Recursion in x86 NASM Assembly
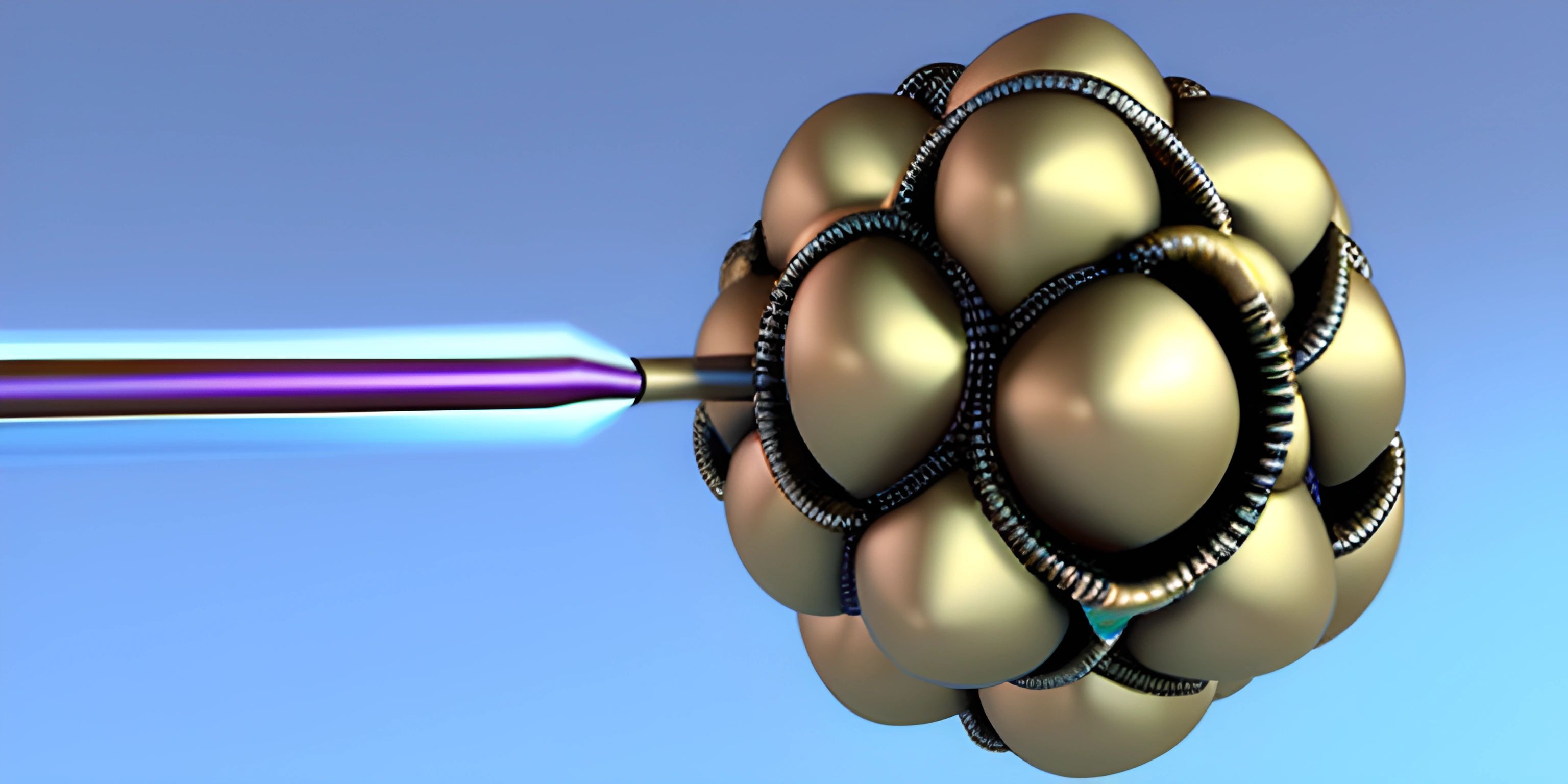
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Recursion might sound like a complicated term, but it's actually a simple concept. Recursion is a way of solving problems by breaking them down into smaller instances of the same problem, and then solving these smaller problems until you reach a base case. When working with recursion in x86 NASM assembly, things might look a bit different than when working with higher-level languages. But fear not, we'll break it down for you!
