Exploring Basic MATLAB Syntax and Commands
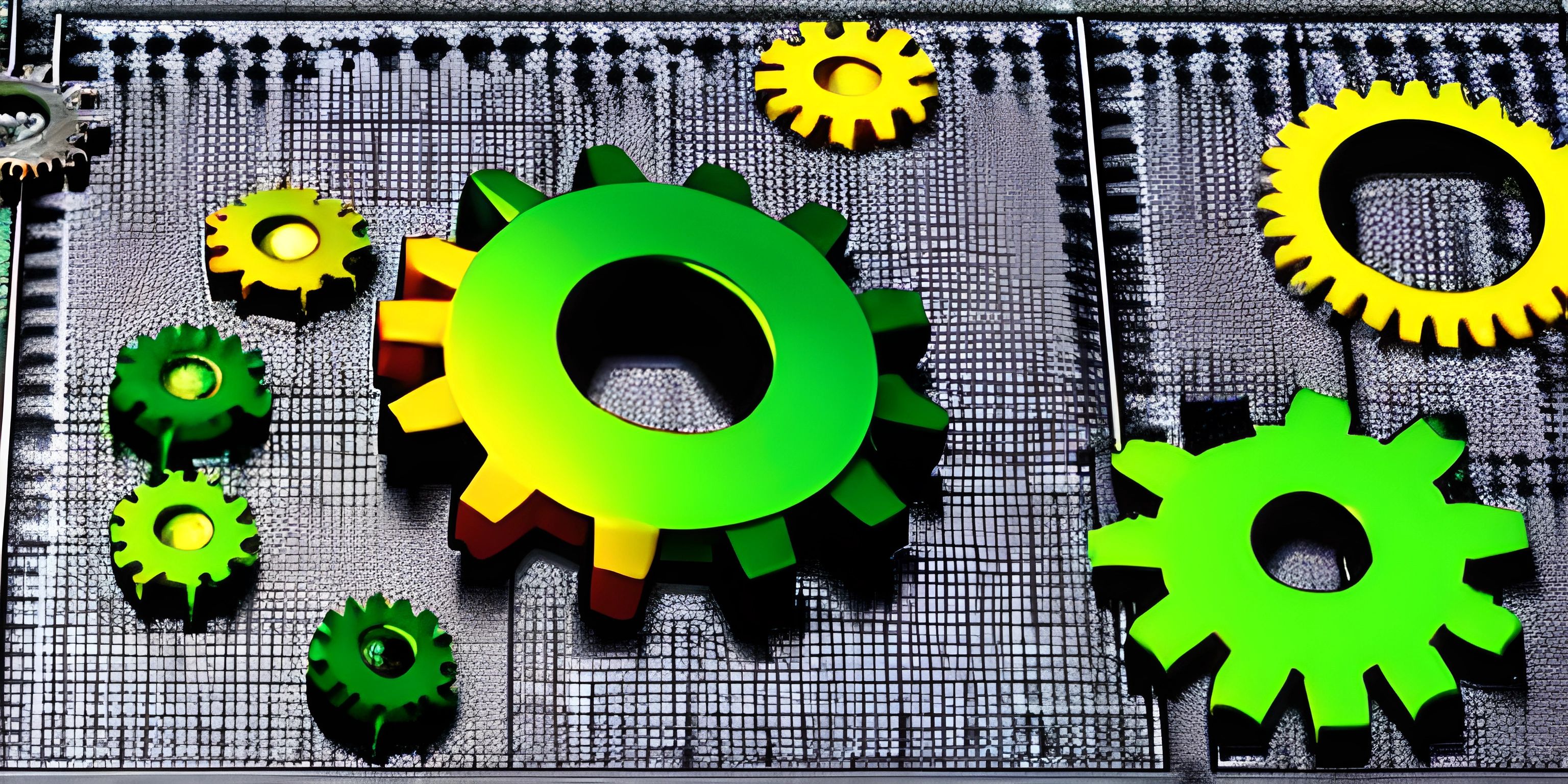
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MATLAB, short for Matrix Laboratory, is a powerful and versatile programming language often used in engineering, mathematics, and scientific applications. Its syntax and commands may seem unusual at first, but don't worry – we'll walk you through the basics so you can start working with MATLAB in no time.
The Basics
MATLAB is built around working with matrices and vectors, and its syntax reflects that. Let's start by looking at some fundamental MATLAB commands.
Creating Matrices and Vectors
To create a matrix or a vector in MATLAB, you can use square brackets [ ]
and separate the elements with spaces or commas. Rows are separated by semicolons ;
:
% Create a row vector row_vector = [1, 2, 3] % Create a column vector column_vector = [1; 2; 3] % Create a 3x3 matrix matrix = [1, 2, 3; 4, 5, 6; 7, 8, 9]
Basic Operations
MATLAB supports various basic operations on matrices and vectors, including addition, subtraction, and multiplication. For example:
A = [1, 2; 3, 4] B = [4, 3; 2, 1] % Addition C = A + B % Subtraction D = A - B % Matrix multiplication E = A * B
Functions and Commands
MATLAB comes with a vast library of built-in functions. Here are some essential ones to get you started:
length
The length
function returns the length of the longest dimension of a matrix or vector:
v = [1, 2, 3, 4, 5] len_v = length(v) % Returns 5
size
The size
function returns the dimensions of a matrix:
M = [1, 2, 3; 4, 5, 6] size_M = size(M) % Returns [2, 3]
zeros and ones
The zeros
and ones
functions create matrices of specified dimensions, filled with zeros or ones, respectively:
zero_matrix = zeros(2, 3) one_matrix = ones(3, 3)
linspace
The linspace
function generates linearly spaced vectors:
% Create a vector with 10 values from 0 to 1 lin_space = linspace(0, 1, 10)
Plotting
MATLAB is particularly popular for its powerful plotting capabilities. Let's look at some basic plotting commands:
plot
The plot
function creates a 2D line plot of a given dataset:
x = linspace(0, 2*pi, 100) y = sin(x) plot(x, y)
xlabel, ylabel, and title
These functions add labels and a title to your plot:
xlabel('Time') ylabel('Amplitude') title('Sine Wave')
legend
The legend
function adds a legend to your plot, which is useful when displaying multiple datasets:
x = linspace(0, 2*pi, 100) y1 = sin(x) y2 = cos(x) plot(x, y1, x, y2) legend('sin(x)', 'cos(x)')
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is MATLAB?
MATLAB is a high-level programming language and environment primarily designed for numerical computing. It is widely used in engineering, mathematics, and scientific applications. MATLAB stands for Matrix Laboratory, as it is built around matrices and vectors.
How do you create a matrix or a vector in MATLAB?
In MATLAB, you can create a matrix or a vector using square brackets [ ]
. Elements within a row are separated by spaces or commas, while rows are separated by semicolons ;
. For example, row_vector = [1, 2, 3]
creates a row vector, and matrix = [1, 2, 3; 4, 5, 6; 7, 8, 9]
creates a 3x3 matrix.
How do you perform basic operations on matrices and vectors in MATLAB?
MATLAB supports various basic operations on matrices and vectors, such as addition, subtraction, and multiplication. You can perform these operations using the standard arithmetic operators +
, -
, and *
. For example, C = A + B
adds two matrices A and B, and E = A * B
multiplies them.
How do you create a basic 2D plot in MATLAB?
To create a basic 2D plot in MATLAB, you can use the plot
function. This function takes two equal-length vectors as input, representing the x and y coordinates of the data points. For example, plot(x, y)
creates a line plot of the dataset defined by the x and y vectors.