Introduction to JavaFX and Building Graphical Applications
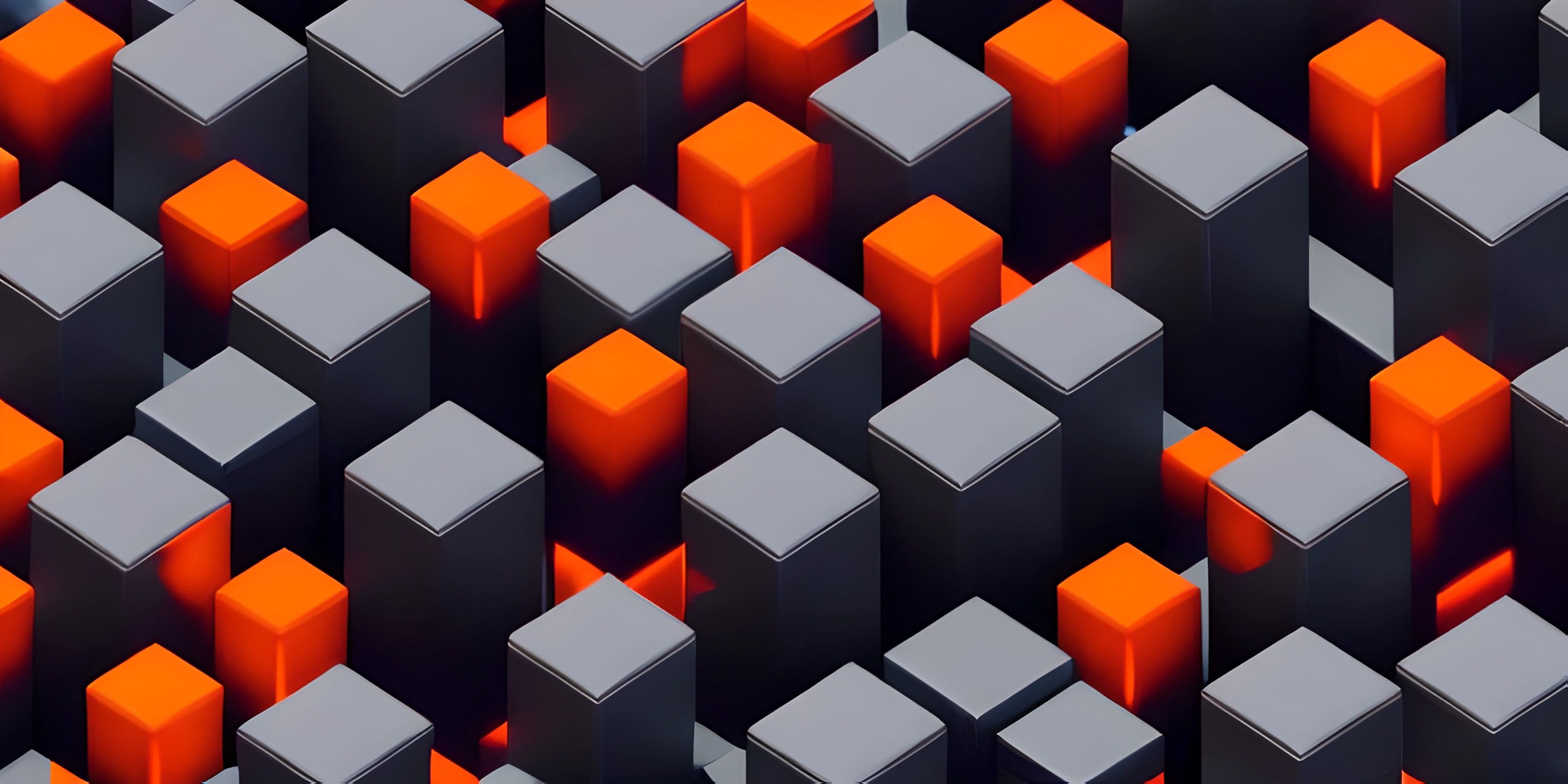
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JavaFX is a fantastic library that allows you to create rich, interactive user interfaces (UI) for your Java applications. With JavaFX, you can easily add graphical elements to your applications, making them more visually appealing and user-friendly. Say goodbye to dull, text-based console applications and hello to stunning, feature-rich graphical interfaces!
What is JavaFX?
JavaFX is a software platform for creating and delivering desktop, mobile, and embedded applications with a rich, immersive user experience. It is the successor to the older Java Swing library and provides a more modern, versatile, and efficient way of building graphical user interfaces.
JavaFX comes with a wide array of built-in components, such as buttons, text fields, tables, and charts, that you can use to create powerful and visually appealing applications. It also supports CSS styling, allowing you to customize the appearance of your UI elements easily.
Getting Started with JavaFX
To start developing JavaFX applications, you'll need to have the Java Development Kit (JDK) installed, preferably JDK 11 or later. JavaFX is no longer part of the standard JDK distribution since JDK 11, so you'll need to download the JavaFX SDK separately from the OpenJFX website.
