JavaScript Overview
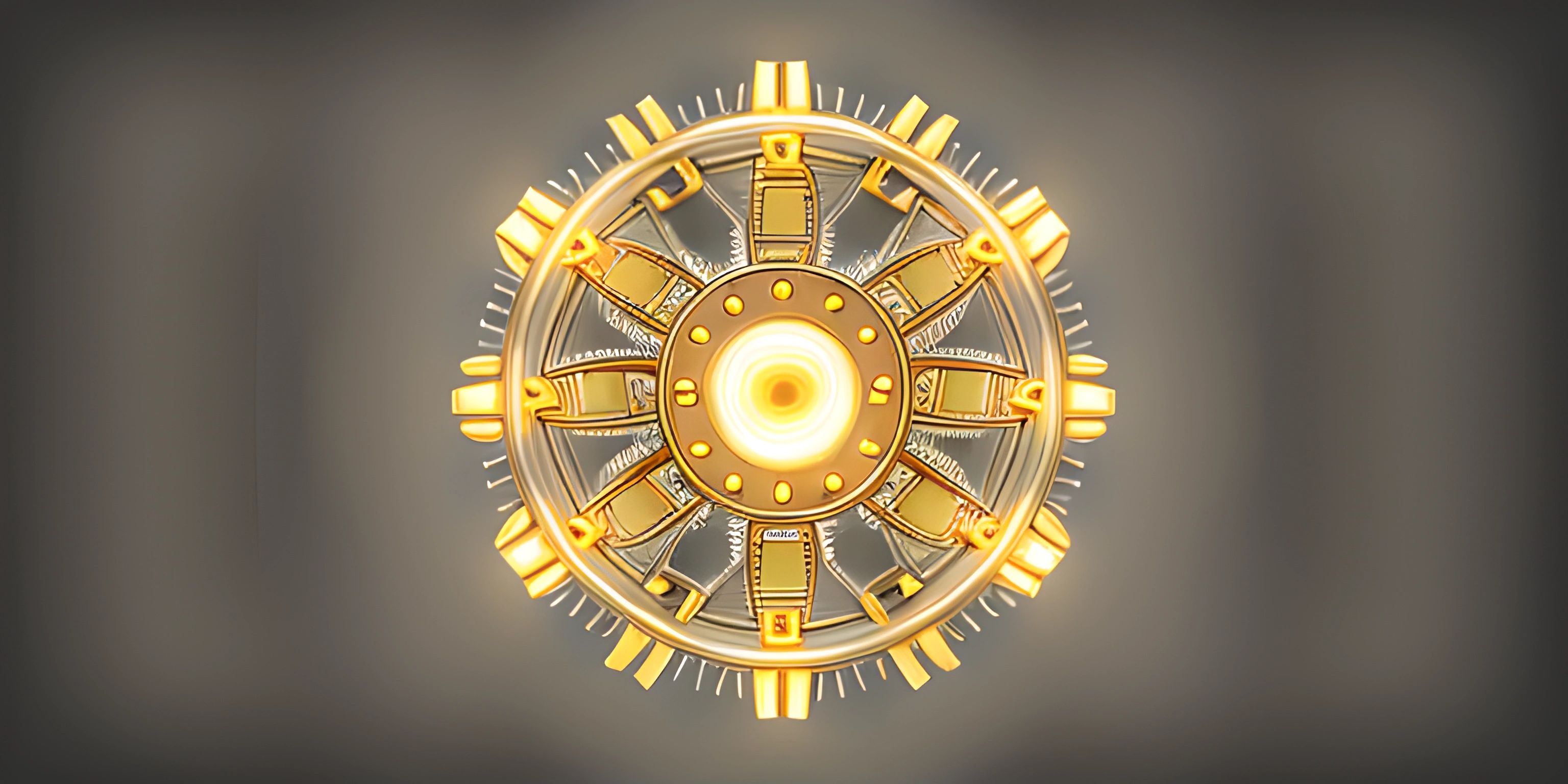
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the wonderful world of JavaScript! JavaScript is a versatile and widely-used programming language, playing a significant role in the world of web development. With its humble beginnings in 1995, this language has come a long way and now powers many of the interactive elements you see in modern websites.
