Examples of Using fs.readFile in Node.js
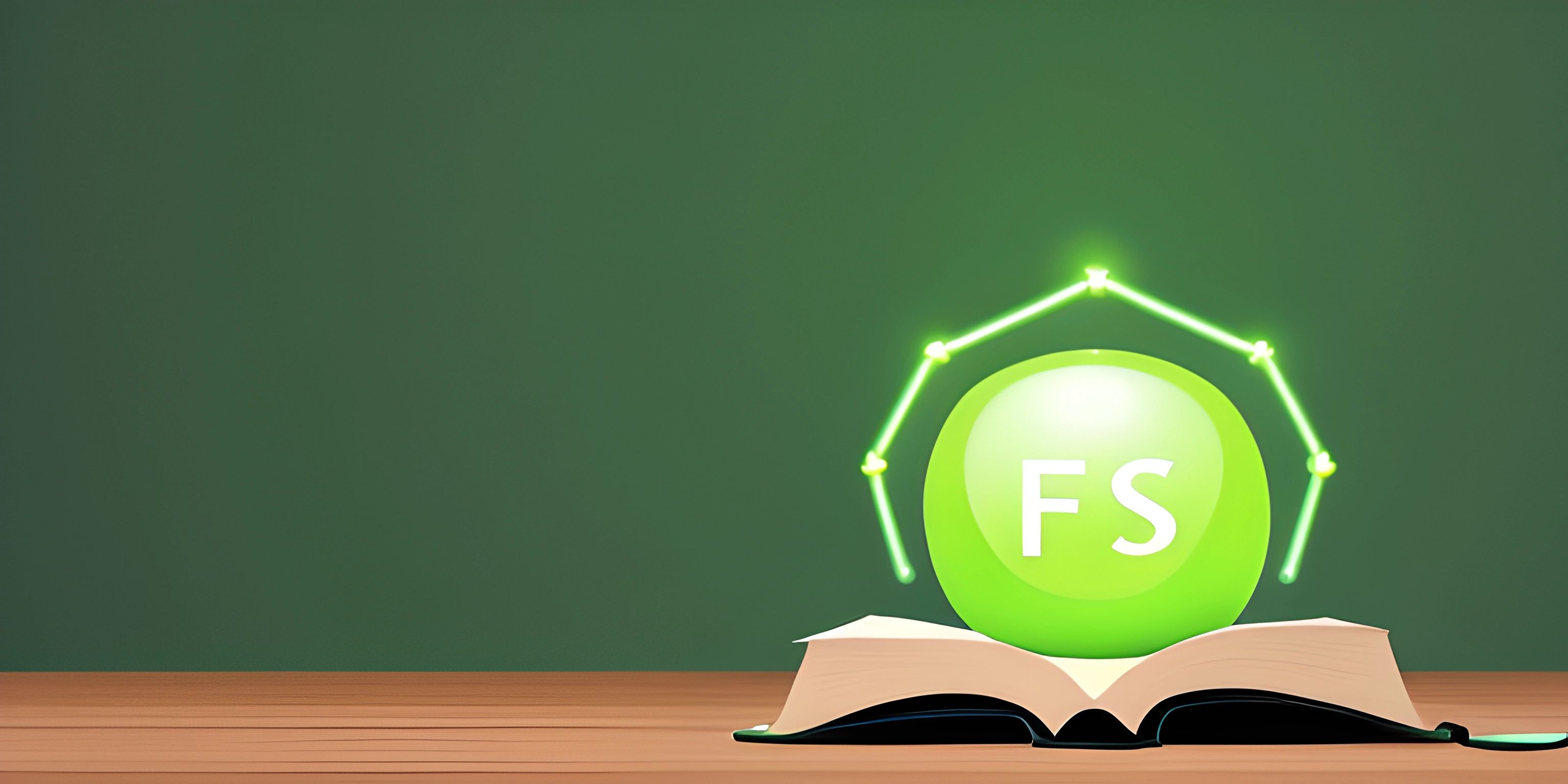
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In this article, we will discuss how to use the fs.readFile
method in Node.js for reading files asynchronously. The fs
module is built into Node.js and provides an API for interacting with the file system.
