Using Regular Expressions for Pattern Matching in JavaScript
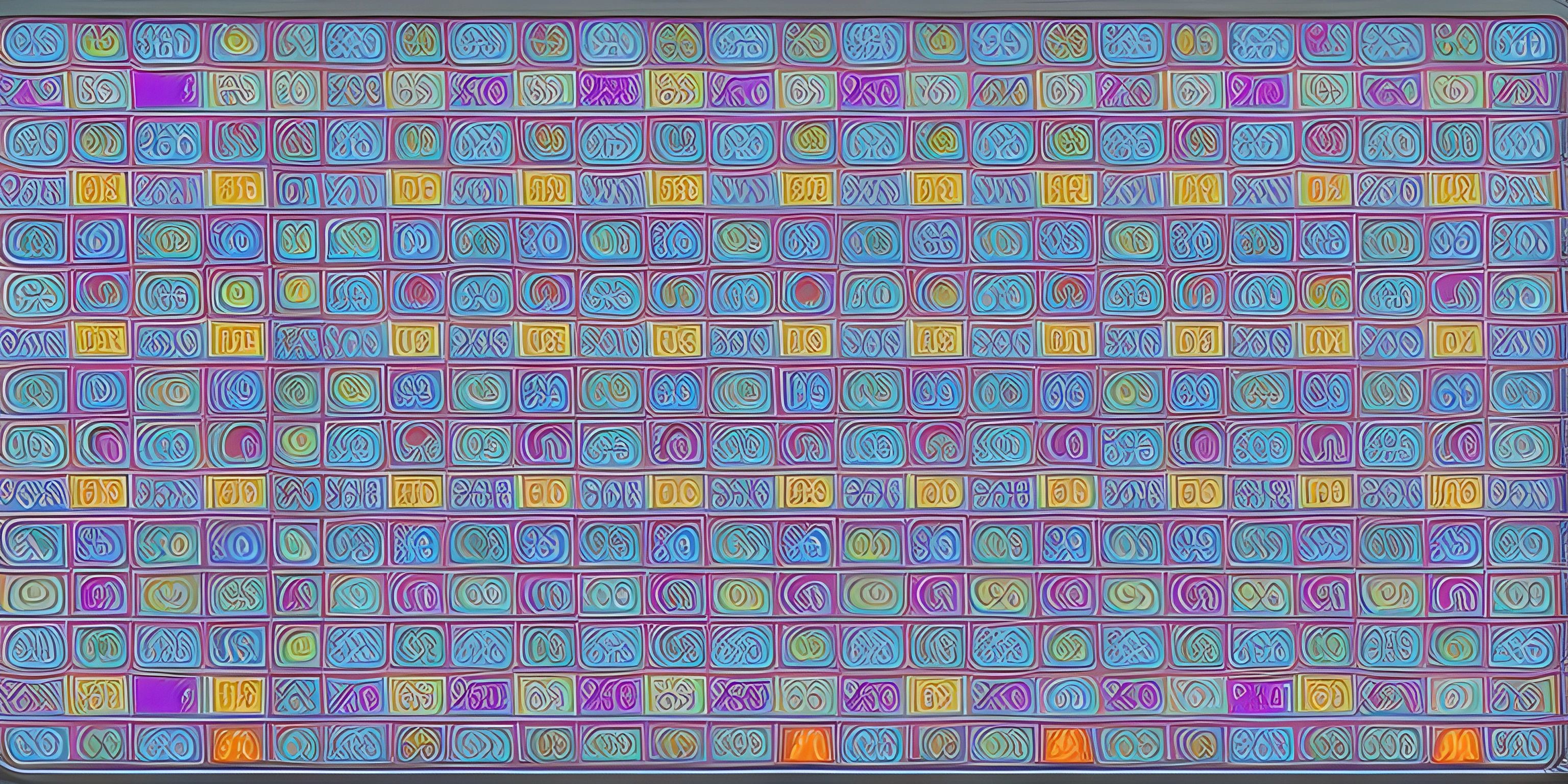
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Regular expressions, also known as regex or regexp, are a powerful tool for working with strings in JavaScript. They allow you to search, match, replace, and manipulate text based on specific patterns. In this article, we will cover the basics of regular expressions and some common use cases.