Once you have the JDK and JavaFX SDK installed, you can start creating your first JavaFX application. Let's take a look at the basic structure of a simple JavaFX application.
Basic Structure of a JavaFX Application
A JavaFX application typically consists of the following components:
-
Main class: This is the entry point of your application and extends the
javafx.application.Application
class. It is responsible for initializing and launching the JavaFX application. -
Stage: The primary container for your JavaFX application's window, also known as the top-level container. It is an instance of the
javafx.stage.Stage
class. -
Scene: A container for all the UI elements in your application, such as buttons, text fields, and images. It is an instance of the
javafx.scene.Scene
class. -
UI elements: The components that make up your application's user interface, also known as nodes. These can be basic UI elements like buttons and text fields or more complex elements like tables and charts.
Here's a simple JavaFX application that displays a "Hello, JavaFX!" message in a window:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class HelloWorld extends Application { @Override public void start(Stage primaryStage) { Label helloLabel = new Label("Hello, JavaFX!"); StackPane root = new StackPane(helloLabel); Scene scene = new Scene(root, 300, 200); primaryStage.setTitle("Hello World"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
In this example, we create a Label
with the text "Hello, JavaFX!", add it to a StackPane
layout, and set the layout as the root of a Scene
. Finally, we set the scene on the Stage
and display the window using the show()
method.
Building Graphical Applications with JavaFX
Now that you have a basic understanding of JavaFX, you can start exploring its rich set of features to create more complex graphical applications. Some of the key aspects to consider when building a JavaFX application include:
-
Layouts: JavaFX provides various layout containers such as
HBox
,VBox
,GridPane
, andBorderPane
to help you organize and arrange your UI elements. -
Event handling: To make your application interactive, you'll need to handle user input events like button clicks, mouse movements, and key presses. JavaFX uses a clean and simple event handling model based on event listeners and event handlers.
-
Binding and properties: JavaFX has a powerful binding and properties framework that allows you to create dynamic, data-driven UI elements that automatically update when their underlying data changes.
-
Animations and effects: To create visually engaging applications, JavaFX provides a rich set of animations and special effects like fade, rotate, and blur that you can apply to your UI elements.
-
FXML and Scene Builder: FXML is an XML-based language for defining the structure of your JavaFX UI. It allows you to separate the UI design from the application logic, making your code cleaner and easier to maintain. JavaFX Scene Builder is a graphical tool for creating and editing FXML files.
As you explore JavaFX further, you'll undoubtedly discover its endless possibilities for creating stunning and feature-rich graphical applications. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Websites (psst, it's free!).
FAQ
What is JavaFX and why should I use it?
JavaFX is a powerful library that enables developers to create rich and interactive user interfaces for Java applications. It provides a wide range of built-in UI components, along with advanced features like animations, CSS styling, and 3D rendering. With JavaFX, you can create visually appealing applications that are also highly functional and responsive.
How do I get started with JavaFX?
To get started with JavaFX, you need to have Java Development Kit (JDK) installed on your machine. Once you have JDK installed, you can set up a JavaFX project in your favorite Integrated Development Environment (IDE), such as IntelliJ IDEA or Eclipse. You'll need to include the JavaFX library in your project's classpath and then start building your JavaFX application. Here's a simple example of a JavaFX "Hello, World!" application:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class HelloWorld extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { Label helloLabel = new Label("Hello, World!"); StackPane root = new StackPane(); root.getChildren().add(helloLabel); primaryStage.setScene(new Scene(root, 300, 250)); primaryStage.setTitle("JavaFX - Hello World"); primaryStage.show(); } }
What are the main components of a JavaFX application?
The main components of a JavaFX application include:
- Application: The entry point of your JavaFX application, extending the
javafx.application.Application
class. - Stage: The main window or frame of your application.
- Scene: A container for all the visual elements in your application.
- UI Controls: The interactive components like buttons, text fields, and sliders.
- Layouts: The containers that help organize and position UI controls in the application.
How do I style my JavaFX application?
JavaFX supports CSS styling to customize the appearance of your application. You can create a CSS file with the desired styles and load it into your application using the following code:
scene.getStylesheets().add(getClass().getResource("yourStylesheet.css").toExternalForm());
In your CSS file, you can define styles for various UI components, like this:
.button { -fx-background-color: #4CAF50; -fx-text-fill: white; -fx-padding: 10px; }
Can I create 3D graphics in JavaFX?
Yes, JavaFX includes support for 3D graphics through its built-in 3D shapes and APIs. You can create 3D scenes, render 3D objects, apply transformations, and even add lighting and camera effects. To get started with 3D graphics in JavaFX, you can use classes like javafx.scene.shape.Box
, javafx.scene.shape.Cylinder
, javafx.scene.shape.Sphere
, and many more.