A Brief History
JavaScript was created by Brendan Eich in just 10 days while he was working at Netscape. Originally named Mocha, it was later renamed to LiveScript and finally to JavaScript, mainly for marketing reasons. Despite its name, JavaScript is not related to Java, but rather has more in common with languages like C and Python.
Where is JavaScript Used?
JavaScript was initially designed to add interactivity to web pages, allowing developers to create engaging and dynamic user experiences. Over time, its capabilities have grown, and it is now used in a variety of contexts, such as:
-
Client-side scripting: JavaScript is popular for adding interactivity to web pages, including animations, form validation, and user-triggered events.
-
Server-side scripting: With the introduction of Node.js, JavaScript has expanded its reach to server-side scripting, allowing developers to build complete web applications using a single language.
-
Mobile app development: Libraries and frameworks like React Native and Ionic enable developers to build mobile apps using JavaScript.
-
Desktop app development: Tools such as Electron allow developers to create cross-platform desktop applications using web technologies like JavaScript, HTML, and CSS.
Basic Programming Concepts
JavaScript shares many fundamental programming concepts with other languages, such as:
Variables
Variables are used to store and manipulate data in a program. In JavaScript, you can declare variables using the let
and const
keywords.
let name = "Alice"; const age = 30;
Functions
Functions are blocks of code that can be defined and called by name. Functions can take arguments and return values.
function greet(name) { return `Hello, ${name}!`; } console.log(greet("Alice")); // Output: "Hello, Alice!"
Control Structures
Control structures, like if
statements and loops, allow you to execute different blocks of code based on conditions or iterate through data.
if (age >= 18) { console.log("You are an adult."); } else { console.log("You are a minor."); } for (let i = 1; i <= 5; i++) { console.log(i); }
Objects and Arrays
JavaScript is an object-oriented language, and objects are used to represent complex data structures. Arrays are a special type of object used to store lists of values.
let person = { name: "Alice", age: 30, }; let numbers = [1, 2, 3, 4, 5];
Now you have a basic understanding of JavaScript's history, uses, and core programming concepts. There's so much more to explore, so don't stop here! Dive into the world of JavaScript and start creating amazing web applications, mobile apps, and more. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is JavaScript, and how is it different from other programming languages?
JavaScript is a lightweight, interpreted, and versatile programming language that's primarily used for web development. It allows developers to add interactivity, animations, and other dynamic elements to websites. While other languages like Python and Ruby are multi-purpose, JavaScript is primarily focused on client-side scripting for web browsers, although it has evolved to be used in server-side programming with the help of platforms like Node.js.
How did JavaScript come to be, and what's its history?
JavaScript was created in 1995 by Brendan Eich, a Netscape Communications programmer. It was initially named Mocha, then LiveScript, and ultimately JavaScript to capitalize on the popularity of Java at the time. Despite the similarities in naming, JavaScript and Java are entirely separate programming languages. JavaScript has evolved over the years, with the ECMAScript standard shaping its development and ensuring compatibility across different web browsers.
What are some common uses for JavaScript in web development?
JavaScript plays a crucial role in modern web development, powering many dynamic aspects of websites. Common uses include:
- Animations and transitions
- Form validation and submission
- User interaction tracking
- Modifying HTML and CSS on the fly
- Creating responsive and interactive user interfaces
- Fetching and processing data from APIs
Can JavaScript be used for server-side programming?
Yes! Although JavaScript was initially designed for client-side scripting, the introduction of Node.js has enabled JavaScript to be used for server-side programming as well. Node.js is an open-source runtime environment that allows developers to write and run JavaScript code on the server side, making it possible to build full-stack applications with JavaScript.
What are some basic programming concepts in JavaScript?
JavaScript shares many fundamental programming concepts with other languages, such as:
- Variables and data types (strings, numbers, booleans, arrays, objects)
- Control structures (if/else, loops, switch)
- Functions and scope
- Events and event listeners
- Object-oriented programming and prototypes
- Error handling and exceptions