Rust Closures
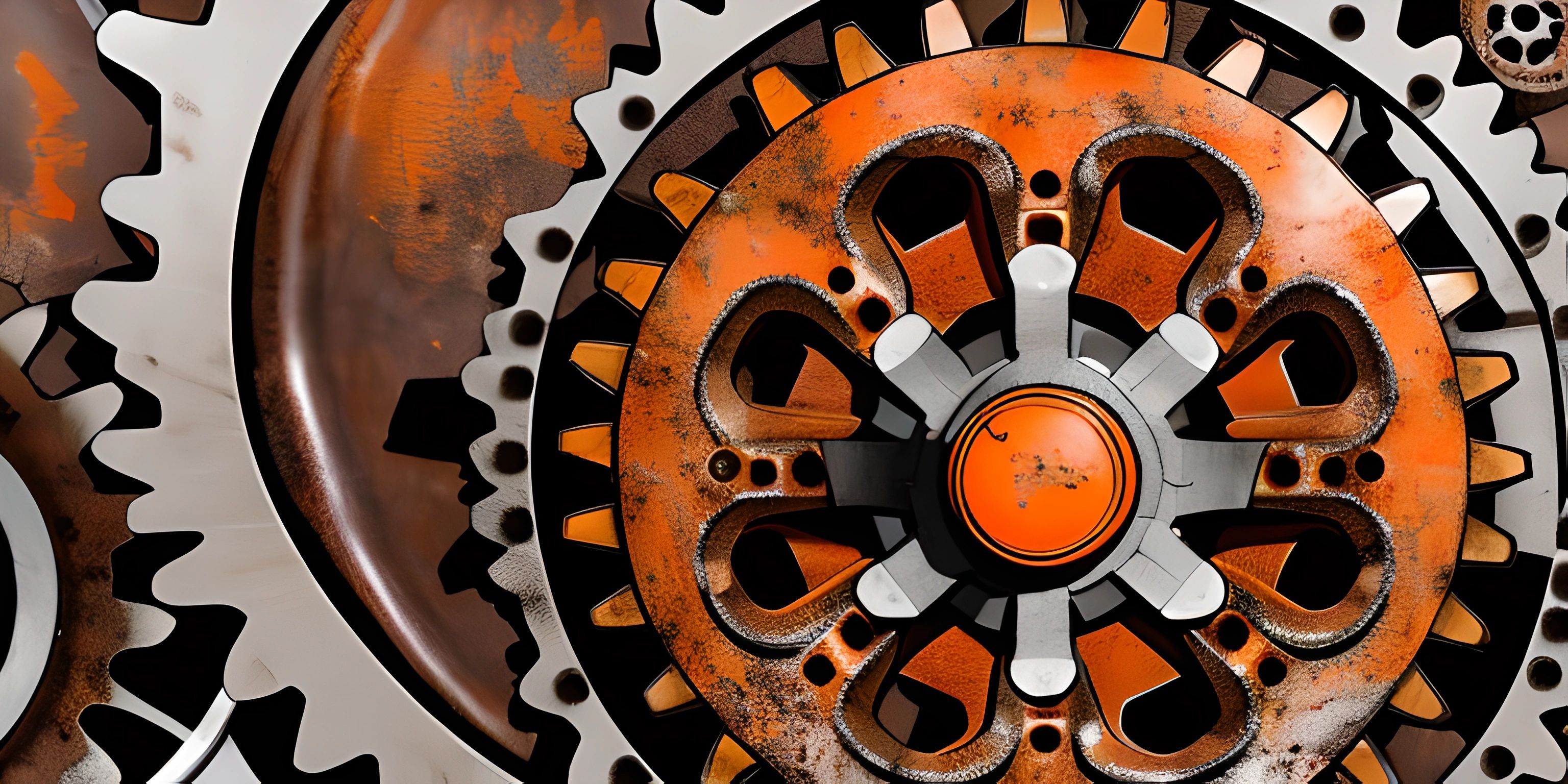
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Closures are a powerful feature in the Rust programming language that allow you to create anonymous functions. These are similar to lambdas in other languages, and they come in handy when you need a small, reusable piece of code.
