GDB Introduction
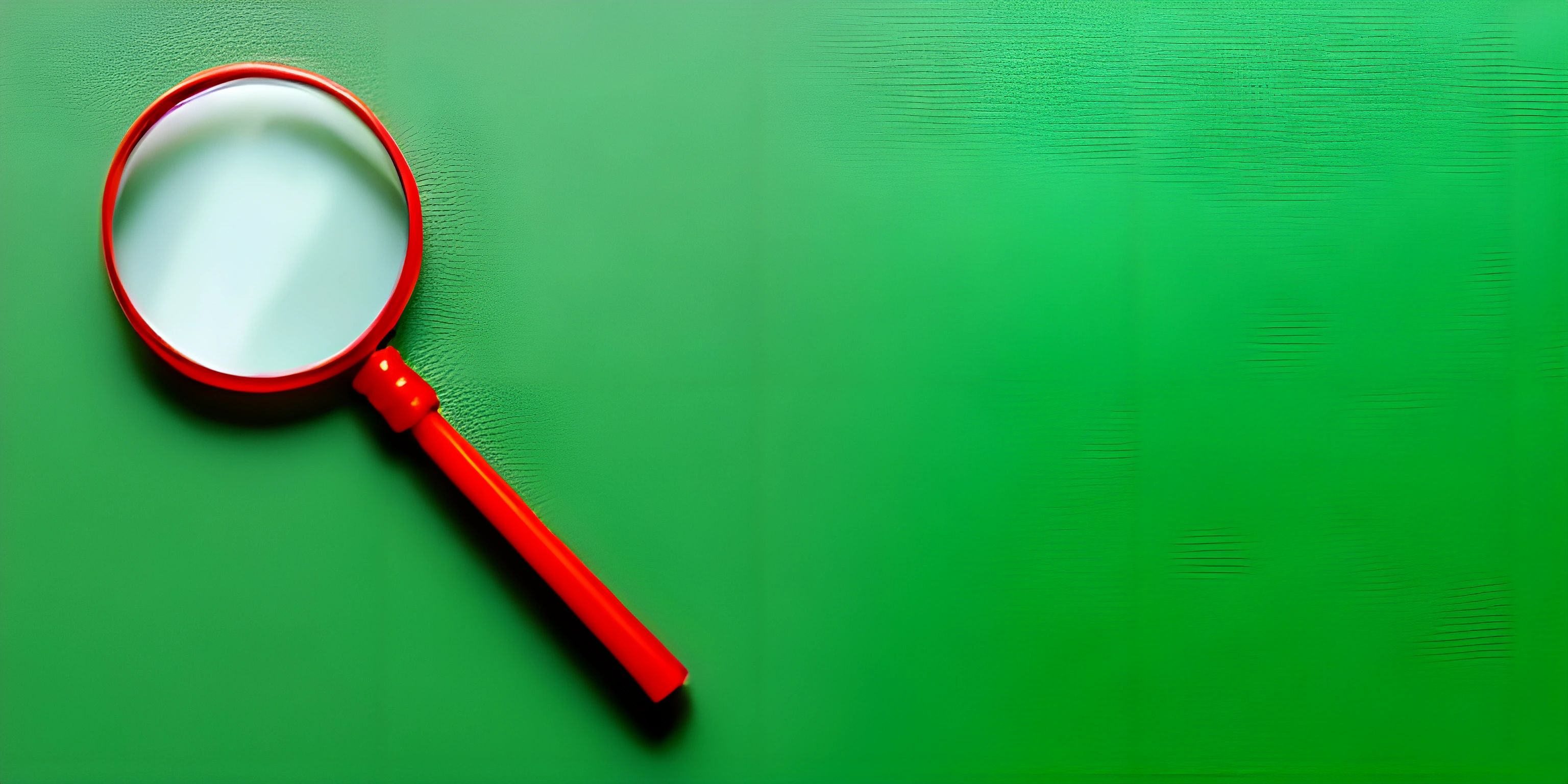
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you're a C or C++ developer, you're bound to face the inevitable: bugs! And when you find one, you can either use printf
statements to debug, or you can turn to the powerful GNU Debugger, GDB. In this article, we'll explore GDB and see how it can make your life easier when debugging your code.
What is GDB?
GDB, or the GNU Debugger, is a debugging tool for C and C++ programs. It allows you to set breakpoints, step through your code, and inspect variables and memory to figure out what's going on under the hood. GDB is an open-source debugger that's been around for decades and has become a staple in the C and C++ development world.
Getting Started with GDB
To get started, you'll need to install GDB – it's likely available through your package manager. For example, on Ubuntu or Debian-based systems, you can use the following command to install GDB:
sudo apt-get install gdb
Once you have GDB installed, you'll need to compile your C or C++ program with debugging symbols. This is done by adding the -g
flag to your gcc
or g++
command, like this:
gcc -g my_program.c -o my_program
Now, you're ready to start debugging your program using GDB. To do this, simply run the following command, replacing my_program
with your compiled binary:
gdb my_program
GDB Commands
GDB has a vast array of commands to help you navigate and debug your code. Here are some essential commands to get you started:
run
: Starts the execution of your program.break
: Sets a breakpoint at a specific line or function. For example,break main
orbreak 42
.continue
: Resumes the execution of your program after a breakpoint.step
: Executes the current line of code and stops at the next line or function call.next
: Similar tostep
, but it doesn't enter function calls.print
: Displays the value of a variable or expression. For example,print my_var
orprint 2 + 3
.backtrace
: Shows the call stack, which is a list of functions that have been called to reach the current point in the code.
For a more comprehensive list of GDB commands, you can refer to the official GDB documentation.
Example: Debugging a Simple C Program
Let's look at a simple example to see how GDB can help you find bugs in your C or C++ code. Suppose you have the following C program, example.c
:
#include <stdio.h> int add(int x, int y) { return x + y; } int main() { int a = 5; int b = 10; int sum = add(a, b); printf("The sum is: %d\n", sum); return 0; }
Compile the program with debugging symbols:
gcc -g example.c -o example
Now, let's use GDB to debug example
:
gdb example
As you can see, GDB allows you to step through your code, inspect variables, and find issues with ease. With a solid understanding of GDB and its commands, you'll be well-equipped to squash bugs and improve your C and C++ code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Mandelbrot Set (psst, it's free!).
FAQ
Why should I use GDB instead of `printf` debugging?
GDB provides a powerful, interactive environment for debugging C and C++ programs. It allows you to set breakpoints, step through your code, and inspect variables and memory at runtime. While printf
debugging can be helpful in some cases, GDB offers a more efficient and comprehensive debugging solution.
How do I compile my C or C++ program with debugging symbols?
To compile your C or C++ program with debugging symbols, add the -g
flag to your gcc
or g++
command, like this: gcc -g my_program.c -o my_program
. The debugging symbols will allow GDB to display source code and variable information when debugging your program.
What is the difference between the `step` and `next` commands in GDB?
The step
command in GDB executes the current line of code and stops at the next line or function call, allowing you to step into function calls. The next
command, on the other hand, executes the current line of code and stops at the next line, but it doesn't enter function calls. This allows you to step over function calls when you're not interested in debugging their internals.