Functions in MATLAB
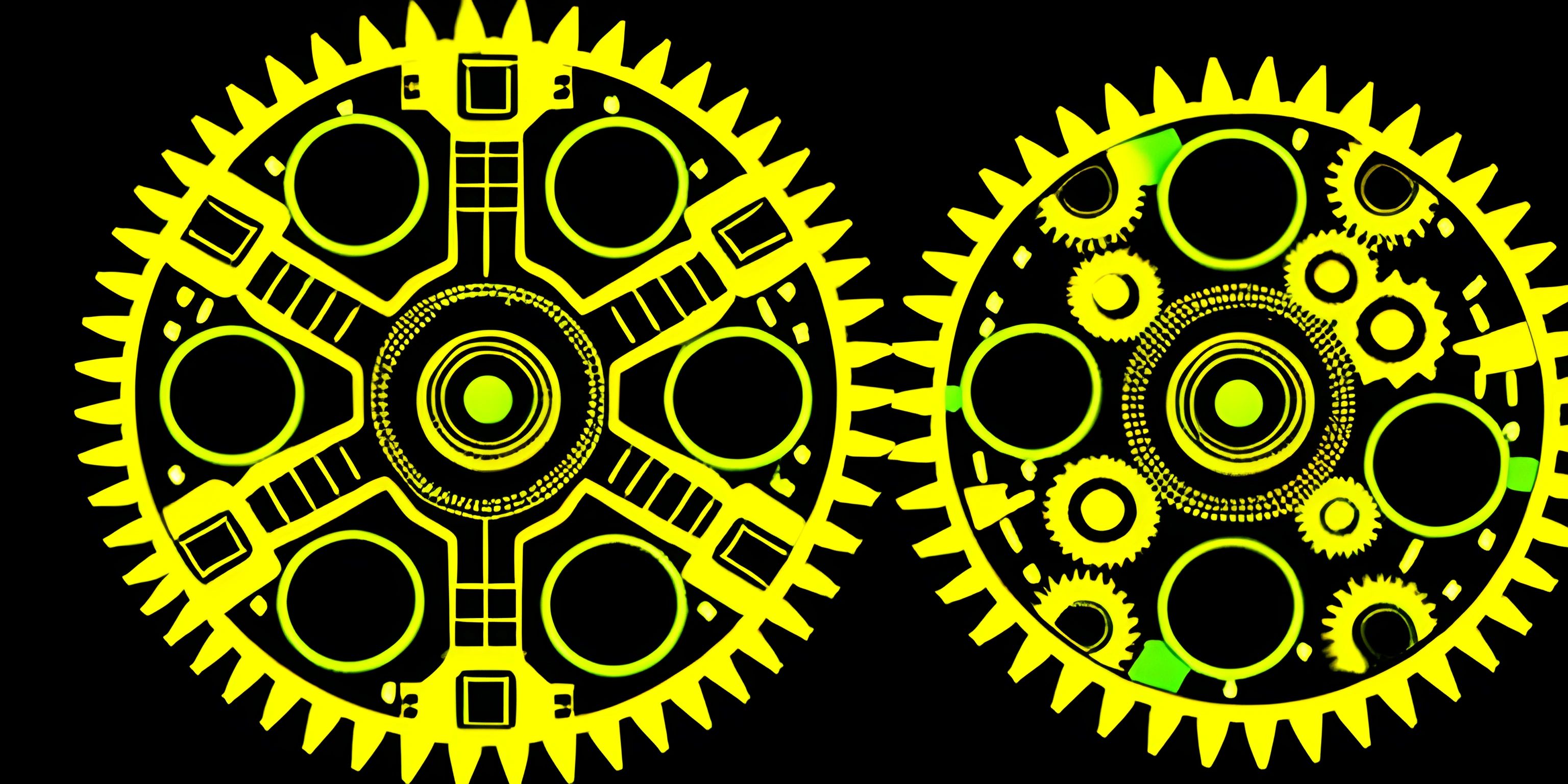
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MATLAB is a powerful programming language and environment widely used for numerical and symbolic computing. One of its key features is the ability to create and use functions. Functions in MATLAB are essential as they help break down complex problems into smaller, manageable tasks and promote code reusability.
Defining Functions in MATLAB
In MATLAB, functions are defined in separate .m
files, where the file name must match the function's name. To create a simple function, start by using the function
keyword, followed by the output variables (in square brackets, if more than one), the function name, and input variables in parentheses.
Here is the basic syntax for defining a function in MATLAB:
function [output1, output2] = functionName(input1, input2) % Function body % ... end
Let's create a simple function called sumAndDifference
that takes two numbers as input and returns their sum and difference:
function [sum, difference] = sumAndDifference(a, b) sum = a + b; difference = a - b; end
Save this code in a file named sumAndDifference.m
.
Calling Functions in MATLAB
To call a function, simply use its name followed by input arguments in parentheses. Here's an example of calling the sumAndDifference
function:
[s, d] = sumAndDifference(4, 2); disp(['Sum: ', num2str(s), ', Difference: ', num2str(d)]);
This will output:
Sum: 6, Difference: 2
Local Variables and Scope
Variables defined inside a function have local scope, meaning they are only accessible within the function. This helps avoid naming conflicts and keeps variables organized.
Consider the following example:
function [result] = myFunction(x) a = 5; result = a * x; end
In this function, the variable a
is local to myFunction
and cannot be accessed outside of it.
Anonymous Functions
MATLAB also supports anonymous functions, which are short, single-expression functions that don't require a separate .m
file. They are defined using the @
symbol, followed by input arguments, and an expression.
Here's an example of an anonymous function that squares its input:
square = @(x) x^2; result = square(4); disp(['Square: ', num2str(result)]);
This will output:
Square: 16
Anonymous functions are useful when you need a simple, quick function without creating a separate file.
FAQ
How are functions defined in MATLAB?
Functions in MATLAB are defined using the function
keyword, followed by output variables, the function name, and input variables. They are stored in separate .m
files with the file name matching the function's name.
How do I call a function in MATLAB?
To call a function in MATLAB, simply use its name followed by input arguments in parentheses, like result = functionName(input1, input2)
.
What is the scope of variables inside a function?
Variables defined inside a function have local scope, meaning they can only be accessed within that function. This helps avoid naming conflicts and keeps variables organized.
What are anonymous functions in MATLAB?
Anonymous functions in MATLAB are short, single-expression functions that don't require a separate .m
file. They are defined using the @
symbol, followed by input arguments and an expression. They are useful for creating simple, quick functions without a separate file.