Working with Strings and Arrays in JavaScript Functions
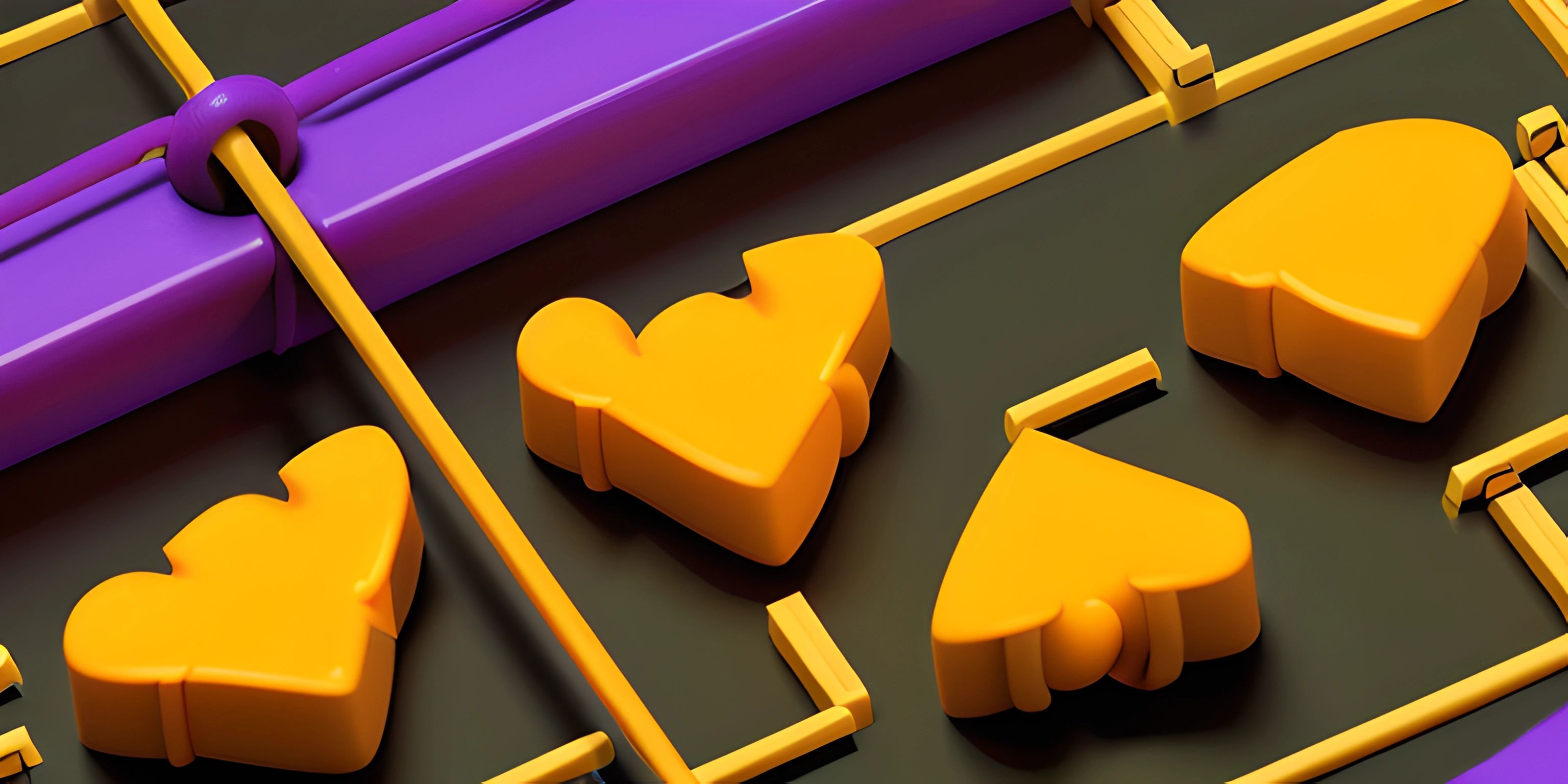
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JavaScript offers various methods and properties for working with strings and arrays. In this article, we will explore how to manipulate strings and arrays within JavaScript functions.
Strings in JavaScript Functions
Strings are sequences of characters, and JavaScript provides several built-in methods to manipulate and work with them. Let's look at some common string methods:
length
The length
property returns the number of characters in a string.
function getStringLength(str) { return str.length; } console.log(getStringLength("Hello, Cratecode!")); // Output: 16
concat()
The concat()
method is used to join two or more strings.
function joinStrings(str1, str2) { return str1.concat(str2); } console.log(joinStrings("Hello, ", "Cratecode!")); // Output: Hello, Cratecode!
slice()
The slice()
method extracts a part of a string and returns it as a new string.
function sliceString(str, start, end) { return str.slice(start, end); } console.log(sliceString("Hello, Cratecode!", 0, 5)); // Output: Hello
Arrays in JavaScript Functions
Arrays are used to store multiple values in a single variable. JavaScript provides several built-in methods to work with arrays. Let's look at some common array methods:
length
The length
property returns the number of elements in an array.
function getArrayLength(arr) { return arr.length; } console.log(getArrayLength(["apple", "banana", "cherry"])); // Output: 3
push()
The push()
method adds new items to the end of an array.
function addToArray(arr, item) { arr.push(item); return arr; } console.log(addToArray(["apple", "banana", "cherry"], "orange")); // Output: ["apple", "banana", "cherry", "orange"]
splice()
The splice()
method can be used to add, remove, or replace elements in an array.
function removeFromArray(arr, index) { arr.splice(index, 1); return arr; } console.log(removeFromArray(["apple", "banana", "cherry"], 1)); // Output: ["apple", "cherry"]
These are just a few examples of the methods and properties available for working with strings and arrays in JavaScript functions. For a more comprehensive list, consult the Mozilla Developer Network documentation for strings and arrays.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What are some common methods to manipulate strings in JavaScript functions?
Some common methods to manipulate strings in JavaScript functions are:
concat()
: combines two or more strings.indexOf()
: returns the index of the first occurrence of a specified value, or -1 if not found.slice()
: extracts a section of a string and returns it as a new string.split()
: separates a string into an array of substrings based on a specified separator.toLowerCase()
: converts the characters in a string to lowercase.toUpperCase()
: converts the characters in a string to uppercase.replace()
: searches for a specified value and replaces it with another value.
How can I manipulate arrays within JavaScript functions?
To manipulate arrays within JavaScript functions, you can use the following methods:
push()
: adds new elements to the end of an array.pop()
: removes the last element of an array.shift()
: removes the first element of an array.unshift()
: adds new elements to the beginning of an array.splice()
: adds/removes elements to/from an array, and returns the removed elements.slice()
: returns a shallow copy of a portion of an array.concat()
: merges two or more arrays.join()
: combines all elements of an array into a single string.
How can I find the length of a string or an array in JavaScript?
You can find the length of a string or an array by using the .length
property. For example:
let myString = "Hello, world!"; console.log(myString.length); // Outputs: 13 let myArray = [1, 2, 3, 4, 5]; console.log(myArray.length); // Outputs: 5
How can I iterate over the elements of a string or an array in JavaScript?
You can use a for
loop or the forEach()
method to iterate over the elements of a string or an array. For example:
let myString = "Hello"; for (let i = 0; i < myString.length; i++) { console.log(myString[i]); // Outputs: H, e, l, l, o } let myArray = [1, 2, 3]; myArray.forEach(function(element) { console.log(element); // Outputs: 1, 2, 3 });