Drawing Primitives in p5.js
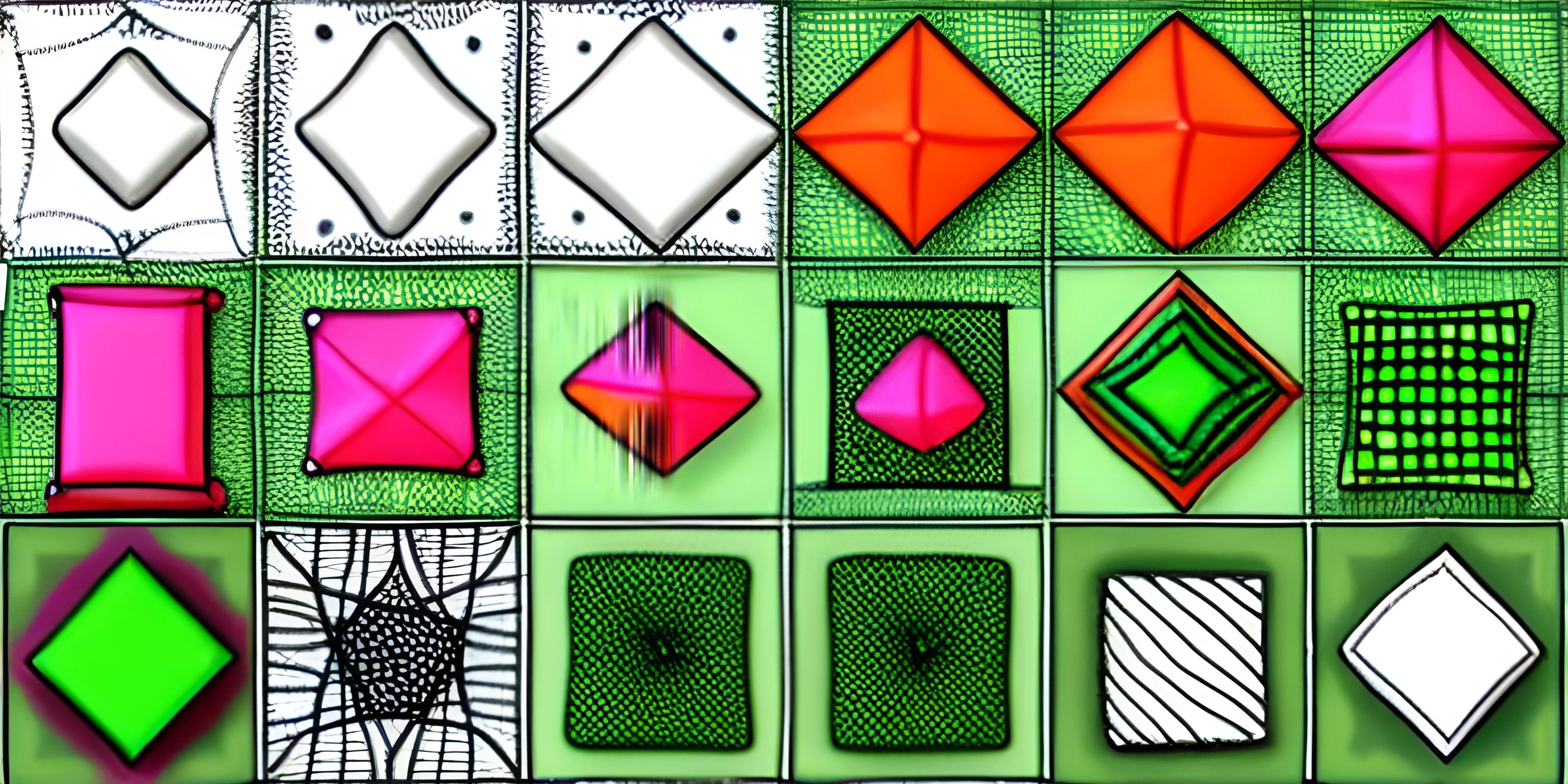
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When you're just getting started with p5.js, a JavaScript library with a full set of drawing functionality, one of the first things you'll want to do is start drawing shapes. In p5.js, these shapes are known as primitives. Primitives are the building blocks of your p5.js sketches, the basic elements that you can combine and manipulate to create more complex visuals.
Primitives List
p5.js offers an array of primitives for you to play with. These include:
- Points
- Lines
- Rectangles
- Circles
- Ellipses
- Triangles
- Quadrilaterals
Points and Lines
The simplest primitives are points and lines. You might think of a point as the most basic shape, just a single spot in space. In p5.js, you can draw a point using the point()
function:
function draw() { point(30, 20); }
This would draw a point at the position (30, 20). A line, on the other hand, is simply a connection between two points. You can draw a line using the line()
function:
function draw() { line(30, 20, 80, 60); }
This would draw a line from the point (30, 20) to the point (80, 60).
Rectangles and Circles
Moving on to 2D shapes, we have rectangles and circles. To draw a rectangle in p5.js, you'd use the rect()
function, like so:
function draw() { rect(30, 20, 50, 100); }
This would draw a rectangle with the top-left corner at (30, 20), a width of 50, and a height of 100. Circles are similar, but instead of width and height, you specify a diameter. Use the circle()
function for that:
function draw() { circle(30, 20, 50); }
This draws a circle with the center at (30, 20) and a diameter of 50.
Other Shapes
There are other shapes to explore in p5.js as well, like ellipses, triangles, and quadrilaterals. Once you've mastered the basics, feel free to dive deeper into these more complex primitives. The sky's the limit!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
What are primitives in p5.js?
Primitives are basic shapes provided by p5.js that you can use to create your own drawings, including points, lines, rectangles, circles, ellipses, triangles, and quadrilaterals.
How do I draw a rectangle in p5.js?
To draw a rectangle in p5.js, use the rect()
function. This function requires four arguments: the x and y coordinates of the top-left corner of the rectangle, followed by the width and height of the rectangle.
Can I draw a triangle in p5.js?
Yes! You can draw a triangle using the triangle()
function in p5.js. You will need to specify the x and y coordinates for each of the three vertices of the triangle as arguments to this function.
Can I change the color of the primitives I draw?
Absolutely yes! p5.js provides a variety of functions to manipulate the color and style of the primitives you draw. You can use the fill()
function to set the color of the shapes, stroke()
to set the color of the outline, and noStroke()
to draw shapes with no outline.
Can I create complex shapes using primitives in p5.js?
Most certainly. Primitives are the building blocks of your p5.js sketches. You can create almost any shape you can imagine by combining and manipulating these basic shapes. It might require a bit of imagination, but with practice, you can create some truly stunning visuals.