What are Closures?
Closures are essentially anonymous functions that can capture their surrounding environment. They are functions without a name that can be defined and used inline. They have a few key features:
- Closures can capture values from their surrounding scope.
- Closures have a concise syntax, making them easy to use in a variety of situations.
- Rust optimizes closures for performance, so they're very efficient.
Here's an example of a closure in Rust:
let add = |x, y| x + y; println!("The sum is: {}", add(1, 2));
In this example, we define a closure called add
that takes two arguments, x
and y
, and returns their sum. We then use the println!
macro to print the result of calling the closure with arguments 1
and 2
.
Capturing the Environment
One of the most powerful features of closures is their ability to capture values from their surrounding environment. This allows you to use variables from outside the closure within the closure's body. Here's an example:
let x = 5; let add_x = |y| x + y; println!("The sum is: {}", add_x(2));
In this case, the closure add_x
captures the value of x
from its surrounding environment and uses it in the closure's body. When we call add_x(2)
, it adds 2
to the captured value of x
, resulting in an output of 7
.
Closure Types and Traits
Rust closures implement one of the following three traits, depending on how they capture variables from their environment:
Fn
: The closure captures by reference (&T
).FnMut
: The closure captures by mutable reference (&mut T
).FnOnce
: The closure captures by value (T
), consuming the captured variables.
The Rust compiler is smart enough to infer the most restrictive trait needed for your closure, but you can also explicitly specify the trait if necessary.
Higher-Order Functions and Closures
Closures are often used in conjunction with higher-order functions, which are functions that take other functions as arguments or return them. This allows for powerful and expressive code. Here's an example using the map
function:
let numbers = vec![1, 2, 3, 4, 5]; let doubled: Vec<i32> = numbers.into_iter().map(|x| x * 2).collect(); println!("Doubled numbers: {:?}", doubled);
In this example, we use a closure |x| x * 2
as an argument to the map
function, which applies the closure to each element in the numbers
vector. The result is a new vector containing the doubled values.
Conclusion
Closures are a powerful feature in Rust that enable you to write concise, expressive, and efficient code. They can capture their environment, have a simple syntax, and work seamlessly with higher-order functions. By mastering closures, you'll unlock a new level of flexibility and power in your Rust programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Async Rust (psst, it's free!).
FAQ
What is a closure in Rust?
A closure in Rust is an anonymous function that can capture its environment, making it particularly useful for tasks that require access to variables from the surrounding scope. Closures have a compact syntax and can be used as arguments, temporary functions, or even return values.
How do I define a closure in Rust?
In Rust, you can define a closure using the ||
syntax, followed by a block or an expression. The closure takes input parameters, if required, between the ||
symbols. Here's an example:
let add = |x, y| x + y; let sum = add(5, 7); println!("Sum: {}", sum);
How do closures capture their environment in Rust?
Closures in Rust can capture variables from their surrounding environment using three different methods: by reference (&T
), mutable reference (&mut T
), or by value (T
). The compiler automatically infers the capture method based on how the closure uses the variables. However, you can also manually specify the capture method using the move
keyword.
Can I use a closure as an argument to a function in Rust?
Yes, you can use a closure as an argument to a function in Rust. To do so, define the function parameter with the Fn
or FnMut
trait bound, depending on the required level of mutability. Here's an example:
fn perform_operation(operation: impl Fn(i32, i32) -> i32, x: i32, y: i32) -> i32 { operation(x, y) } let multiply = |x: i32, y: i32| x * y; let result = perform_operation(multiply, 4, 5); println!("Result: {}", result);
How do I return a closure from a function in Rust?
To return a closure from a function in Rust, use the impl
keyword along with the Fn
trait bound. You'll also need to use the move
keyword to ensure the closure captures its environment properly. Here's an example:
fn create_adder(x: i32) -> impl Fn(i32) -> i32 { move |y| x + y } let add_five = create_adder(5); let result = add_five(10); println!("Result: {}", result);