Examples of using fs.readFileSync in Node.js
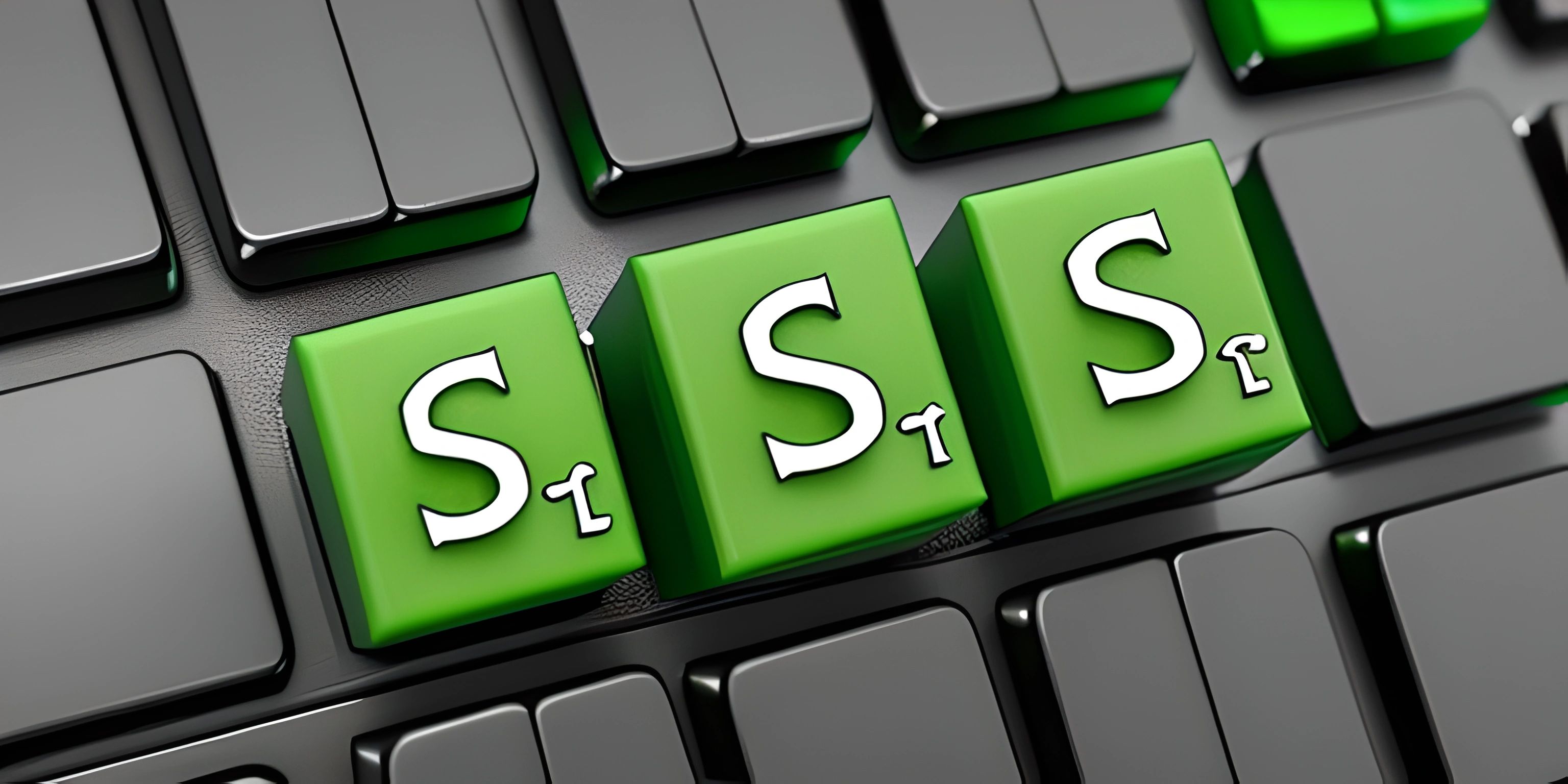
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The fs.readFileSync
method is a part of the fs
(file system) module in Node.js. It allows you to read the entire content of a file synchronously, meaning that the function will block the execution of the rest of the code until the file is completely read. This can be useful in cases where you need to read a file before proceeding with other operations in your code.
