NASM Tutorial
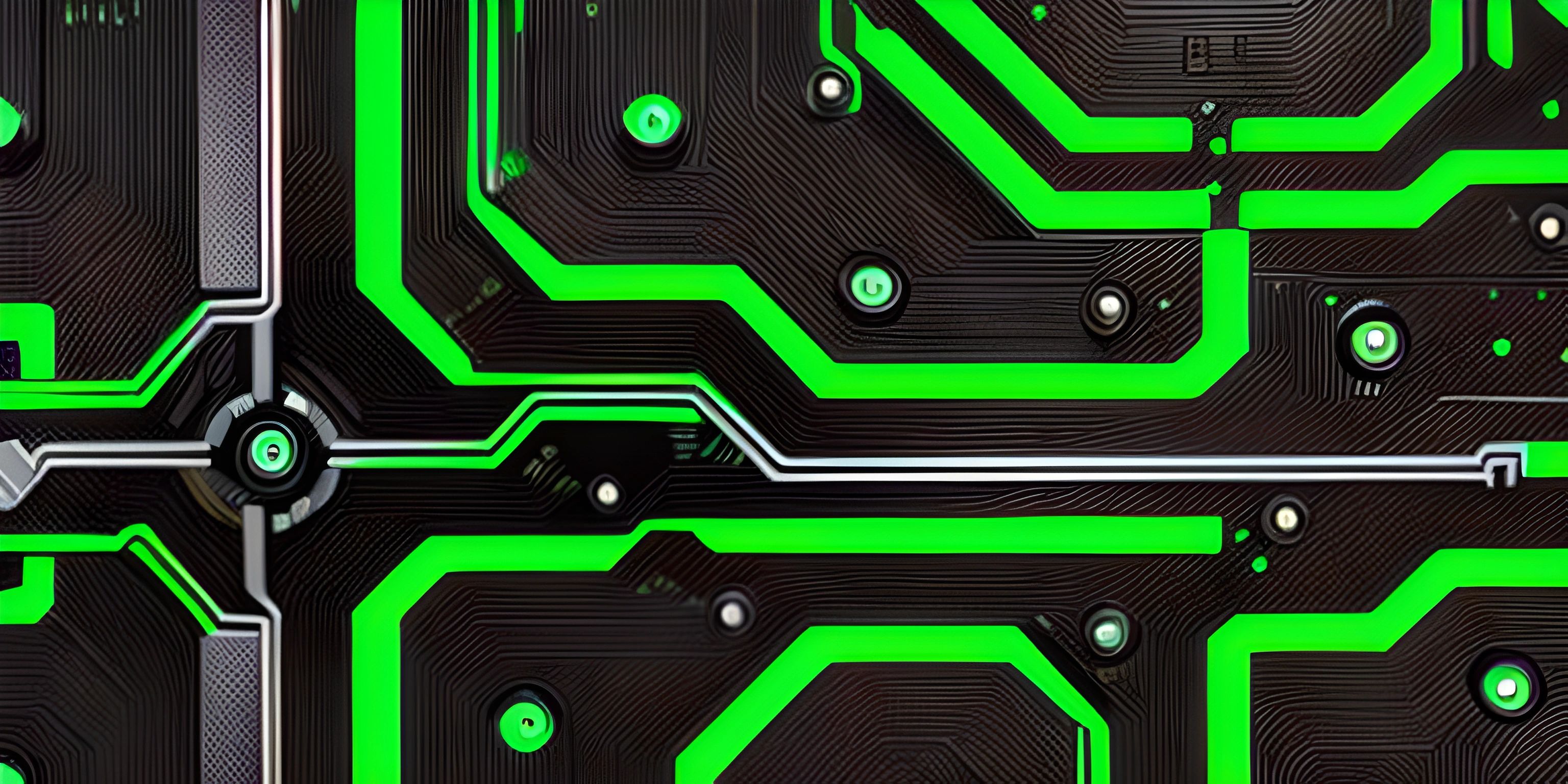
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of assembly! In this article, we'll be diving into the Netwide Assembler (NASM), an assembler for the Intel x86 and x64 architectures. We'll cover its syntax, basic instructions, and how to create a simple program using NASM.
What is NASM?
