Creating and Using Functions in JavaScript
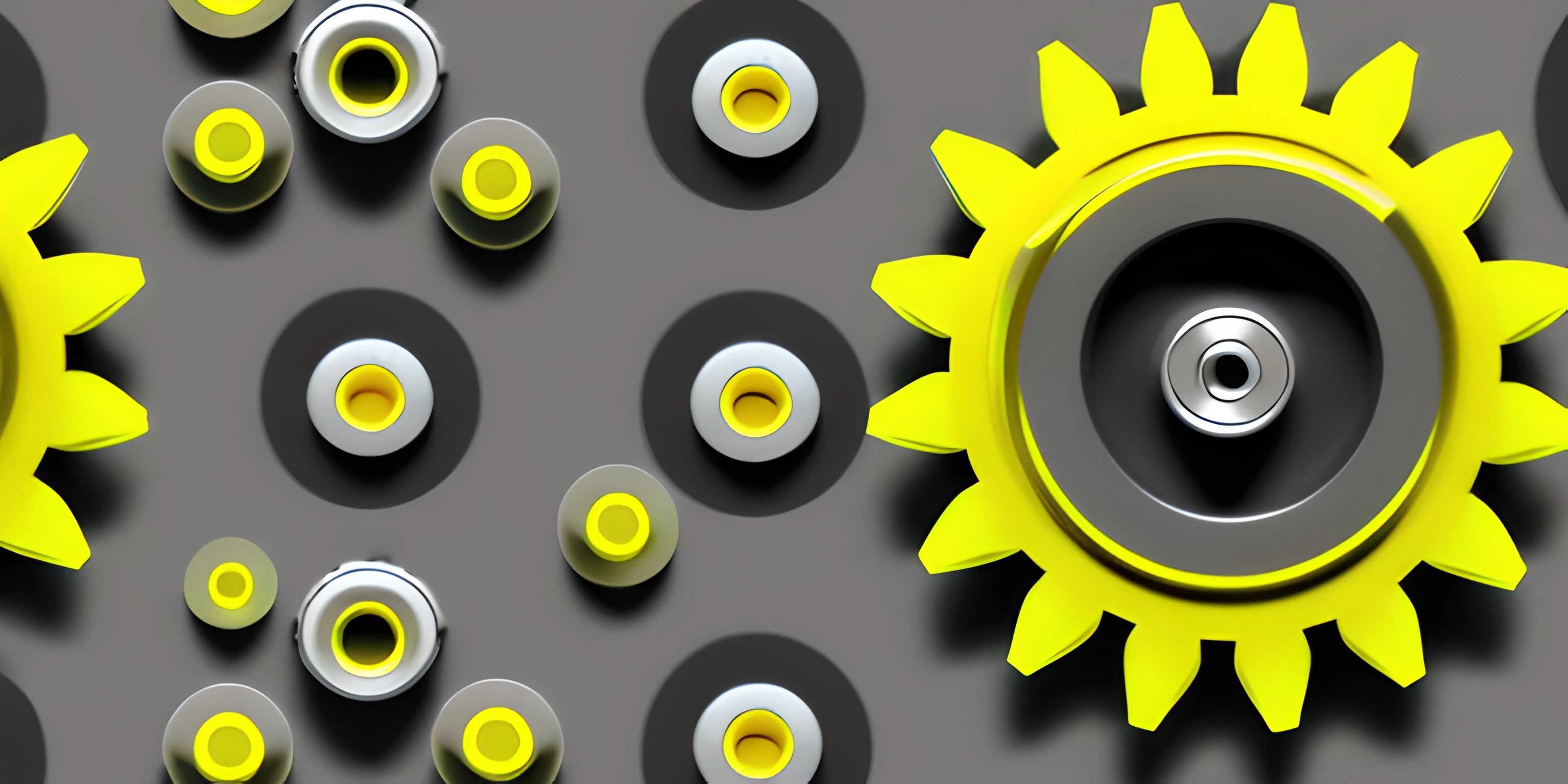
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming, one concept that consistently pops up is the mighty function. Functions are crucial for structuring your code, making it reusable, and keeping it organized. In this article, we'll focus on creating and using functions in JavaScript, a widely popular and versatile programming language.
