Java API: An Exploration
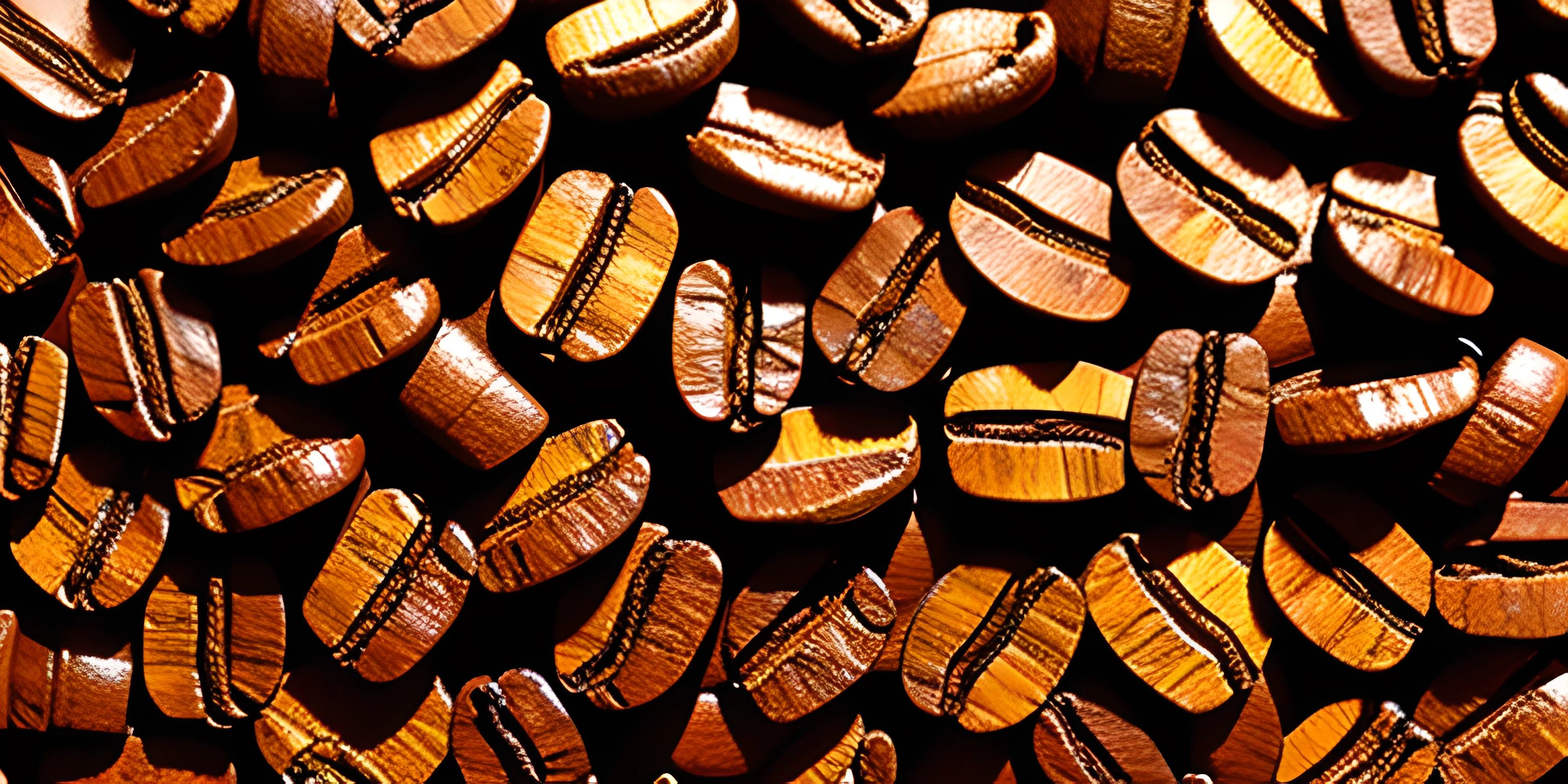
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The Java API is like an enormous toolbox for Java developers, filled with a plethora of classes and libraries that can be wielded to craft elegant and efficient solutions. From data manipulation to web services, the Java API has got you covered. Let's explore this treasure trove and learn how to make the best use of it.
What is the Java API?
