MATLAB Functions
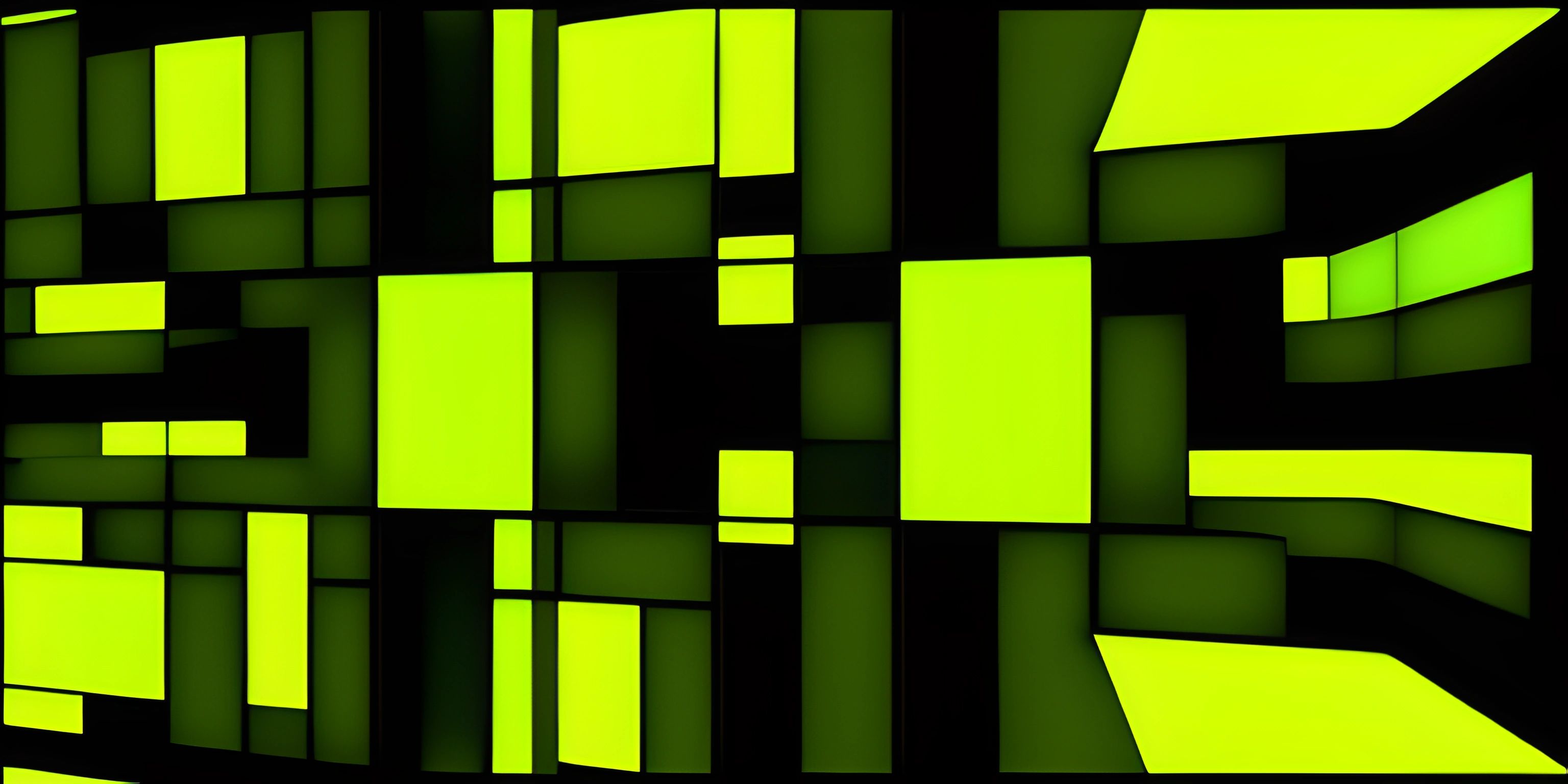
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MATLAB is a powerful and versatile programming language widely used in various fields, such as engineering, science, and finance. One of the fundamental concepts in MATLAB is the function, which allows you to organize and reuse your code. Functions are essential for keeping your code clean, modular, and efficient. In this article, we'll discuss how to create and use functions in MATLAB.
Creating Functions in MATLAB
In MATLAB, functions are defined using the function
keyword, followed by the output arguments, the function name, and the input arguments. Functions can be saved as separate files with the .m
extension, and the file name must match the function name. Here's a basic structure of a MATLAB function:
function [output_arguments] = functionName(input_arguments) % Your code here end
Let's create a simple function that adds two numbers:
function [sum] = addNumbers(a, b) sum = a + b; end
Save this function as addNumbers.m
in your working directory.
Calling Functions in MATLAB
To use a function in MATLAB, you simply call the function by its name and provide the required input arguments. In the case of our addNumbers
function, we can call it like this:
result = addNumbers(3, 4); disp(result); % This will display 7
This code snippet calls the addNumbers
function with the input arguments 3
and 4
, and stores the resulting sum in the variable result
. Then, the disp()
function is used to display the result to the console.
Multiple Input and Output Arguments
MATLAB functions can have multiple input and output arguments. Let's create a function that takes two numbers and returns their sum, difference, product, and quotient:
function [sum, difference, product, quotient] = performOperations(a, b) sum = a + b; difference = a - b; product = a * b; quotient = a / b; end
Save this function as performOperations.m
. Now, let's call this function with two numbers:
[a_sum, a_diff, a_prod, a_quot] = performOperations(5, 2); disp(a_sum); % This will display 7 disp(a_diff); % This will display 3 disp(a_prod); % This will display 10 disp(a_quot); % This will display 2.5
In this example, the performOperations
function returns four output values, which are assigned to four individual variables in the calling script.
Conclusion
Functions are a crucial building block in MATLAB programming, allowing you to create reusable and modular code. In this article, we've discussed how to create and use functions in MATLAB, as well as handle multiple input and output arguments. With this knowledge, you can now start writing more efficient and organized MATLAB programs.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using JavaScript (psst, it's free!).
FAQ
How do you create a function in MATLAB?
To create a function in MATLAB, use the function
keyword followed by the output arguments, the function name, and the input arguments. Save the function as a separate file with the same name as the function and a .m
extension.
How do you call a function in MATLAB?
To call a function in MATLAB, use the function name followed by the required input arguments in parentheses. Assign the output arguments to variables if necessary.
Can MATLAB functions have multiple input and output arguments?
Yes, MATLAB functions can have multiple input and output arguments. To create a function with multiple arguments, simply include them in the function definition separated by commas.
What is the purpose of using functions in MATLAB?
Functions in MATLAB help you organize and reuse your code, making it cleaner, more modular, and more efficient. Functions also help in breaking down complex tasks into smaller, more manageable pieces.