Working with Events and Event Listeners in JavaScript
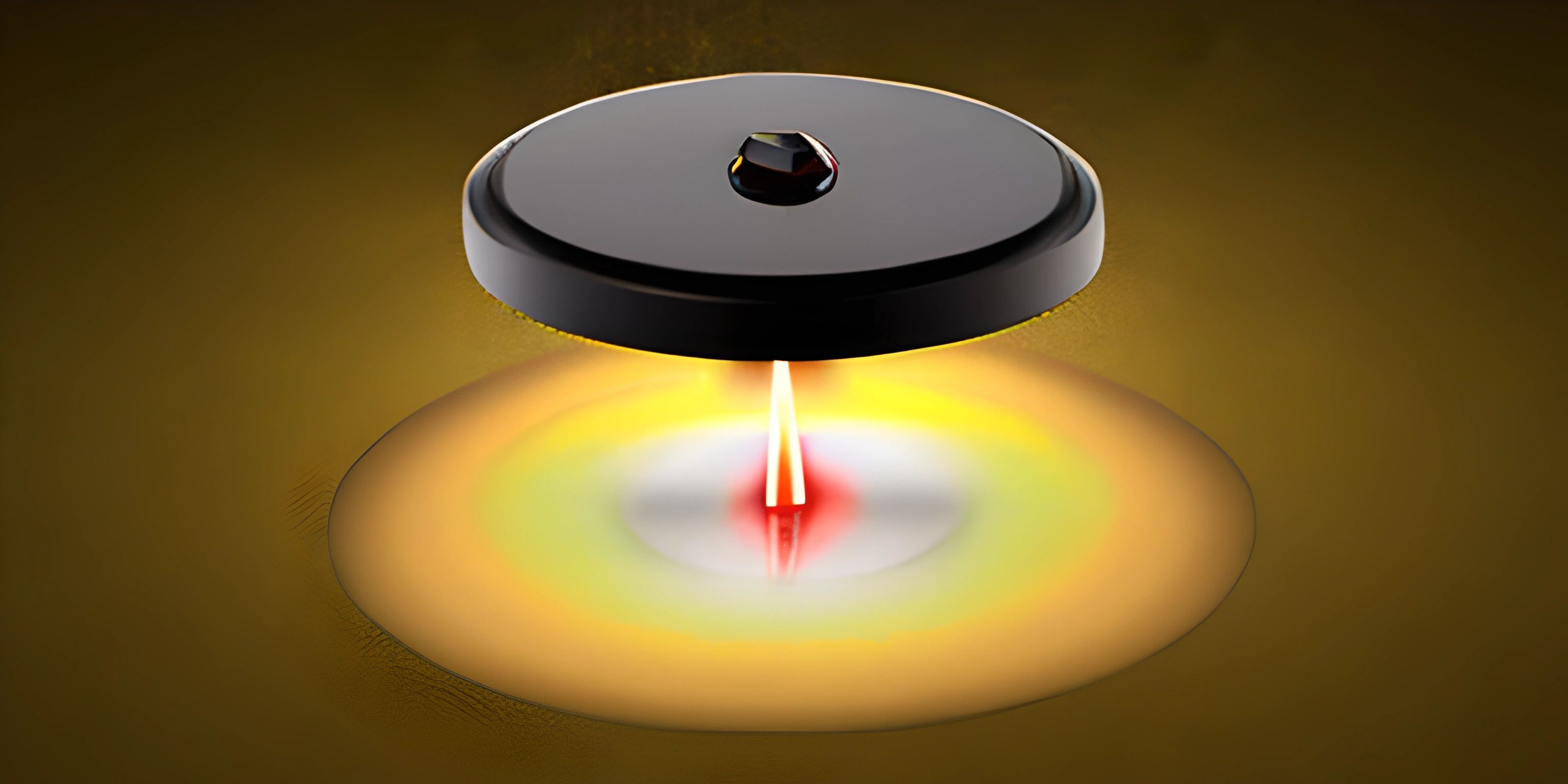
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JavaScript events are actions or occurrences, such as a user clicking a button or a page finishing loading, that can be detected and acted upon by your code. Event listeners are functions that can be attached to HTML elements to "listen" for specific events and execute code in response.
