Introduction to p5.js
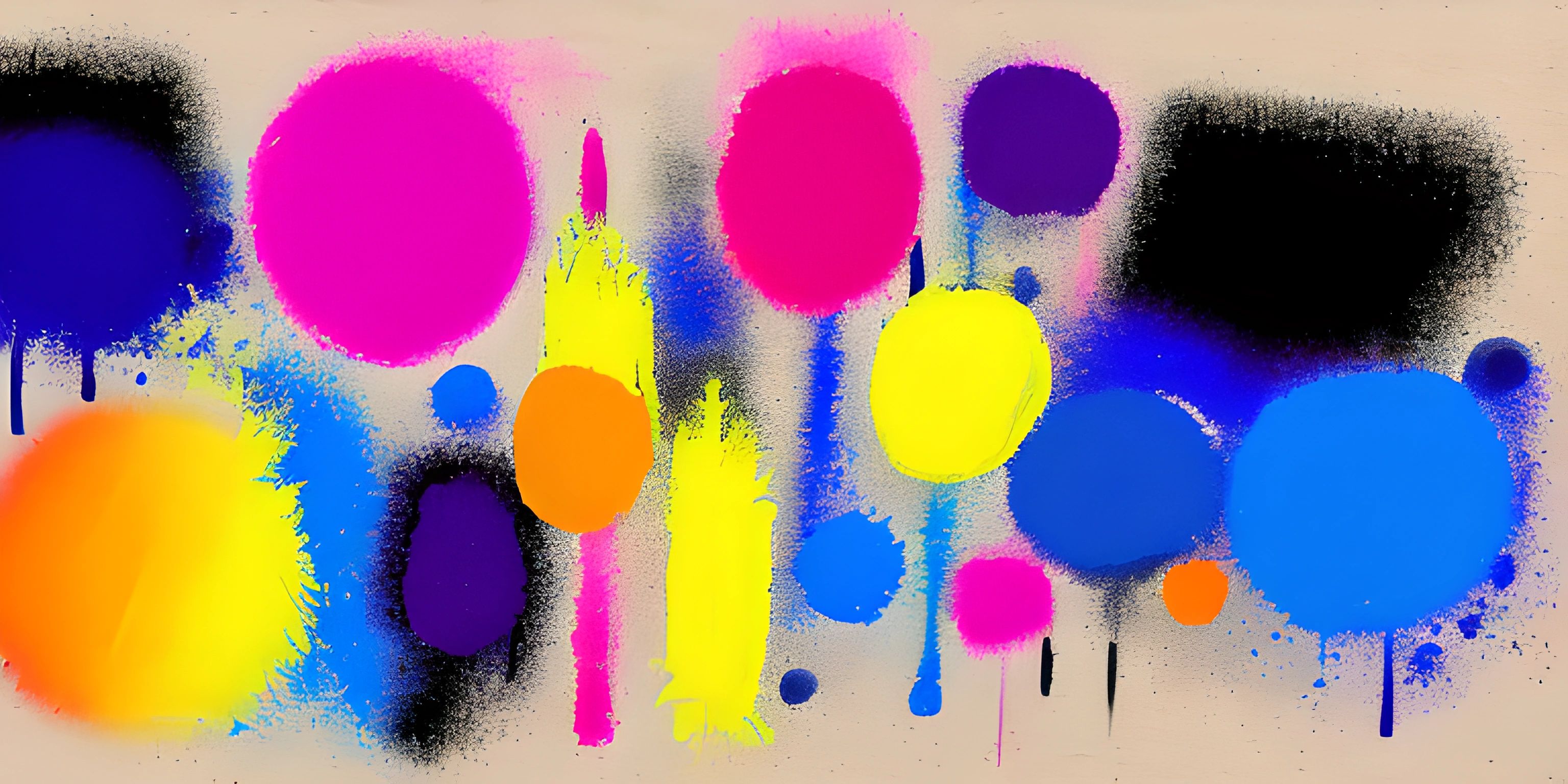
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
p5.js is a powerful and versatile JavaScript library that makes it easy to create interactive digital art. Born from the philosophy of Processing, p5.js expands upon those ideas to bring the power of creative coding to the web.
What is p5.js?
p5.js is an open-source library that facilitates the creation of graphics and interactive applications in a simple and intuitive way. It provides a set of functions and objects that help you draw shapes, manipulate colors, handle user input, and more, all within the context of the HTML5 canvas.
Getting Started with p5.js
To begin using p5.js, you'll need to include the library in your HTML file. You can either download it directly or link to the latest version using a CDN, like so:
<!DOCTYPE html> <html> <head> <script src="https://cdn.jsdelivr.net/npm/p5"></script> </head> <body> <script src="sketch.js"></script> </body> </html>
Now, you'll want to create your sketch.js
file, which is where your p5.js code will live.
The p5.js Lifecycle
In a p5.js sketch, there are two main functions you'll use: setup()
and draw()
.
setup()
: This function runs once when the sketch starts. It's a great place to set up initial configurations, such as the canvas size and background color.draw()
: This function runs in a loop, updating and redrawing your sketch every frame. This is where you'll put the code that creates and animates your digital art.
Here's a simple example of a p5.js sketch:
function setup() { createCanvas(800, 600); background(200); } function draw() { if (mouseIsPressed) { fill(255); } else { fill(0); } ellipse(mouseX, mouseY, 80, 80); }
This sketch creates a canvas with a light gray background. When you click and drag your mouse, it will draw black circles, which turn white when the mouse is pressed.
Exploring p5.js Functions
p5.js offers a wide array of functions to help you create and manipulate graphics. Here are a few popular ones to get you started:
createCanvas(width, height)
: Creates a canvas of the specified size.background(color)
: Sets the background color of the canvas.fill(color)
: Sets the color used to fill shapes.stroke(color)
: Sets the color used for lines and borders of shapes.ellipse(x, y, width, height)
: Draws an ellipse with the given parameters.rect(x, y, width, height)
: Draws a rectangle with the given parameters.line(x1, y1, x2, y2)
: Draws a line between two points.
There are many more functions available in p5.js, so don't hesitate to explore the official documentation to discover them all.
Interactivity and User Input
One of the most exciting aspects of p5.js is the ability to create interactive experiences. There are various built-in functions and variables to help you handle user input, such as mouseX
, mouseY
, mouseIsPressed
, and keyIsPressed
. These, combined with event listeners (e.g., mousePressed()
, keyPressed()
), provide a solid foundation for building interactive digital art.
Now that you have a basic understanding of p5.js, it's time to dive in and create your own interactive masterpieces. Don't worry if it feels overwhelming at first – the p5.js community is filled with helpful resources and examples to inspire and guide you on your creative journey. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Art with Code (psst, it's free!).