Exploring Imports in Java
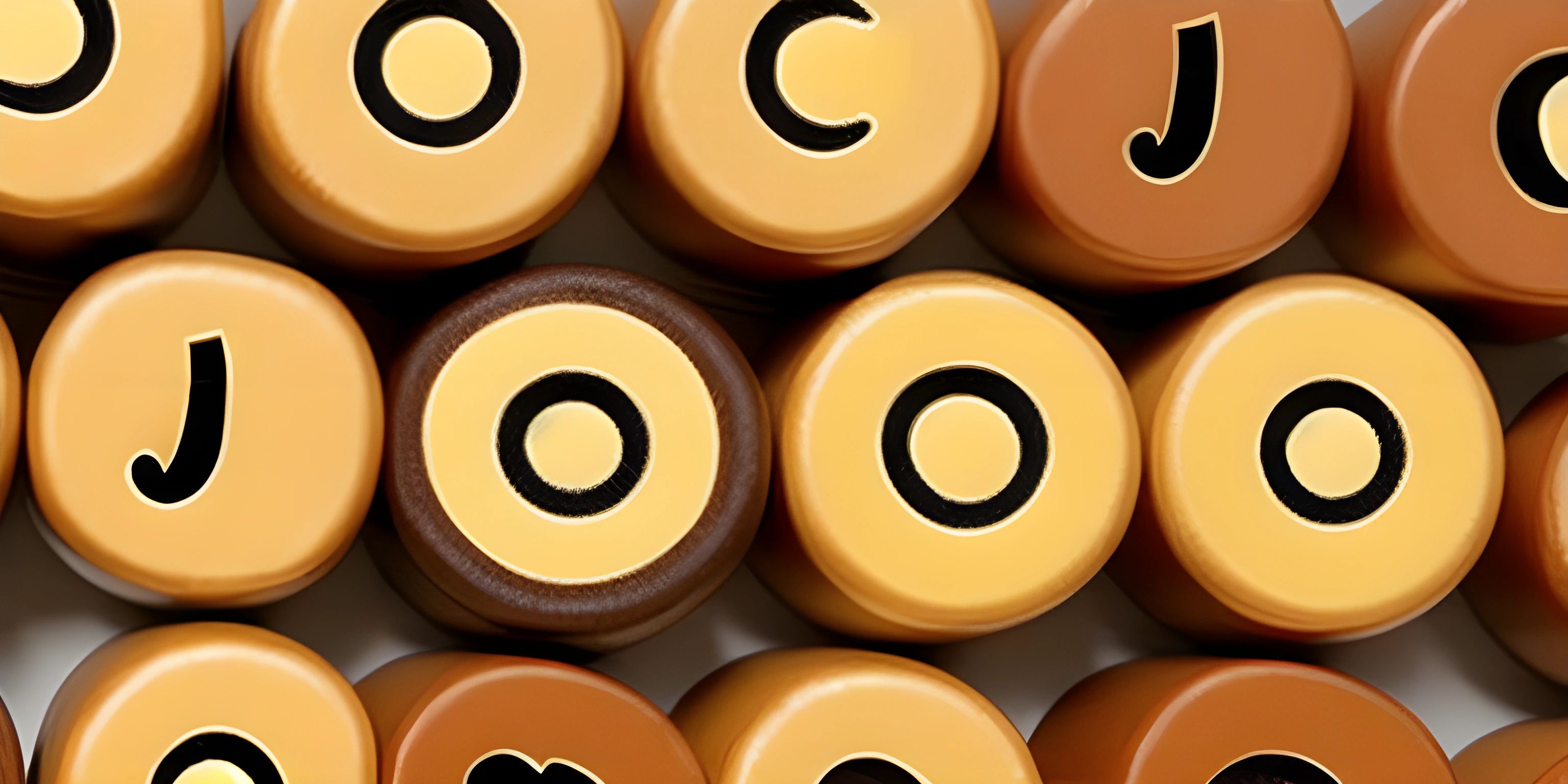
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java is a vast programming language with countless classes and libraries. To keep things organized and efficient, we don't want to cram all the classes into one place. That's where imports come into play. Imports are like little helpers that bring in specific classes or entire packages when we need them. So let's embark on an exploration of Java imports and learn how to use them effectively.
Purpose of Java Imports
Imagine you're a chef preparing a meal. Your kitchen is full of ingredients, but you don't want to clutter your countertop with everything at once. Instead, you grab the specific ingredients you need for your dish. Java imports work the same way. They allow you to bring in only the classes or packages you need for your code, keeping your program neat and efficient.
Using imports in Java helps you to:
- Avoid the clutter of unnecessary classes.
- Save memory by importing only what you need.
- Make your code more readable and maintainable.
Java Import Syntax
Now that we understand the purpose of imports, let's dive into the syntax. The import statement is placed at the beginning of your Java file, right after the package statement (if any). The syntax looks like this:
import packageName.className;
Or, to import an entire package:
import packageName.*;
For example, let's say we want to use Java's ArrayList
class, which is part of the java.util
package. We'd write:
import java.util.ArrayList;
With this import statement, we can now create an ArrayList
object without writing the full package name every time.
Static Imports
Java also supports static imports, which allow you to import static members of a class. With static imports, you can directly access the static members without needing to specify the class name. The syntax is:
import static packageName.className.staticMember;
For example, let's import the Math
class's constant PI
:
import static java.lang.Math.PI;
Now you can use PI
directly in your code without the Math
prefix.
Tips for Using Imports
- Keep your imports organized: Group related imports together and use comments to describe their purpose.
- Avoid using wildcard imports (
*
): They can lead to naming conflicts and make your code harder to read. Explicitly importing specific classes shows exactly what your code depends on. - Use an Integrated Development Environment (IDE): Modern IDEs can automatically manage imports for you and suggest the necessary imports when you use a class.
Now you're ready to navigate the world of Java imports like a seasoned explorer. Bon voyage!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the purpose of imports in Java?
Imports in Java serve to bring in specific classes or entire packages when needed. They help avoid clutter, save memory, and make code more readable and maintainable.
How do you import a specific class in Java?
To import a specific class in Java, use the import statement followed by the package name and class name, like this: import packageName.className;
.
What is a static import in Java?
A static import in Java allows you to import static members of a class, enabling you to access them directly without specifying the class name. The syntax is: import static packageName.className.staticMember;
.
How can I organize my imports effectively?
To organize imports effectively, group related imports together, use comments to describe their purpose, avoid using wildcard imports (*
), and make use of an Integrated Development Environment (IDE) to manage imports automatically.