An Introduction to the Netwide Assembler (NASM)
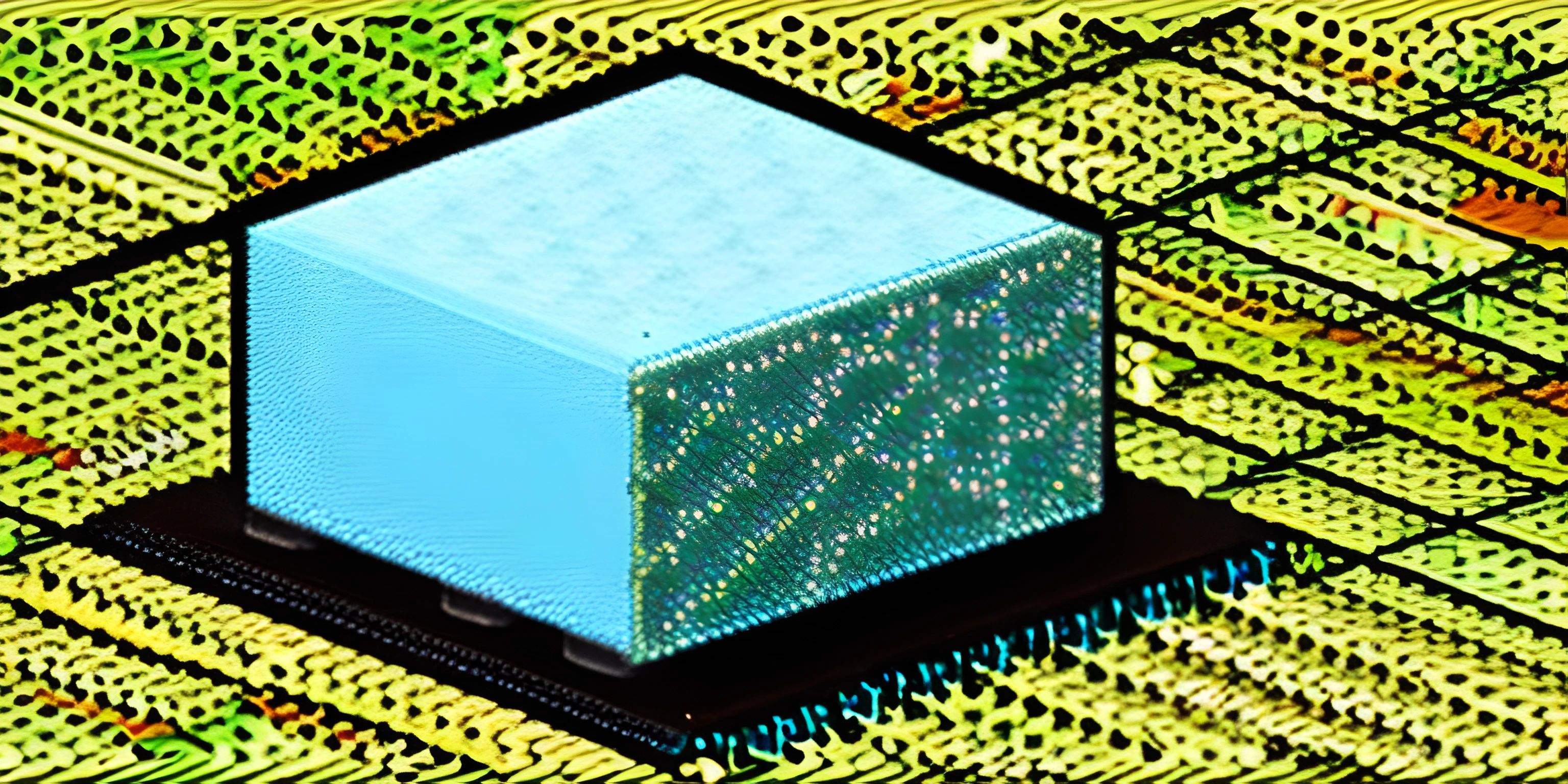
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When you think about programming, you might imagine high-level languages like Python, JavaScript, or Java. However, there's a whole other world of programming that exists beneath the surface: assembly language programming. In this realm, we tinker directly with the nuts and bolts of a computer's hardware, and one of the most popular tools for that is the Netwide Assembler (NASM).
