Reversing Strings and Traversing Arrays in Reverse Using JavaScript
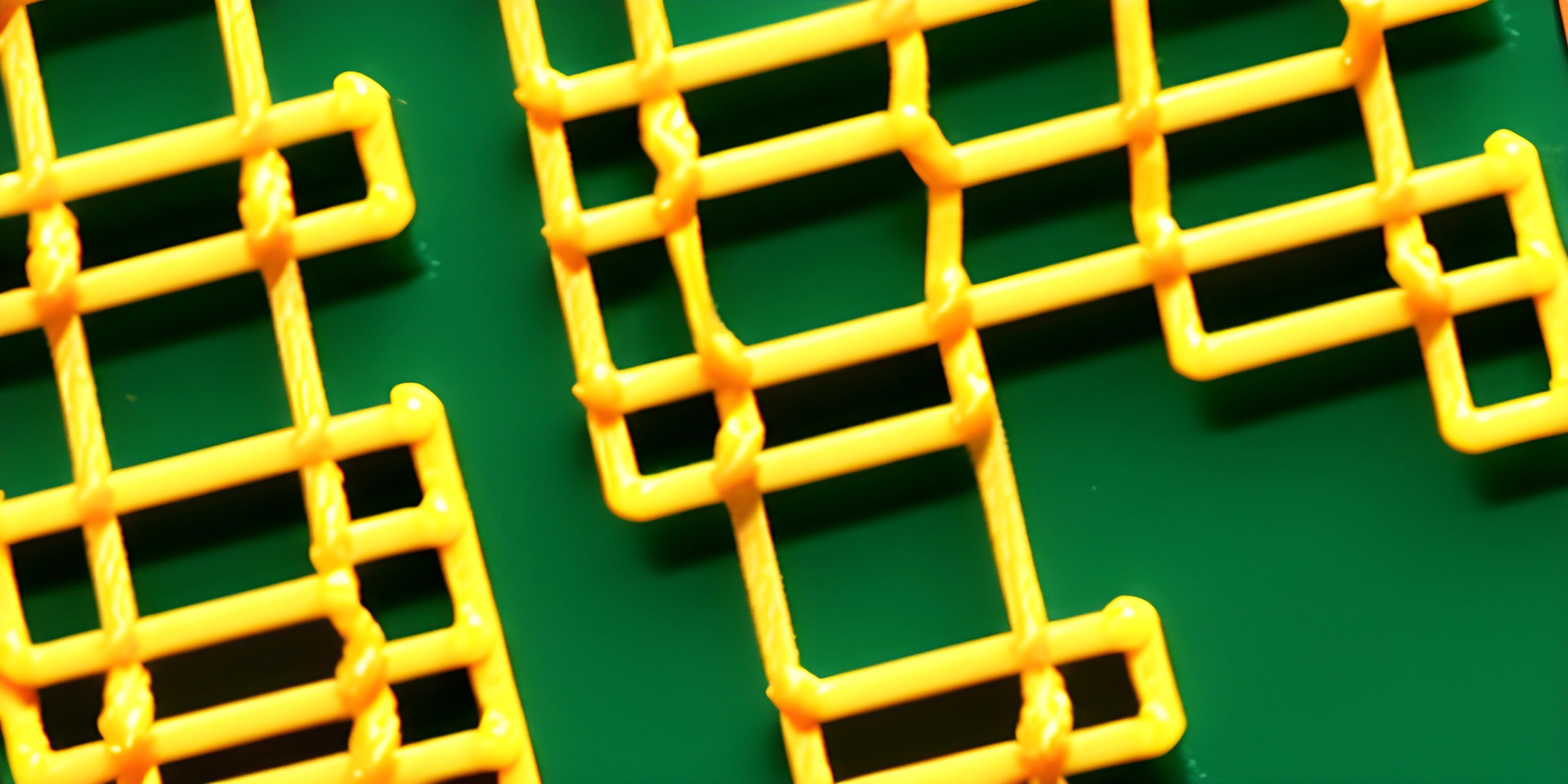
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When working with JavaScript, it's not uncommon to find yourself needing to reverse a string or traverse an array in backwards order. Whether it's for a coding challenge, a fun text effect, or some complex data manipulation, the ability to reverse your way through strings and arrays can be a handy tool to have in your developer's utility belt.
Reversing Strings
In JavaScript, strings are not inherently reversible. They do not have a built-in .reverse()
method like arrays do. But don't worry, we have three simple steps to reverse a string.
-
Split the string into an array of characters. The
.split('')
method turns our string into an array of individual characters.let str = "hello"; let strArray = str.split(''); // ["h", "e", "l", "l", "o"]
-
Reverse the array. Now, we can use the
.reverse()
method, which does exist for arrays.let reversedArray = strArray.reverse(); // ["o", "l", "l", "e", "h"]
-
Join the reversed array back into a string. The
.join('')
method takes an array and turns it back into a string.let reversedStr = reversedArray.join(''); // "olleh"
Voila! Your string is reversed. You can even chain these methods together for a one-liner solution.
let reversedStr = "hello".split('').reverse().join(''); // "olleh"
Traversing Arrays in Reverse
Sometimes, you might need to traverse an array in reverse order. You can do this with a simple for loop and a bit of creative indexing.
Normally when we loop through an array, we start at index 0 and increment our index until we reach the end. To traverse in reverse, we start at the end of the array (index array.length - 1
) and decrement our index until we reach 0.
Here's an example:
let arr = [1, 2, 3, 4, 5]; for(let i = arr.length - 1; i >= 0; i--) { console.log(arr[i]); // 5, 4, 3, 2, 1 }
Remember, array indices start at 0, so the last element in an array is at index array.length - 1
, not array.length
.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Reverse Word Splitter (psst, it's free!).
FAQ
How can I reverse a string in JavaScript?
You can reverse a string in JavaScript by first converting it to an array using the .split('')
method, reversing the array using the .reverse()
method, and then converting it back to a string using the .join('')
method.
How do I traverse an array in reverse order in JavaScript?
To traverse an array in reverse order in JavaScript, you can use a for loop that starts at the last index of the array (which is array.length - 1
) and decrements the index until it reaches 0.
Why doesn't JavaScript have a built-in method to reverse strings?
JavaScript treats strings as primitive and immutable data types, which means they cannot be directly modified. That's why there's no built-in reverse method for strings. However, we can convert the string to an array, reverse the array, and then convert it back to a string.
Can I combine the steps to reverse a string into one line in JavaScript?
Yes, you can combine all the steps into one line using method chaining. For example: "hello".split('').reverse().join('')
will reverse the string "hello".