Creating Regular Expressions
There are two ways to create a regular expression in JavaScript:
- Using a regular expression literal: Enclose the pattern between forward slashes (
/
), like this:
const regex = /pattern/;
- Using the
RegExp
constructor: Pass the pattern as a string to theRegExp
constructor, like this:
const regex = new RegExp("pattern");
Regular Expression Flags
Flags are used to modify the behavior of regular expressions. You can add one or more flags to a regex by appending them after the closing forward slash or passing them as a second argument to the RegExp
constructor. Here are some common flags:
g
: Global search (find all matches, not just the first one)i
: Case-insensitive searchm
: Multiline mode (causes^
and$
to match the start and end of each line, not just the whole string)
Basic Regular Expression Methods
RegExp.test()
The test()
method checks if the regular expression matches any part of a given string. It returns true
if a match is found, and false
otherwise.
const regex = /hello/i; console.log(regex.test("Hello, world!")); // true
String.match()
The match()
method searches a string for matches of the regular expression. If the regex has the g
flag, it returns an array of all matches found. If the g
flag is not used, it returns an array with the first match and its capture groups.
const regex = /\d+/g; const str = "I have 3 apples and 5 oranges."; console.log(str.match(regex)); // ["3", "5"]
Common Use Cases
Matching Email Addresses
Here's a simple example of using a regular expression to match email addresses:
const emailRegex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/; console.log(emailRegex.test("[email protected]")); // true console.log(emailRegex.test("john.doe@example")); // false
Replacing Text
You can use the String.replace()
method to replace text in a string using a regular expression. Here's an example that replaces all occurrences of "apple" with "orange":
const regex = /apple/gi; const str = "I have an Apple and another apple."; console.log(str.replace(regex, "orange")); // "I have an orange and another orange."
These are just the basics of using regular expressions in JavaScript. Regex is a powerful tool, and learning more about its syntax and capabilities will help you handle more complex string manipulation tasks.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Enums (psst, it's free!).
FAQ
How can I create a regular expression in JavaScript?
There are two ways to create a regular expression in JavaScript. You can use the RegExp constructor, like this:
let regex = new RegExp("pattern", "flags");
Or you can use the literal syntax, like this:
let regex = /pattern/flags;
Replace "pattern" with your desired pattern and "flags" with any optional modifiers, such as "g" for global or "i" for case-insensitive.
How can I test if a string matches a regular expression?
You can use the .test()
method of a regular expression to check if a string matches the pattern. For example:
let regex = /hello/i; let text = "Hello, world!"; console.log(regex.test(text)); // Outputs: true
This checks if the string "Hello, world!" matches the case-insensitive pattern "hello".
How do I extract matches from a string using regular expressions?
You can use the .match()
method of a string to get an array of matches. For example:
let regex = /\d+/g; let text = "I have 3 apples and 5 bananas."; console.log(text.match(regex)); // Outputs: ["3", "5"]
This extracts all the numbers from the string and returns them in an array.
How can I replace parts of a string using regular expressions?
Use the .replace()
method of a string to replace parts that match a regular expression. For example:
let regex = /apples|bananas/g; let text = "I have 3 apples and 5 bananas."; console.log(text.replace(regex, "fruits")); // Outputs: "I have 3 fruits and 5 fruits."
This replaces all instances of "apples" and "bananas" with "fruits" in the given string.
How can I use capture groups in regular expressions?
Capture groups allow you to extract specific parts of a match. Use parentheses to create capture groups in your regular expression. For example:
let regex = /(\d+) (apples|bananas)/; let text = "I have 3 apples and 5 bananas."; let match = text.match(regex); console.log(match[1]); // Outputs: "3" console.log(match[2]); // Outputs: "apples"
This captures the number and the type of fruit in the first match.