Adding Event Listeners
To add an event listener to an HTML element, you can use the addEventListener
method. The method takes two arguments: the type of event you want to listen for, and the function to be executed when the event occurs.
Here's an example of adding a click event listener to a button:
// Get the button element by its ID var button = document.getElementById("myButton"); // Define the function to be executed when the button is clicked function handleClick() { alert("Button clicked!"); } // Add the click event listener to the button button.addEventListener("click", handleClick);
Removing Event Listeners
To remove an event listener, you can use the removeEventListener
method. It takes the same arguments as addEventListener
: the event type and the function to be removed.
// Remove the click event listener from the button button.removeEventListener("click", handleClick);
Note: To remove an event listener, the function passed to removeEventListener
must be the same as the one passed to addEventListener
. Anonymous functions cannot be removed.
Event Object
When an event is triggered, an event object is created and passed to the event listener function. This object contains information about the event, such as the target element, the type of event, and any additional data associated with the event.
Here's an example of using the event object to display the target element's ID:
function handleClick(event) { alert("Button clicked: " + event.target.id); } button.addEventListener("click", handleClick);
Event Bubbling and Capturing
Event bubbling and capturing are two ways in which event propagation can occur in the DOM. By default, most events bubble, meaning that they propagate from the target element up through its ancestors until they reach the root element.
Event capturing is the opposite: events propagate from the root element down to the target element.
You can control the propagation mode by passing a third argument to addEventListener
. If true
, the event will be captured; if false
or omitted, the event will bubble.
// Capture the click event button.addEventListener("click", handleClick, true);
Preventing Default Behavior
Some events have a default behavior associated with them, such as following a link when it's clicked. You can prevent this default behavior by calling the preventDefault
method on the event object.
function handleLinkClick(event) { event.preventDefault(); alert("Link clicked, but not followed."); } var link = document.getElementById("myLink"); link.addEventListener("click", handleLinkClick);
By understanding and using events and event listeners in JavaScript, you can create more interactive and dynamic web applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Websites (psst, it's free!).
FAQ
What is an event in JavaScript?
An event in JavaScript is an action or occurrence detected by the browser, such as a click, a keypress, or a page load. Events allow you to make your web applications interactive by responding to user actions or changes in the environment.
How do I create an event listener in JavaScript?
To create an event listener in JavaScript, use the addEventListener()
method. This method takes two arguments: the event type (e.g., "click" or "keypress") and a function to be executed when the event occurs. Here's an example:
const button = document.querySelector("button"); button.addEventListener("click", function() { alert("Button clicked!"); });
Can I remove an event listener after it's been added?
Yes, you can remove an event listener using the removeEventListener()
method. It requires the same arguments as addEventListener()
: the event type and the function to be removed. Keep in mind that you must pass the original function, not an anonymous one. For instance:
const button = document.querySelector("button"); function handleClick() { alert("Button clicked!"); } button.addEventListener("click", handleClick); // Later in your code, when you want to remove the event listener: button.removeEventListener("click", handleClick);
How do I access event-related information inside an event listener function?
When an event is triggered, an event object is automatically passed to the listener function. This object contains various properties and methods related to the event, such as its type and target. To access this information inside the listener function, you can use the parameter, typically named event
or e
:
const button = document.querySelector("button"); button.addEventListener("click", function(event) { console.log("Event type:", event.type); // "click" console.log("Event target:", event.target); // button element });
Can I use event delegation to handle events on multiple elements?
Yes, event delegation is a technique that allows you to handle events on multiple elements with a single event listener. By attaching the listener to a parent element, you can take advantage of event bubbling to capture events originating from its descendants. Here's an example:
const list = document.querySelector("ul"); list.addEventListener("click", function(event) { if (event.target.tagName === "LI") { alert("List item clicked: " + event.target.textContent); } });