Working with Strings and Arrays in JavaScript
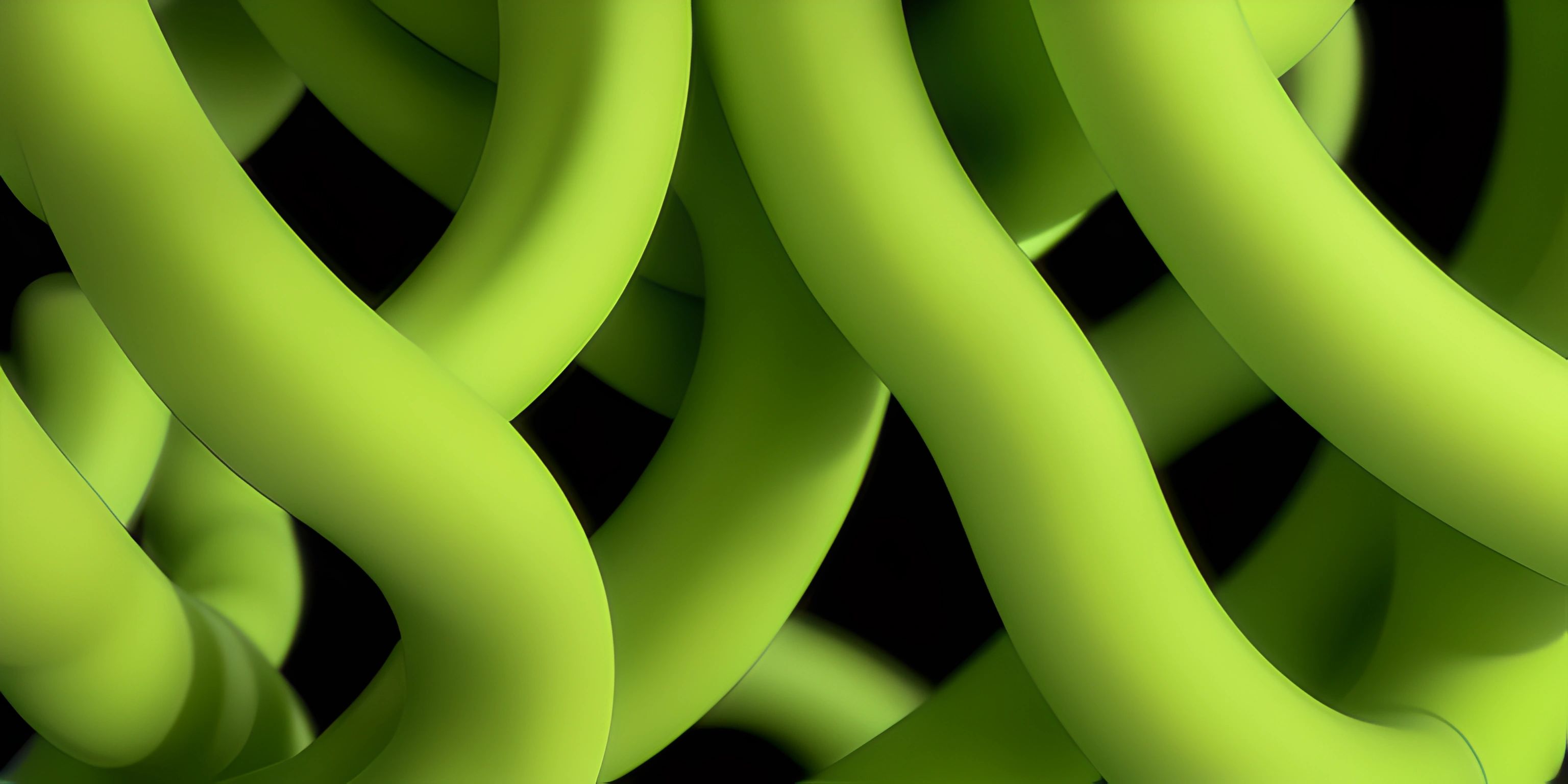
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JavaScript is a versatile programming language that can run on a variety of platforms. In this article, we'll focus on working with strings and arrays in JavaScript using Node.js. Node.js allows us to run JavaScript on the server-side, making it a popular choice for web development.
