MATLAB Basics
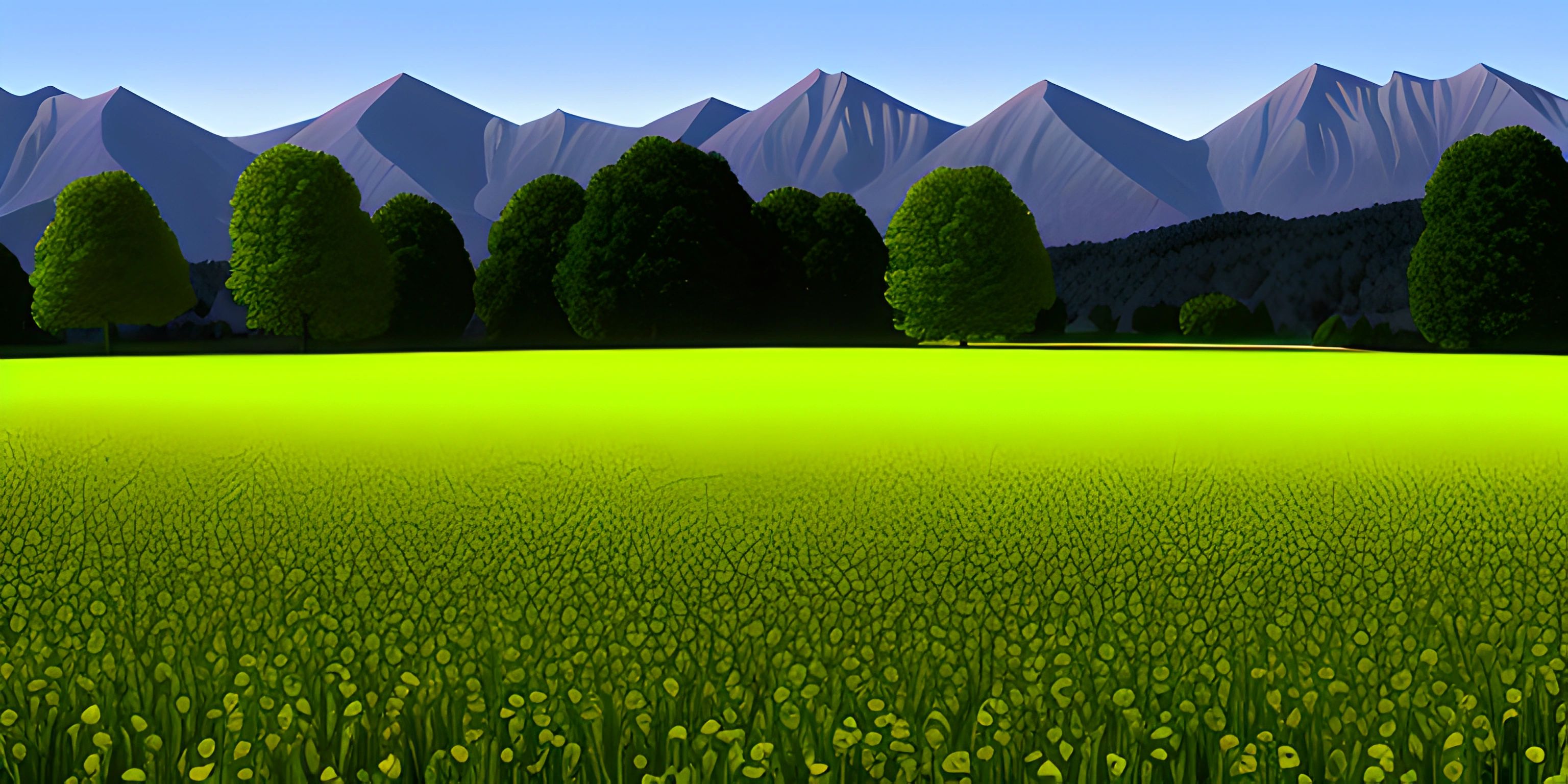
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MATLAB, short for MATrix LABoratory, is a powerful numerical computing environment that's widely used in various fields such as engineering, physics, and finance. In MATLAB, everything is treated as a matrix, which makes it a perfect tool for solving complex mathematical problems. So, let's dive into the world of MATLAB and explore some of its fundamental concepts.
Matrices and Vectors
The heart of MATLAB is all about matrices and vectors. A matrix is a 2-dimensional grid of numbers, while a vector is simply a 1-dimensional matrix (a row or a column). Creating matrices and vectors in MATLAB is a breeze:
% A simple row vector row_vector = [1, 2, 3, 4]; % A simple column vector column_vector = [1; 2; 3; 4]; % A 2x2 matrix A = [1, 2; 3, 4];
Matrix Manipulation
MATLAB offers a wide range of operations and functions to manipulate matrices. Here are some examples:
% Transpose a matrix B = A'; % Multiply matrices C = A * B; % Invert a matrix D = inv(A); % Calculate the determinant det_A = det(A);
Functions
Like any other programming language, MATLAB allows you to define your own functions. Functions help you reuse code, modularize your programs, and make them easier to manage. Here's an example function that takes a matrix and a scalar value, and multiplies each element of the matrix by the scalar:
function result = scale_matrix(matrix, scalar) result = matrix * scalar; end
You can then use this function in your code like this:
A = [1, 2; 3, 4]; scaled_A = scale_matrix(A, 2);
Plotting
MATLAB is widely known for its powerful plotting capabilities. With just a few lines of code, you can create stunning visualizations to help you analyze data or present your results. Here's a simple example to plot a sine wave:
% Create an array of linearly spaced points between 0 and 2*pi x = linspace(0, 2 * pi, 100); % Calculate the sine of x y = sin(x); % Create a plot plot(x, y); % Label the axes xlabel('x'); ylabel('sin(x)'); % Add a title title('A Sine Wave');
These are just the basics of what you can accomplish with MATLAB. As you explore further, you'll discover a wide range of powerful tools and techniques to help you tackle even the most complex mathematical challenges.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Mandelbrot Set (psst, it's free!).
FAQ
What is MATLAB?
MATLAB, short for MATrix LABoratory, is a numerical computing environment widely used in various fields such as engineering, physics, and finance. It treats everything as a matrix, making it an ideal tool for solving complex mathematical problems.
What are matrices and vectors in MATLAB?
In MATLAB, a matrix is a 2-dimensional grid of numbers, while a vector is a 1-dimensional matrix, either a row or a column. They form the core of MATLAB's functionality, and many operations and functions revolve around manipulating them.
How do you create a function in MATLAB?
In MATLAB, you can create a function using the "function" keyword, followed by the output variable, the function name, and the input parameters in parentheses. The function body is then enclosed in the "end" keyword. For example:
function result = scale_matrix(matrix, scalar) result = matrix * scalar; end
How do you create a basic plot in MATLAB?
To create a basic plot in MATLAB, you can use the "plot" function, passing in the data points for the x and y axes. You can also add labels, titles, and other elements to the plot using functions like "xlabel", "ylabel", and "title". For example:
x = linspace(0, 2 * pi, 100); y = sin(x); plot(x, y); xlabel('x'); ylabel('sin(x)'); title('A Sine Wave');