LLDB Introduction
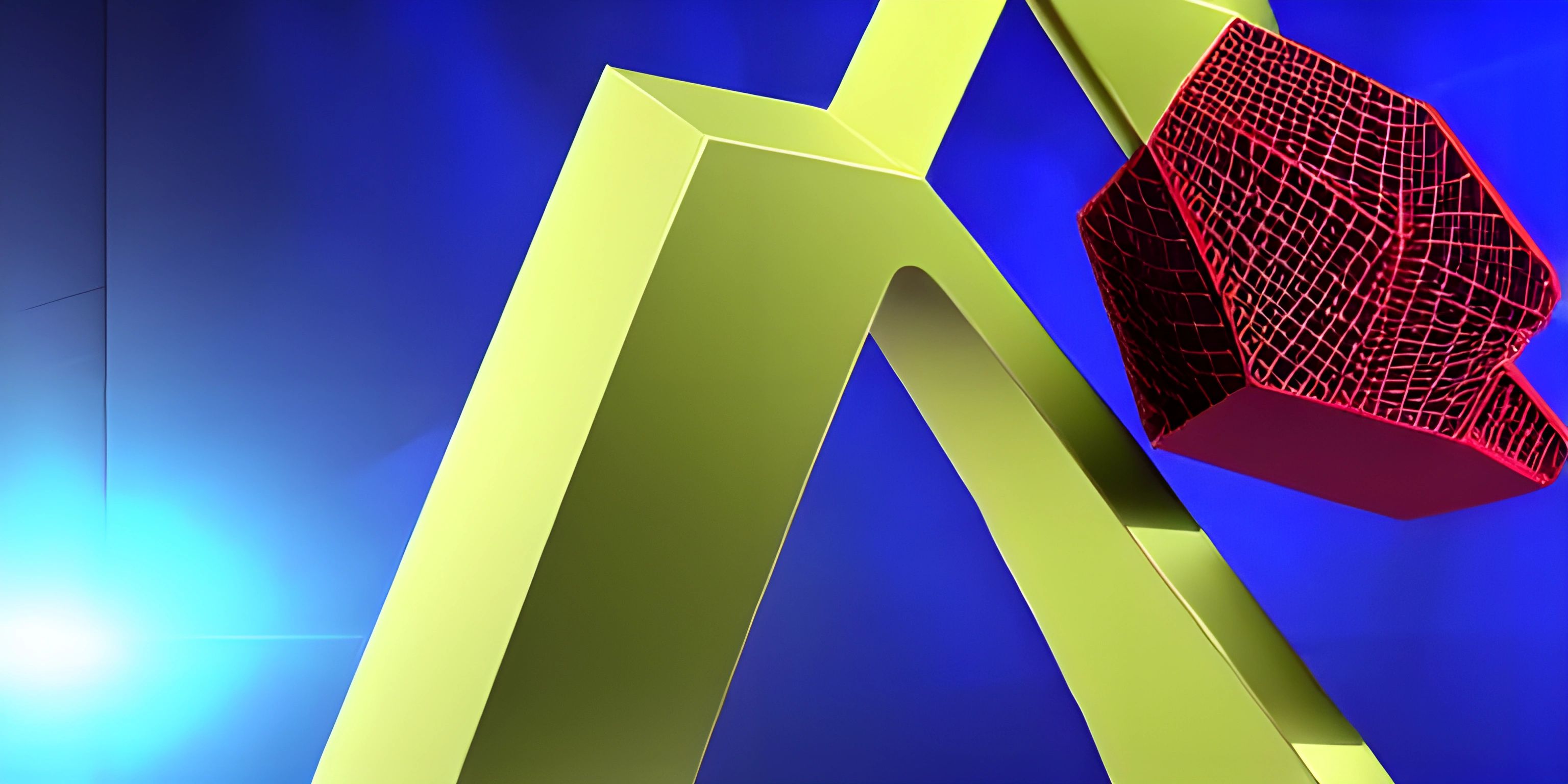
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Debugging is an essential part of the software development process. When your program behaves unexpectedly or crashes, you'll need a powerful tool to help you figure out what went wrong. Enter LLDB - the LLVM Debugger. It's designed to work with languages like C, C++, and others that use LLVM as their backend. In this guide, you'll learn about LLDB and how it can help you diagnose issues in your code.
What is LLDB?
LLDB is a command-line debugger that's part of the LLVM project. It's built on top of LLVM libraries, which allows it to quickly and efficiently debug programs written in C, C++, and other languages that utilize LLVM. It has many powerful features, such as:
- Support for multiple languages, including C, C++, Objective-C, Swift, Ada, and others
- Breakpoints, watchpoints, and expression evaluation
- Extensive support for examining and modifying program state
- Scripting capabilities using Python
- Cross-platform support, including macOS, Linux, and Windows
Getting Started with LLDB
To get started with LLDB, first, you need to install it. It's typically included with Clang and the LLVM toolchain, but you can also find standalone packages for macOS, Linux, and Windows.
Once installed, you can run the lldb
command followed by the name of the executable you want to debug:
$ lldb my_program
This will launch LLDB, and you'll see its command prompt. From here, you can issue various commands to control the execution of your program, examine its state, and modify it as needed.
Common LLDB Commands
Here are some common LLDB commands that you'll find useful during your debugging sessions:
run
: Starts the execution of your programbreakpoint set
: Sets a breakpoint at a specific location (e.g., at a function or line of source code)step
: Executes the current line of code and moves to the next one, stepping into functions if necessarynext
: Executes the current line of code and moves to the next one, stepping over functionsframe variable
: Displays the values of variables in the current stack frameexpression
: Evaluates an expression in the context of the current program state
For a full list of commands, you can consult the official LLDB documentation.
Advanced Features
In addition to basic debugging commands, LLDB offers advanced features that can help you gain deeper insights into your program's behavior:
- Reverse Debugging: LLDB supports reverse debugging, which allows you to step backward through your program's execution. This can be useful when you want to examine the events that led up to a particular issue.
- Memory Analysis: With LLDB, you can examine your program's memory, search for specific values, and even modify memory directly.
- Python Scripting: LLDB provides a Python API, allowing you to write custom scripts to automate debugging tasks or extend the debugger with new functionality.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is LLDB?
LLDB is a command-line debugger that's part of the LLVM project. It's designed to work with languages like C, C++, and others that use LLVM as their backend. It has many powerful features, such as support for multiple languages, breakpoints, watchpoints, expression evaluation, scripting capabilities using Python, and cross-platform support.
How do you start LLDB for a specific program?
To start LLDB for a specific program, you can run the lldb
command followed by the name of the executable you want to debug. For example: $ lldb my_program
. This will launch LLDB and its command prompt, where you can issue various commands to control the execution of your program, examine its state, and modify it as needed.
What are some common LLDB commands?
Some common LLDB commands include run
, breakpoint set
, step
, next
, frame variable
, and expression
. These commands allow you to control the execution of your program, set breakpoints, step through code, examine variable values, and evaluate expressions in the context of the current program state.