Understanding Arrays: An Introduction
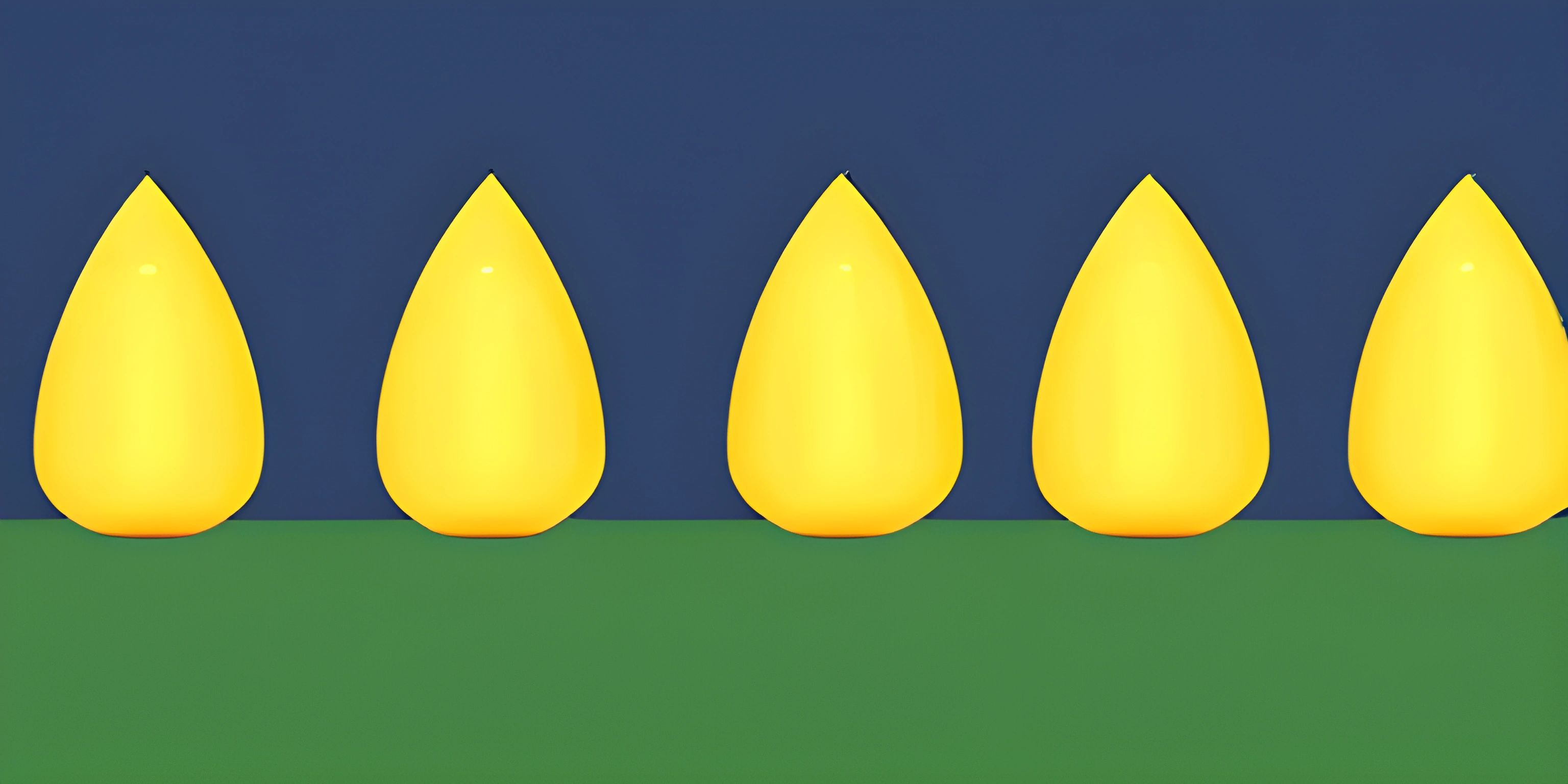
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Before we dive into the world of arrays, let's imagine a scenario. You are the proud owner of a grocery store and you have to keep track of various products. Now, you could have an individual name for each of your products, but that would be cumbersome, wouldn’t it? Instead, you group them into categories like fruits, vegetables, dairy, and so forth. These categories are a lot like arrays in programming.
What's an Array Anyway?
An array is a collection of similar types of data stored in contiguous memory locations. It's like a big family where Mom and Dad, the kids, Grandma, Grandpa, and even the dog and cat, live together under one roof. They all have different names, but they're part of the same family, so they live at the same address. That's what an array is - a group of elements of the same data type living under the same name.
Creating an Array
Creating an array is like planning a family reunion. You need to decide who to invite. In programming, we declare an array with a specific data type followed by array name and size. Here's an example in Javascript:
var familyMembers = ["Mom", "Dad", "Sis", "Bro", "Grandma", "Grandpa", "Dog", "Cat"];
In this case, familyMembers
is the array (or the family), and all the names inside the brackets are the elements (or the family members).
Accessing an Array
Remember how we mentioned that each family member has a unique name, but they all live under the same address? Well, in programming, we refer to this as the array index. It's like the family member's specific bedroom within the family home.
To access a specific array element, we use the array name followed by the index in square brackets. Here's how you can access "Sis" from our familyMembers
array:
var sister = familyMembers[2];
Wait a minute! Why 2? Shouldn't it be 3 since "Sis" is the third element? Well, in most programming languages, arrays are zero-indexed, which means they start counting from 0 instead of 1. So "Mom" is at index 0, "Dad" is at index 1, and "Sis" is at index 2. This is one family tradition that might seem weird at first, but you'll get used to it!
Arrays are one of the most widely used data structures in programming, and understanding them is vital to becoming a proficient programmer. So, welcome to the family!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Word Splitter (psst, it's free!).
FAQ
What is an array in programming?
An array in programming is a collection of similar types of data stored in contiguous memory locations. It's like a group of elements of the same data type living under the same name.
How do you create an array?
In most programming languages, you create an array by declaring it with a specific data type, the array name, and size. For example, in Javascript, you could create an array like this: var familyMembers = ["Mom", "Dad", "Sis", "Bro", "Grandma", "Grandpa", "Dog", "Cat"];
.
How do you access elements within an array?
To access a specific array element, you use the array name followed by the index number in square brackets. For example, to access "Sis" from the familyMembers
array, you would write: var sister = familyMembers[2];
.
What does zero-indexed mean?
Zero-indexed means that counting starts from 0 instead of 1. So in an array, the first element is at index 0, the second element is at index 1, and so on.
Why are arrays important in programming?
Arrays are important in programming because they allow programmers to store multiple values in a single variable, instead of declaring separate variables for each value. They also provide a way to group related data together, which can simplify code and make it easier to understand and maintain.