Building Interactive UIs with ReactJS
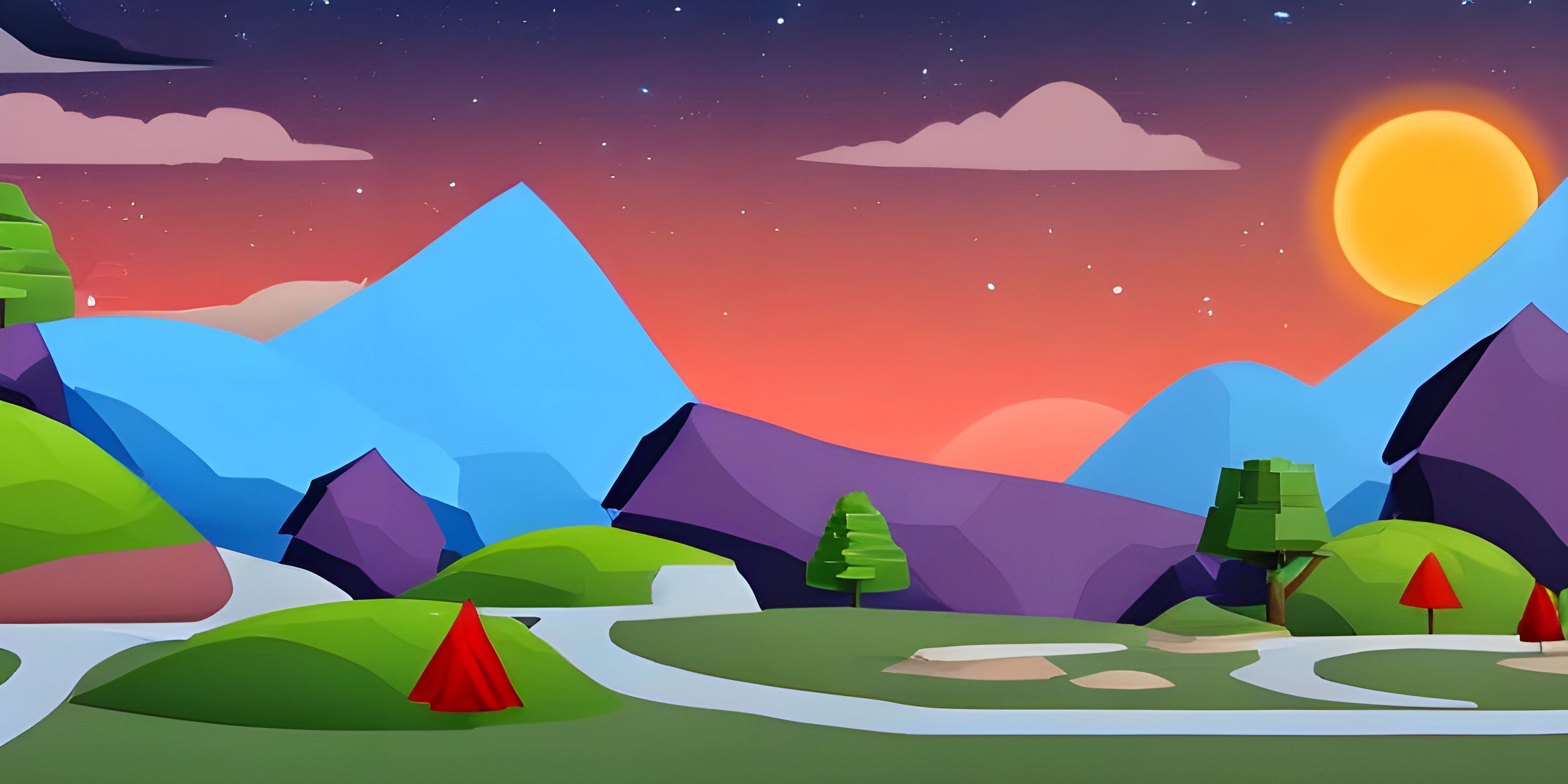
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
ReactJS, a JavaScript library for building user interfaces, is like the Swiss Army Knife of the modern web development toolkit. It allows you to chop, slice, and dice your way through complex UI problems with ease. So, buckle up, let's take a deep dive into the world of ReactJS and interactive UIs!
Setting the ReactJS Stage
Before we start creating masterpieces, we need to understand what ReactJS is. Simply put, ReactJS is a JavaScript library developed by Facebook to build user interfaces. It's like the paint, and the browser is our canvas.
Building Blocks: Components
Imagine building a house with Lego blocks. In ReactJS, we build interfaces with components. Each component is a reusable piece of UI, and together, they form complex user interfaces. It's kind of like assembling a puzzle, but a lot more fun!
function Welcome(props) { return <h1>Hello, {props.name}</h1>; } const element = <Welcome name="John Doe" />; ReactDOM.render( element, document.getElementById('root') );
In our snippet above, Welcome
is a component that we can reuse throughout our application. We just need to pass in the name
prop to customize it. React's component-based structure keeps our code DRY (Don't Repeat Yourself) and maintainable.
Props and State: The Heart of React
Props and state are the lifeblood of a React component. Think of them as the strings that control our React puppet.
Props (short for properties) in React are used to pass data from parent components down to child components.
The state, on the other hand, is data that is local or private to the component and allows the component to change and react to user interactions.
class Toggle extends React.Component { constructor(props) { super(props); this.state = {isToggleOn: true}; // This binding is necessary to make `this` work in the callback this.handleClick = this.handleClick.bind(this); } handleClick() { this.setState(state => ({ isToggleOn: !state.isToggleOn })); } render() { return ( <button onClick={this.handleClick}> {this.state.isToggleOn ? 'ON' : 'OFF'} </button> ); } }
In our Toggle
component above, we're using state to handle button clicks. When the user clicks the button, the state changes, causing the component to re-render with the new state. It's React magic in action!
Remember, in the world of React, props flow down, and state lives within.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is ReactJS?
ReactJS is a JavaScript library developed by Facebook for building user interfaces. It allows you to build complex UIs using reusable components and makes managing state in your application a breeze.
What are components in ReactJS?
Components are the building blocks of a ReactJS application. Each component represents a piece of UI and can be reused throughout the application. Components are also responsible for rendering the UI and handling user interaction.
What are props and state in ReactJS?
Props and state are the core concepts that enable dynamic and interactive components in ReactJS. Props are used to pass data from parent components to child components, while the state is used for data that is local or private to a component. The state can also change over time, typically in response to user interaction.
How does ReactJS handle user interactions?
ReactJS handles user interactions through event handlers, such as onClick, onMouseOver, etc., defined in components. When these events are triggered by user interaction, ReactJS updates the component's state, causing the component to re-render with the new state, thus providing a dynamic and interactive user experience.
Can I use ReactJS for mobile application development?
Yes, with React Native, a separate framework that uses ReactJS principles, you can create mobile applications for both Android and iOS. However, React Native is not the same as ReactJS, which is primarily for web development.