What is Assembly Language?
Before diving into NASM, let's briefly discuss what assembly language is. It is a low-level programming language that is specific to a computer's architecture. Unlike high-level languages, which are abstracted from the hardware, assembly language allows you to manipulate the computer's memory, registers, and other components directly.
What is NASM?
NASM is an assembler that translates assembly language code into machine code for x86 and x86-64 architectures. It is open-source, free, and widely used for low-level programming tasks, such as writing operating systems, bootloaders, and device drivers.
One of the key features of NASM is its flexible syntax, which makes it easy to understand and write assembly code. It supports various output formats, including raw binary, ELF, and COFF, making it suitable for different platforms and purposes.
Getting Started with NASM
To begin your journey into NASM, you'll need to install the assembler itself. You can find the latest version and installation instructions on the official NASM website.
Once you have NASM installed, create a new text file and save it with an .asm
extension. This will be your assembly source code file. Let's write a simple "Hello, World!" program:
; This is a comment in NASM section .data hello db 'Hello, World!', 0Ah section .text global _start _start: ; Write Hello, World! to the screen mov eax, 4 mov ebx, 1 lea ecx, [hello] mov edx, 13 int 0x80 ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
This code writes "Hello, World!" to the screen and then exits. You can assemble and run it using the following commands:
nasm -f elf64 hello.asm -o hello.o ld -o hello hello.o ./hello
The first command assembles the source code into an object file, the second links the object file into an executable, and the third runs the executable. VoilĂ ! You've just written and executed your first NASM program.
Learning More
Now that you've had a taste of NASM and assembly language programming, there's a whole world waiting to be explored. Some topics to dive into include:
As you delve deeper into NASM and assembly programming, you'll gain a greater appreciation for the inner workings of a computer and develop a new perspective on programming. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is NASM and why is it popular for assembly language programming?
The Netwide Assembler (NASM) is an assembler and disassembler for the Intel x86 and x86-64 architectures. It is popular for assembly language programming due to its simple syntax, cross-platform compatibility, and comprehensive documentation. NASM has a wide range of applications, such as operating systems, bootloaders, and low-level system utilities.
How do I install NASM on my computer?
To install NASM, follow the instructions for your specific operating system:
-
Windows: Download the latest NASM release from the official website and follow the installation instructions provided in the download.
-
macOS: Use Homebrew to install NASM by running the command:
brew install nasm
-
Linux: Use your distribution's package manager to install NASM. For example, on Ubuntu, run the command:
sudo apt-get install nasm
Can you provide a simple NASM program example?
Sure! Here's a simple "Hello, World!" program written in NASM assembly language:
section .data hello db 'Hello, World!',0 section .text global _start _start: ; Write "Hello, World!" to stdout mov eax, 4 mov ebx, 1 lea ecx, [hello] mov edx, 13 int 0x80 ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
This program writes "Hello, World!" to the standard output and then exits.
How do I assemble and run a NASM program?
To assemble and run a NASM program, follow these steps:
-
Save your assembly code to a file with the
.asm
extension, e.g.,hello.asm
. -
Use NASM to assemble the program:
nasm -f elf64 hello.asm -o hello.o
This command generates an object file
hello.o
from thehello.asm
source file. -
Link the object file to create an executable:
ld hello.o -o hello
This command creates an executable file named
hello
. -
Run the executable:
./hello
This command runs the
hello
executable, displaying the output of your program.
What are some resources to learn assembly language programming with NASM?
There are several resources available to help you learn assembly language programming with NASM:
- NASM Official Documentation: A comprehensive guide to NASM, including its syntax, directives, and standard library.
- Programming from the Ground Up: A free ebook that teaches assembly language programming using NASM and the GNU toolchain.
- Online tutorials and blog posts: There are numerous tutorials and blog posts available on the internet that cover various aspects of NASM and assembly language programming.
- Community forums and discussion boards: Engage with other NASM users and assembly language enthusiasts to share knowledge, ask questions, and discuss relevant topics.