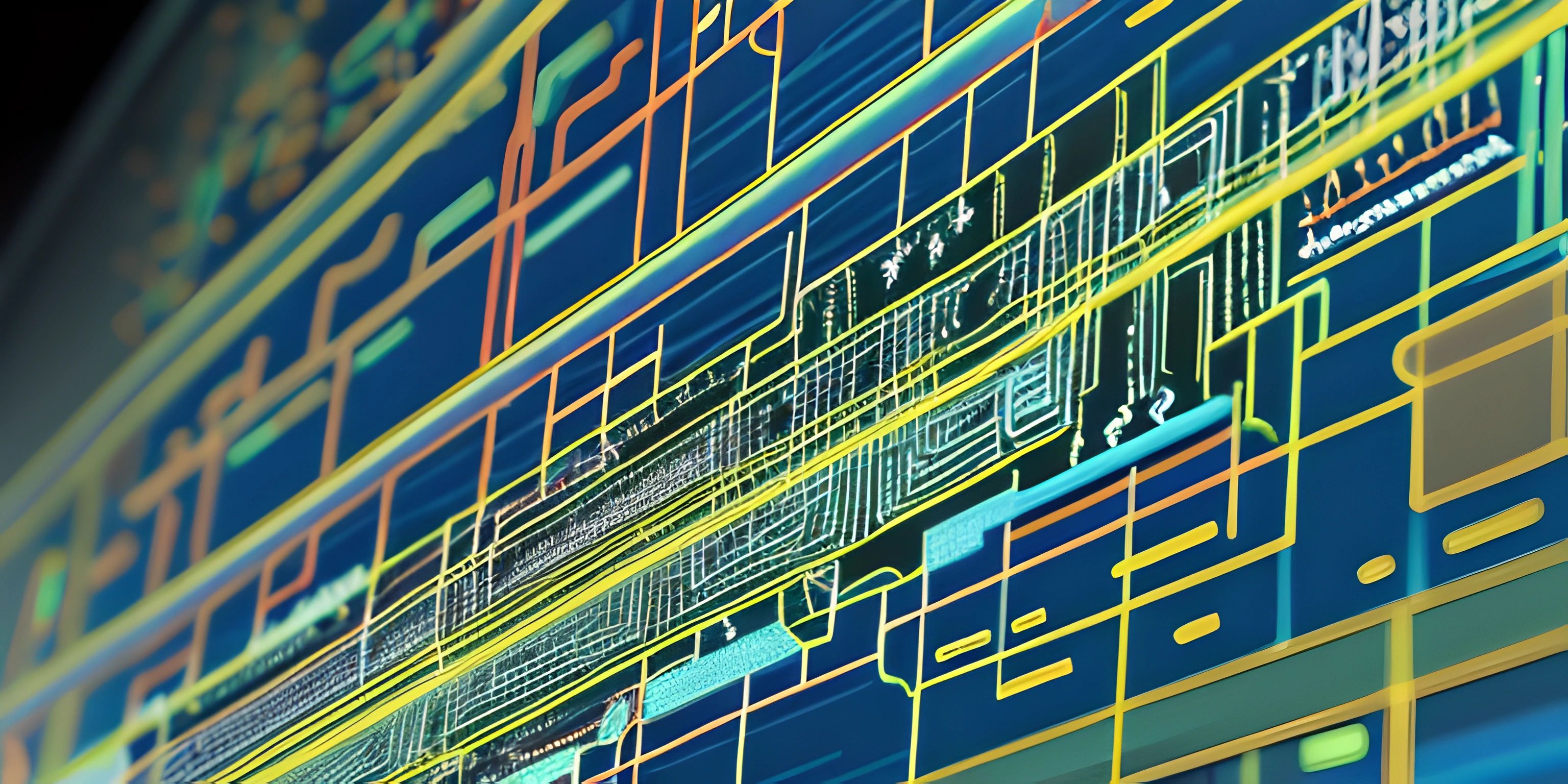
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Abstraction is a key concept in the world of object-oriented programming (OOP), and Java is no exception. It allows us to focus on the essential features of an object while ignoring the details. In other words, abstraction is all about generalizing and simplifying complex systems by breaking them down into manageable pieces.
Abstract Classes
An abstract class in Java is a blueprint for creating objects with common characteristics and behavior. It cannot be instantiated on its own, which means you can't create an object directly from an abstract class. Instead, other classes must inherit from the abstract class and provide implementations for any abstract methods it contains.
To create an abstract class, use the abstract
keyword:
abstract class Animal { abstract void makeSound(); }
Here, the Animal
class has an abstract method makeSound()
. Any class that inherits from Animal
must implement this method.
Extending Abstract Classes
To inherit from an abstract class, use the extends
keyword:
class Dog extends Animal { void makeSound() { System.out.println("Woof!"); } }
Now, Dog
implements the makeSound()
method from the Animal
abstract class. We can create a Dog
object and call the makeSound()
method:
public static void main(String[] args) { Dog myDog = new Dog(); myDog.makeSound(); // Output: Woof! }
Interfaces
Interfaces in Java are similar to abstract classes, but they provide a higher level of abstraction. They act as a contract that specifies a set of methods that a class must implement. In Java, a class can implement multiple interfaces, allowing for greater flexibility and code reusability.
To create an interface, use the interface
keyword:
interface Flyable { void fly(); }
The Flyable
interface contains a single method, fly()
.
Implementing Interfaces
To implement an interface, use the implements
keyword:
class Bird extends Animal implements Flyable { void makeSound() { System.out.println("Chirp!"); } void fly() { System.out.println("The bird is flying."); } }
The Bird
class now implements both the Animal
abstract class and the Flyable
interface, providing implementations for the makeSound()
and fly()
methods.
public static void main(String[] args) { Bird myBird = new Bird(); myBird.makeSound(); // Output: Chirp! myBird.fly(); // Output: The bird is flying. }
Conclusion
Abstraction is an essential concept in Java and other object-oriented programming languages. It helps to simplify complex systems by focusing on essential features and hiding the details. In Java, you can achieve abstraction through abstract classes and interfaces, allowing you to create flexible, reusable, and easy-to-maintain code. Dive deeper into Object-Oriented Programming in Java to further enhance your programming skills!