Strings in JavaScript
A string is a sequence of characters. In JavaScript, strings can be created using double quotes ("
) or single quotes ('
). However, we prefer double quotes where possible. Here's an example of creating a string:
let message = "Hello, Cratecode!"; console.log(message); // Output: Hello, Cratecode!
Common String Operations
- Concatenation: Combining two or more strings.
let firstName = "John"; let lastName = "Doe"; let fullName = firstName + " " + lastName; console.log(fullName); // Output: John Doe
- Length: Finding the length of a string.
let text = "Cratecode"; console.log(text.length); // Output: 9
- Indexing: Accessing a specific character in a string.
let text = "Cratecode"; console.log(text[0]); // Output: C
Arrays in JavaScript
An array is a collection of elements, such as numbers, strings, or other objects. Arrays are created using square brackets ([]
). Here's an example of creating an array:
let colors = ["red", "green", "blue"]; console.log(colors); // Output: [ 'red', 'green', 'blue' ]
Common Array Operations
- Length: Finding the length of an array.
let colors = ["red", "green", "blue"]; console.log(colors.length); // Output: 3
- Indexing: Accessing a specific element in an array.
let colors = ["red", "green", "blue"]; console.log(colors[0]); // Output: red
- Adding elements: Adding an element to the end of an array.
let colors = ["red", "green", "blue"]; colors.push("yellow"); console.log(colors); // Output: [ 'red', 'green', 'blue', 'yellow' ]
- Removing elements: Removing the last element from an array.
let colors = ["red", "green", "blue"]; colors.pop(); console.log(colors); // Output: [ 'red', 'green' ]
- Iterating over elements: Looping through each element in an array.
let colors = ["red", "green", "blue"]; colors.forEach(function(color) { console.log(color); }); // Output: // red // green // blue
These are just a few examples of working with strings and arrays in JavaScript using Node.js. As you continue to learn and practice, you'll discover many more methods and techniques for manipulating and working with these data types.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: File I/O (Reading) (psst, it's free!).
FAQ
What are some common JavaScript string methods?
Some common JavaScript string methods include:
charAt()
: Returns the character at the specified index.concat()
: Joins two or more strings.indexOf()
: Returns the index of the first occurrence of a specified value in a string.replace()
: Replaces a specified value with another value in a string.slice()
: Extracts a part of a string and returns a new string.split()
: Splits a string into an array of substrates.toUpperCase()
: Converts a string to uppercase letters.toLowerCase()
: Converts a string to lowercase letters.
How do you create an array in JavaScript?
To create an array in JavaScript, you can use the following syntax:
let myArray = ["element1", "element2", "element3"];
You can also create an array using the Array
constructor:
let myArray = new Array("element1", "element2", "element3");
What are some common JavaScript array methods?
Some common JavaScript array methods include:
concat()
: Joins two or more arrays and returns a new array.indexOf()
: Returns the first index at which a given element can be found in the array, or -1 if it is not present.join()
: Joins all elements of an array into a string.pop()
: Removes the last element from an array and returns that element.push()
: Adds one or more elements to the end of an array and returns the new length of the array.shift()
: Removes the first element from an array and returns that element.unshift()
: Adds one or more elements to the beginning of an array and returns the new length of the array.splice()
: Adds and/or removes elements from an array.slice()
: Extracts a section of an array and returns a new array.reverse()
: Reverses the order of the elements of an array in place.sort()
: Sorts the elements of an array in place and returns the array.
How do I access elements in a JavaScript array?
To access elements in a JavaScript array, you can use index notation:
let myArray = ["apple", "banana", "cherry"]; console.log(myArray[0]); // Outputs: "apple" console.log(myArray[1]); // Outputs: "banana" console.log(myArray[2]); // Outputs: "cherry"
Remember that the index starts at 0 for the first element.
How can I find the length of a JavaScript array or string?
To find the length of a JavaScript array or string, you can use the .length
property:
let myArray = ["apple", "banana", "cherry"]; console.log(myArray.length); // Outputs: 3 let myString = "Hello, world!"; console.log(myString.length); // Outputs: 13