Basic Usage of fs.readFile
Here's a simple example of using fs.readFile
to read a file named example.txt
:
const fs = require("fs"); fs.readFile("example.txt", "utf8", (err, data) => { if (err) { console.error("Error reading file:", err); return; } console.log("File content:", data); });
In this example, we first require the fs
module. Then, we use the fs.readFile
method to read the content of the example.txt
file. The method takes three arguments:
- The file path (relative or absolute) of the file to read.
- The file encoding (optional) - in this case, we've specified
"utf8"
to read the file as text. - A callback function that will be called when the file is read or an error occurs.
Reading a File without Specifying Encoding
If you don't provide an encoding to fs.readFile
, the data will be returned as a Buffer
object instead of a string. You can then convert the buffer to a string using the toString
method, like this:
const fs = require("fs"); fs.readFile("example.txt", (err, data) => { if (err) { console.error("Error reading file:", err); return; } console.log("File content:", data.toString()); });
Error Handling with fs.readFile
When using fs.readFile
, it's important to handle any errors that may occur. In the examples above, we've used a simple if
statement to check if there's an error and log it to the console. However, you may want to implement more advanced error handling depending on your application's requirements.
For example, you could use a try-catch
block with async/await
and fs.promises.readFile
:
const fs = require("fs").promises; async function readFileAsync(filePath) { try { const data = await fs.readFile(filePath, "utf8"); console.log("File content:", data); } catch (err) { console.error("Error reading file:", err); } } readFileAsync("example.txt");
In this example, we've wrapped the fs.readFile
method in an async function and used try-catch
to handle errors.
With these examples, you should now have a good understanding of how to use the fs.readFile
method in Node.js for reading files asynchronously.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: File I/O (Reading) (psst, it's free!).
FAQ
What is fs.readFile and how does it work in Node.js?
fs.readFile
is a function in the Node.js fs
(file system) module that allows you to read files asynchronously. It takes the file path and an optional encoding, reads the entire file, and then returns its content via a callback function. Here's a basic example:
const fs = require("fs"); fs.readFile("example.txt", "utf8", (err, data) => { if (err) throw err; console.log(data); });
What are the advantages of using fs.readFile over fs.readFileSync?
While fs.readFileSync
reads files synchronously, fs.readFile
reads them asynchronously. This means that your Node.js application won't be blocked while reading the file, allowing it to continue processing other tasks. Asynchronous file reading is especially helpful when dealing with large files, as it prevents your application from becoming unresponsive.
How do I handle errors when using fs.readFile?
When using fs.readFile
, errors can be handled within the callback function. The first argument of the callback is an error object (err
). If an error occurs, this object will contain the details, and you can handle the error accordingly. Here's an example:
const fs = require("fs"); fs.readFile("example.txt", "utf8", (err, data) => { if (err) { console.error("An error occurred:", err); return; } console.log(data); });
Can I read binary files using fs.readFile?
Yes! fs.readFile
can be used to read binary files as well. When reading a binary file, you can omit the encoding parameter, and the function will return a Buffer
object containing the raw binary data. Here's an example:
const fs = require("fs"); fs.readFile("example.bin", (err, data) => { if (err) throw err; console.log(data); });
How can I convert the Buffer object returned by fs.readFile for binary files into a different encoding?
After reading a binary file using fs.readFile
, you can convert the returned Buffer
object into a different encoding using the toString()
method. For example, to convert the buffer into a UTF-8 encoded string, you can use data.toString("utf8")
:
const fs = require("fs"); fs.readFile("example.bin", (err, data) => { if (err) throw err; const utf8string = data.toString("utf8"); console.log(utf8string); });