The Java API (Application Programming Interface) is a collection of pre-built classes and methods that provide a multitude of functionalities for developers to use in their Java programs. It's part of the Java Standard Edition (SE) and is maintained and updated by Oracle. The API is organized into packages which contain related classes and interfaces.
Packages
Packages in the Java API help to group related classes and interfaces, making it easier for developers to find and use them. The package names usually follow a reverse domain naming convention:
com.example.project
This helps to avoid naming conflicts and ensures uniqueness. Some common packages in the Java API include:
java.lang
: This package contains the essential classes for the Java programming language, such asString
,Object
, andMath
.java.util
: This package offers a wide array of utility classes and interfaces, includingArrayList
,HashSet
, andHashMap
.java.io
: The input/output package provides classes for reading and writing streams of data, such asFileInputStream
,FileOutputStream
, andBufferedReader
.
Using the Java API
To employ the classes and methods available in the Java API, you need to import the relevant packages in your code. Once imported, you can effortlessly create objects and call methods from the API.
Importing Packages
To use a package from the Java API, you need to import it using an import statement. The import statement should be placed at the beginning of your Java file, right after the package declaration (if any).
For example, to use the ArrayList
class from the java.util
package, you'd import it like this:
import java.util.ArrayList;
To import an entire package, use the wildcard *
:
import java.util.*;
Creating Objects and Calling Methods
Once you've imported the necessary packages, you can create objects and call methods from the Java API. Let's say we want to create an ArrayList
object and add some elements to it:
import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> myList = new ArrayList<>(); myList.add("Apple"); myList.add("Banana"); myList.add("Cherry"); System.out.println(myList); } }
In this example, we first imported the ArrayList
class from the java.util
package. Then, we created an ArrayList
object called myList
and used the add
method to insert elements into it. Finally, we printed the contents of myList
using the System.out.println
method.
Exploring the Java API Documentation
When working with the Java API, it's crucial to be familiar with the official documentation. The documentation contains a wealth of information on the classes, interfaces, and methods included in the Java API, with detailed explanations and usage examples.
To effectively navigate the documentation, follow these steps:
- Locate the package you're interested in within the list of packages.
- Click on the package name to view the available classes and interfaces.
- Click on a class or interface name to access its detailed documentation.
As you explore the Java API, you'll discover that it offers vast potential for creating powerful applications. The key is to become familiar with the available classes and libraries, practice using them, and refer to the documentation whenever needed. With time and experience, you'll become a Java API virtuoso, ready to tackle any challenge that comes your way.
FAQ
What is the Java API and why is it important?
The Java API (Application Programming Interface) is a vast collection of pre-built classes, interfaces, and methods that allow developers to quickly and efficiently build Java applications. It's important because it provides a standardized way to access and use various functionalities, saving developers time and effort by not having to reinvent the wheel for common tasks.
How can I access the Java API documentation?
You can access the official Java API documentation online at the following URL: Java API Documentation Here, you'll find detailed information about all the classes, interfaces, and methods available in the Java API, along with code examples and explanations.
How do I import and use a class from the Java API in my code?
To use a class from the Java API in your code, you first need to import it. You do this by adding an import
statement at the beginning of your Java file, followed by the full name of the class you want to use. For example, if you want to use the ArrayList
class from the java.util
package, you would add the following line at the beginning of your file:
import java.util.ArrayList;
After importing the class, you can create objects of that class and use its methods in your code.
Can you give an example of using a Java API class in a program?
Sure! Let's say you want to create a list of strings using the ArrayList
class from the Java API. Here's a simple example of how to do that:
import java.util.ArrayList; public class JavaAPIExample { public static void main(String[] args) { ArrayList<String> myList = new ArrayList<String>(); myList.add("Hello"); myList.add("World"); myList.add("Java API"); for (String str : myList) { System.out.println(str); } } }
In this example, we first import the ArrayList
class from the java.util
package. Then, inside our main
method, we create an ArrayList
object called myList
and add three strings to it. Finally, we use a for-each loop to iterate through the list and print each string.
Are there any alternative resources to learn about the Java API?
Absolutely! In addition to the official Java API documentation, there are numerous books, tutorials, and online courses available that cover various aspects of the Java API. Websites like Stack Overflow, Reddit, and various Java programming forums are also great places to ask questions and discuss the Java API with fellow developers.