The Netwide Assembler (NASM) is a popular assembler that translates assembly language into machine code for the Intel x86 and x64 architectures. NASM is open source and has a simple syntax, making it a great starting point for learning assembly.
NASM Syntax
NASM uses a simple and readable syntax. Here's a quick overview of what you'll commonly see:
- Instructions: Assembly instructions are written in mnemonic form, like
mov
,add
, orjmp
. These tell the processor what action to perform. - Registers: Registers are small and fast storage spaces within the processor. Examples include
eax
,ebx
, andecx
(for x86) orrax
,rbx
, andrcx
(for x64). - Operands: Instructions often have operands, which are the values that the instruction operates on. They can be registers, memory addresses, or immediate values (like a number).
An example of a NASM instruction with operands:
mov eax, 1 ; Move the value 1 into the eax register
- Comments: Comments in NASM start with a semicolon (
;
). Everything after the semicolon on the same line will be ignored by the assembler.
Basic NASM Instructions
Here are some basic NASM instructions you'll use frequently:
mov
: Move a value from one location to another (e.g.,mov eax, 1
).add
: Add two values (e.g.,add eax, ebx
).sub
: Subtract two values (e.g.,sub eax, ebx
).mul
: Multiply two values (e.g.,mul eax, ebx
).div
: Divide two values (e.g.,div eax, ebx
).jmp
: Jump to a specific location in the code (e.g.,jmp label
).
Creating a Simple Program with NASM
To create a simple NASM program, follow these steps:
-
Install NASM: Download and install NASM from the official website.
-
Write the Assembly Code: Create a new file called
hello.asm
and add the following code:
section .data helloMessage db 'Hello, World!', 0 section .text global _start _start: ; Write the helloMessage to STDOUT mov eax, 4 mov ebx, 1 lea ecx, [helloMessage] mov edx, 13 int 0x80 ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
This code writes the "Hello, World!" message to STDOUT (the terminal) and then exits the program.
- Assemble the Code: Run the following command to assemble the code into an object file:
nasm -f elf hello.asm
- Link the Object File: Link the object file to create an executable:
ld -m elf_i386 -s -o hello hello.o
- Run the Program: Finally, run the program:
./hello
You should see "Hello, World!" printed to the terminal. Congratulations, you've created your first program with NASM!
Now you're ready to explore more complex assembly programs and dive deeper into the world of low-level programming with NASM. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Lifetimes (psst, it's free!).
FAQ
What is NASM and why should I use it?
NASM, or the Netwide Assembler, is an assembler and disassembler for the Intel x86 architecture. It's a popular choice among programmers because of its simple syntax, flexibility, and wide support for various platforms. Using NASM, you can create efficient, low-level programs and dive deep into the inner workings of your computer.
How do I get started with NASM syntax?
NASM syntax is straightforward, with each instruction typically consisting of an operation (mnemonic) followed by its operands. Operands can be registers, memory addresses, or immediate values. Here's an example of NASM syntax:
mov eax, 1 add eax, ebx
In this example, mov
and add
are the mnemonics, while eax
, 1
, and ebx
are the operands.
What are some basic NASM instructions?
Some commonly used NASM instructions include:
mov
: Move data between registers or memory locations.add
andsub
: Perform addition and subtraction, respectively.inc
anddec
: Increment and decrement a register or memory location by one.push
andpop
: Add or remove data from the stack.call
andret
: Call a subroutine and return from it.
Can you provide a simple example of a NASM program?
Sure! Here's a basic NASM program that adds two integers and stores the result in a register:
section .data num1 dd 5 num2 dd 3 section .text global _start _start: ; Load values into registers mov eax, [num1] mov ebx, [num2] ; Add the values add eax, ebx ; Exit the program mov eax, 1 int 0x80
In this example, we define two integers in the data section, load their values into registers, add them together, and then exit the program.
How do I assemble and run a NASM program?
To assemble and run a NASM program, follow these steps:
- Write your NASM code in a text file with a .asm extension (e.g.,
my_program.asm
). - Assemble the source code using the NASM assembler:
nasm -f elf32 my_program.asm -o my_program.o
- Link the object file to create an executable:
ld -m elf_i386 my_program.o -o my_program
- Run the compiled executable:
./my_program
These steps assume you're using a 32-bit system. For 64-bit systems, replace elf32
with elf64
and elf_i386
with elf_x86_64
.