Functions in a Nutshell
A function in JavaScript is a block of code that can be defined, called, and reused throughout your program. Functions allow you to perform the same action multiple times without duplicating code. They can also be used to break down complex tasks into smaller, more manageable pieces.
Creating a Function in JavaScript
In JavaScript, there are two primary ways to create a function: function declarations and function expressions.
Function Declarations
A function declaration is the most common way to define a function in JavaScript. It starts with the function
keyword, followed by the function name, a pair of parentheses with optional parameters, and finally, a pair of curly braces enclosing the function's code block.
Here's a simple example of a function declaration:
function greet() { console.log("Hello, Cratecoder!"); }
Function Expressions
A function expression is another way to define a function in JavaScript. In this case, a function is assigned to a variable. Function expressions can be anonymous (no name) or named. Here's an example of an anonymous function expression:
const greet = function() { console.log("Hello, Cratecoder!"); };
And here's a named function expression:
const greet = function sayHello() { console.log("Hello, Cratecoder!"); };
Calling (Invoking) Functions in JavaScript
To use a function you've created, you need to call or invoke it. To do this, simply write the function's name followed by a pair of parentheses and a semicolon. For example:
function greet() { console.log("Hello, Cratecoder!"); } greet(); // Output: "Hello, Cratecoder!"
Function Parameters and Arguments
Functions can take input values, known as parameters, which allow them to perform different actions based on the given input. When you call a function, you pass arguments that correspond to the function's parameters.
Here's an example of a function with parameters:
function greet(name) { console.log("Hello, " + name + "!"); } greet("Cratecoder"); // Output: "Hello, Cratecoder!"
You can also use multiple parameters by separating them with commas:
function greet(firstName, lastName) { console.log("Hello, " + firstName + " " + lastName + "!"); } greet("John", "Doe"); // Output: "Hello, John Doe!"
Return Values
Functions can also return a value, allowing you to use the result of the function elsewhere in your code. To return a value from a function, use the return
keyword followed by the value or expression you want to return.
Here's an example of a function that returns a value:
function add(a, b) { return a + b; } const sum = add(10, 20); console.log(sum); // Output: 30
And that wraps up our introduction to creating and using functions in JavaScript! With this newfound knowledge, you're now equipped to make your code more organized, reusable, and efficient. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using JavaScript (psst, it's free!).
FAQ
What is the basic structure of a JavaScript function?
The basic structure of a JavaScript function consists of the function
keyword, followed by the function name, a pair of parentheses ()
containing the function's parameters, and a pair of curly braces {}
containing the function's code block. Here's an example:
function greet(name) { console.log("Hello, " + name + "!"); }
How do I call a JavaScript function?
To call a JavaScript function, simply write the function name followed by the arguments enclosed in parentheses. For example, if you have a function called greet
that takes one parameter, you can call it like this:
greet("John Doe");
How can I return a value from a JavaScript function?
You can use the return
keyword to return a value from a JavaScript function. The value will be passed back to the caller of the function. Here's an example of a function that adds two numbers and returns the result:
function add(a, b) { return a + b; } var result = add(2, 3); // result will be 5
Can I have a function with no parameters in JavaScript?
Yes, you can create a function with no parameters in JavaScript. Just omit the parameters within the parentheses. Here's an example of a function that displays a greeting message without taking any parameters:
function greet() { console.log("Hello, world!"); }
What are anonymous functions and how do I create one in JavaScript?
Anonymous functions are functions without a name. They are often used as arguments for other functions or as callbacks. To create an anonymous function, just omit the function name. Here's an example of an anonymous function that is passed as an argument to the setTimeout
function:
setTimeout(function() { console.log("This message will be displayed after 2 seconds."); }, 2000);