Understanding Recursion
Recursion is a programming technique where a function calls itself. It's often used to solve problems that can be divided into smaller, identical problems. Imagine having a stack of books, and you want to know the total weight of all of them. You could weigh each book individually and add the weights together, or you could weigh one book, then weigh the rest of the books as a smaller stack, and add those two weights together. This process can be repeated until only one book remains.
Recursion in x86 NASM Assembly
In x86 NASM assembly, recursion is implemented by using the call instruction to invoke a function (also known as a subroutine or procedure). When the function is called, the address of the next instruction is stored on the stack, to be used later by the ret instruction to return to the calling point. The function can then call itself in the same manner.
Let's take a look at a simple example of recursion, calculating the factorial of a number (n! = n * (n-1) * (n-2) * ... * 1). Here's a basic implementation of a recursive factorial function in x86 NASM assembly:
section .text global _start _start: ; Place the number whose factorial we want to calculate in the EAX register mov eax, 5 ; Call the recursive factorial function call factorial ; The result will be stored in the EAX register ; Exit the program mov eax, 1 xor ebx, ebx int 0x80 ; Recursive factorial function factorial: ; Base case: if EAX is 1, return 1 cmp eax, 1 je .base_case ; Recursive case: multiply EAX by the factorial of (EAX - 1) push eax ; Save the current value of EAX on the stack dec eax ; Decrement EAX by 1 call factorial ; Call the factorial function recursively ; Multiply the result by the original value of EAX pop ebx ; Retrieve the original value of EAX from the stack imul eax, ebx ; Multiply EAX by EBX (result = EAX * EBX) ret .base_case: ; If EAX is 1, return 1 mov eax, 1 ret
This example demonstrates the implementation of recursion in x86 NASM assembly. The factorial function calls itself with a decremented value of EAX until it reaches the base case (EAX = 1). The results of each recursive call are multiplied together to calculate the factorial of the original input number.
Now that you've had a taste of recursion in x86 NASM assembly, you'll be well-prepared to tackle more complex recursive problems in your assembly programming journey. Remember, practice makes perfect!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is recursion and how is it used in x86 NASM assembly language?
Recursion is a programming concept where a function calls itself in order to solve a problem. In x86 NASM assembly language, recursion can be implemented by following the steps of saving the current state, calling the function again with updated parameters, and, finally, restoring the original state before returning the result. This technique allows you to break down complex problems into smaller, more manageable tasks.
How do I implement a recursive function in x86 NASM Assembly?
To implement a recursive function in x86 NASM Assembly, follow these steps:
- Save the current state of the registers and the stack pointer.
- Update the parameters for the recursive call.
- Call the function again.
- Restore the original state of the registers and the stack pointer.
- Calculate and return the result based on the returned value from the recursive call. Here's a simple example of a recursive function to calculate the factorial of a number:
section .text global _start _start: ; Calculate 5! mov eax, 5 call factorial ; Return result in eax ; Exit the program mov eax, 1 int 0x80 factorial: ; Base case cmp eax, 1 je end_factorial ; Save registers and stack pointer pusha ; Update parameters for recursive call dec eax ; Recursive call call factorial ; Restore registers and stack pointer popa ; Calculate and return the result imul eax, [esp + 4 * 3] end_factorial: ret
How can I optimize the performance of recursive functions in x86 NASM Assembly?
Recursive functions can lead to performance issues, such as stack overflow and slow execution, if not handled properly. To optimize the performance of recursive functions in x86 NASM Assembly, you can:
- Use tail recursion when possible, which is a form of recursion that allows the compiler to optimize the code and eliminate stack overflow issues.
- Implement memoization to store the results of previously computed function calls, reducing the number of redundant calculations.
- Consider using an iterative approach instead of recursion if the problem can be solved more efficiently that way.
How do I handle stack overflow issues in recursive functions in x86 NASM Assembly?
Stack overflow issues can occur in recursive functions when the stack size exceeds the available memory. To handle stack overflow issues in x86 NASM Assembly, you can:
- Optimize the recursive function using tail recursion, which eliminates the need for additional stack frames.
- Increase the stack size by adjusting the stack frame allocation or the program's linker settings.
- Use an iterative approach instead of recursion if it's more suitable for the problem at hand.
- Implement memoization to reduce the depth of the recursion tree and the number of stack frames needed.