In this article, we will go through examples of using fs.readFileSync
in Node.js.
Basic Usage
First, you need to import the fs
module:
const fs = require("fs");
Next, use the fs.readFileSync
method to read a file:
const data = fs.readFileSync("example.txt", "utf-8"); console.log(data);
In this example, we read the content of the file example.txt
and store it in the data
variable. The second argument, "utf-8"
, specifies the encoding used to read the file. If you don't provide an encoding, the method will return a Buffer
object instead of a string.
Handling Errors
When using fs.readFileSync
, it's important to handle any errors that may occur, such as the file not being found. You can use a try-catch block to catch any errors:
const fs = require("fs"); try { const data = fs.readFileSync("nonexistent.txt", "utf-8"); console.log(data); } catch (error) { console.error("Error reading file:", error); }
In this example, we try to read a non-existent file, and the catch block handles the error by logging it to the console.
Reading a JSON File
You can use fs.readFileSync
to read a JSON file and parse its content:
const fs = require("fs"); try { const data = fs.readFileSync("example.json", "utf-8"); const jsonData = JSON.parse(data); console.log(jsonData); } catch (error) { console.error("Error reading JSON file:", error); }
In this example, we read a file named example.json
, and then parse its content using JSON.parse()
.
Remember that using synchronous methods in Node.js can block the event loop and affect the performance of your application. It's recommended to use asynchronous methods, such as fs.readFile
, in most cases. However, using fs.readFileSync
can be suitable for small scripts or situations where you need to read a file before proceeding with the rest of your code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: File I/O (Reading) (psst, it's free!).
FAQ
What is fs.readFileSync in Node.js?
fs.readFileSync
is a method in the built-in fs
(file system) module in Node.js. It is used to read the contents of a file synchronously, meaning it blocks other operations until the file reading is complete. The method returns the data from the file as a buffer or a string, depending on the specified encoding.
How do I use fs.readFileSync in my code?
To use fs.readFileSync
, you first need to import the fs
module using require
. Then, simply call the fs.readFileSync
method with the file path as the first argument and, optionally, the file encoding as the second argument. Here's an example:
const fs = require("fs"); // Read the file contents as a buffer const data = fs.readFileSync("example.txt"); console.log(data); // Output: <Buffer 48 65 6c 6c 6f 2c 20 77 6f 72 6c 64 21> // Read the file contents as a string const dataString = fs.readFileSync("example.txt", "utf8"); console.log(dataString); // Output: Hello, world!
Can I specify different encodings when using fs.readFileSync?
Yes, you can specify different encodings when using fs.readFileSync
. The most common encoding is utf8
, but you can also use other encodings such as ascii
, base64
, hex
, and more. To specify an encoding, pass it as the second argument to the method:
const fs = require("fs"); const data = fs.readFileSync("example.txt", "ascii"); console.log(data); // Output: Hello, world!
What happens if the file doesn't exist or there's an error while reading the file?
If the file doesn't exist or there's an error while reading the file, fs.readFileSync
will throw an error. To handle this, you can use a try-catch
block:
const fs = require("fs"); try { const data = fs.readFileSync("nonexistent.txt", "utf8"); console.log(data); } catch (error) { console.error("Error reading file:", error); }
Are there any performance implications of using fs.readFileSync?
Yes, since fs.readFileSync
is a synchronous operation, it blocks other tasks in the event loop while it reads the file. This can impact the performance of your application, especially when reading large files. For better performance, consider using the asynchronous counterpart fs.readFile
instead.