p5.js Introduction
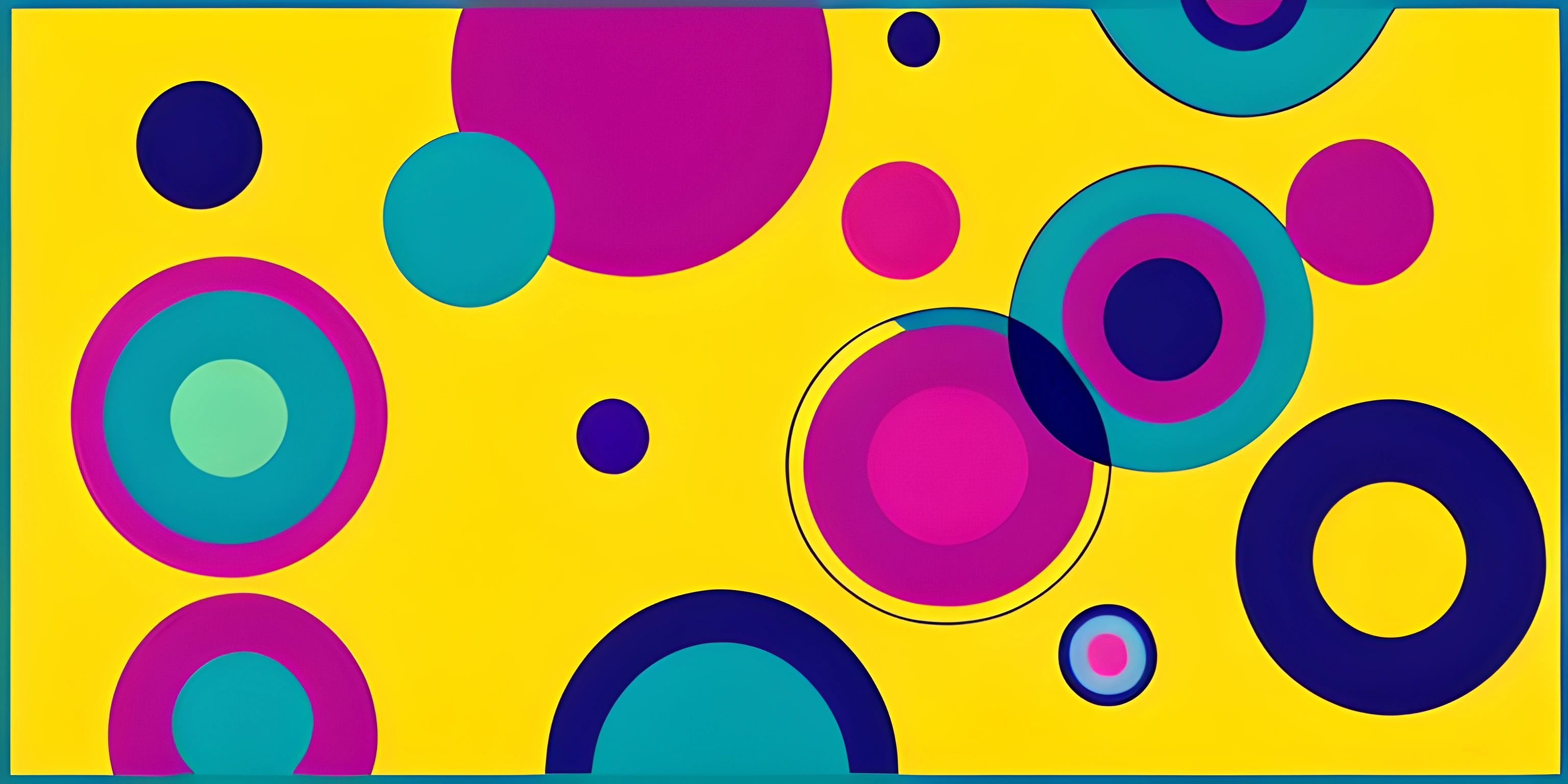
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
p5.js is a JavaScript framework that makes coding visual and interactive elements a breeze. It was created as a spiritual successor to the popular Processing programming language, with the goal of bringing similar capabilities to the web.
Features
p5.js is designed around the idea of making it easy to create graphics, animations, and interactivity in a browser. Some of its most notable features include:
- A simple and easy-to-understand syntax
- A powerful drawing and animation API (Application Programming Interface)
- A built-in library for handling input (mouse, keyboard, etc.)
- Support for creating and managing HTML elements
- A thriving community and a vast collection of examples and libraries
The p5.js Sketch
At the core of p5.js is the concept of a sketch, which is a single JavaScript file that describes your program. A basic p5.js sketch consists of two main functions: setup()
and draw()
.
The setup()
function is executed once when the program starts and is typically used for initializing variables, creating a canvas, and setting up any necessary HTML elements.
The draw()
function, on the other hand, is executed continuously in a loop, allowing you to create animations and respond to user input. Inside the draw()
function, you can use various p5.js functions to draw shapes, handle input, and manipulate the canvas.
Here's a simple example of a p5.js sketch that draws a moving ellipse:
function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(mouseX, mouseY, 50, 50); }
In this example, the createCanvas
function sets up a 400x400 pixel canvas, while the background
function clears the canvas each frame with a light gray color. The ellipse
function then draws an ellipse at the current mouse position with a width and height of 50 pixels.
Getting Started
To start using p5.js, you can either download the library and include it in your HTML file, or simply reference it from a CDN (Content Delivery Network) like so:
<script src="https://cdn.jsdelivr.net/npm/p5"></script>
Once you've included the p5.js library in your project, you can start creating your sketch by adding a new JavaScript file and linking it in your HTML file.
Whether you're a beginner or a seasoned programmer, p5.js offers a fun and accessible way to explore the world of creative coding. Its intuitive API and extensive documentation make it a great choice for learning programming concepts, creating art, or developing interactive web applications. So, why not give it a try? The possibilities are endless, and the p5.js community is always there to help and inspire you along the way!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is p5.js?
p5.js is an open-source JavaScript framework that makes it easy to create graphics and interactive art in the browser. It's built on top of the native HTML5 canvas and WebGL, and provides a user-friendly API for drawing shapes, colors, and animations.
Why should I use p5.js?
p5.js is perfect for artists, designers, educators, and beginners who want to create visually engaging and interactive content in the browser. With its easy-to-learn syntax and extensive documentation, you can quickly dive into the world of creative coding without needing extensive programming experience.
How do I get started with p5.js?
To get started with p5.js, you need to include its library in your HTML file. You can either download the library from the p5.js website or use a CDN link. Then, create a JavaScript file where you'll write your p5.js code. Here's a basic example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>p5.js Example</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> <script src="your-p5js-code.js"></script> </head> <body> </body> </html>
What are the basic building blocks of p5.js?
p5.js revolves around two main functions: setup()
and draw()
. The setup()
function is called once at the beginning of your program and is used to set up the canvas, define initial variables, and load assets. The draw()
function is called continuously in a loop and is where you write the code to create your graphics and animations. Here's an example:
function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(mouseX, mouseY, 50, 50); }
Are there any resources to help me learn p5.js?
Absolutely! The p5.js website offers extensive documentation, tutorials, and examples to help you get started. You can also find many p5.js learning resources on YouTube, such as The Coding Train channel, which features a popular video series called "Introduction to p5.js".