Drawing Ellipses in p5.js
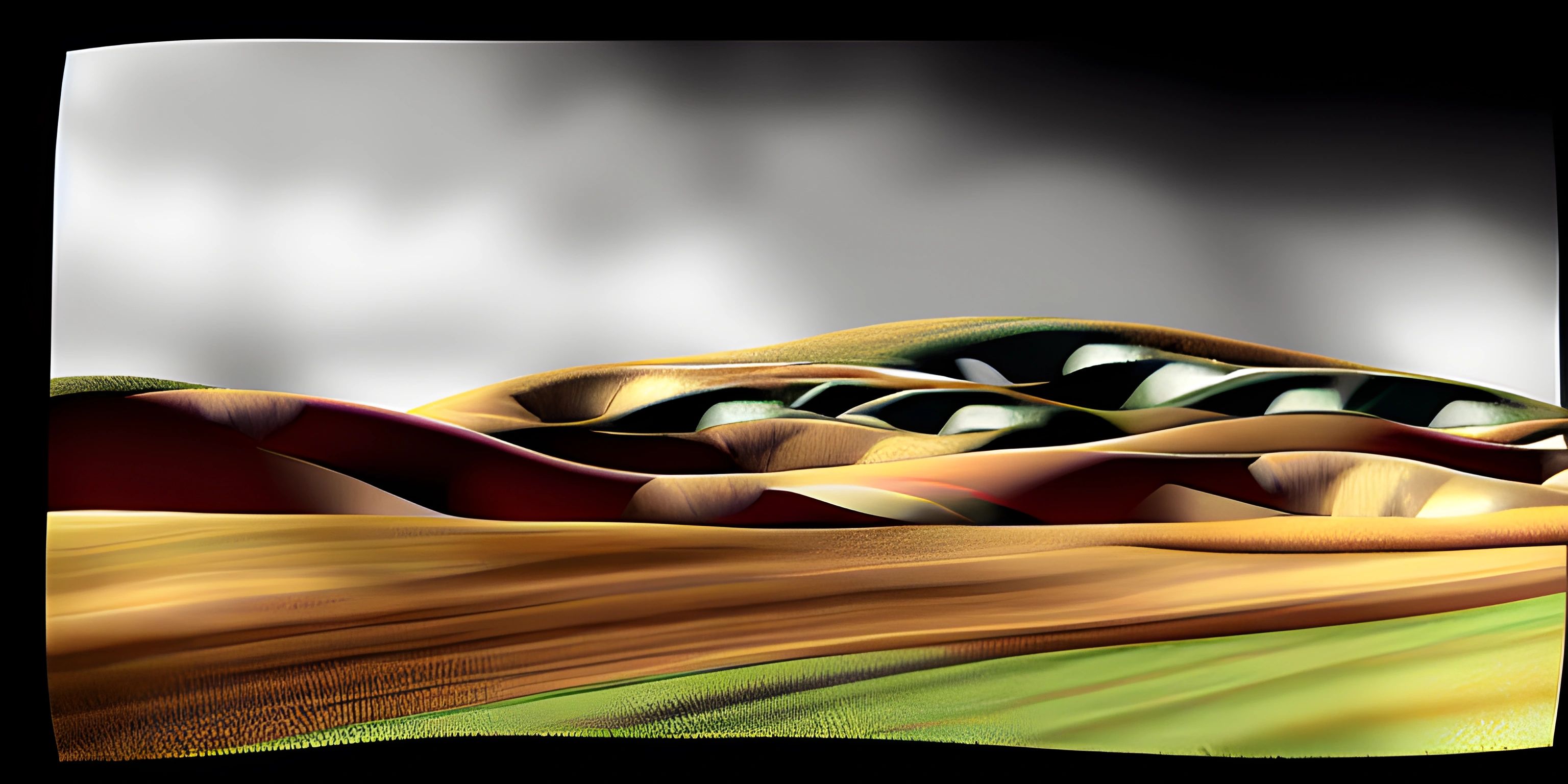
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've dipped your toes in the pool of p5.js, you'll know it's a fantastic library for creating digital art in JavaScript. Today we'll be learning about creating ellipses, and how to position and size them based on other ellipses. Grab your digital paintbrushes, we're diving in!
Creating Ellipses
In p5.js, drawing an ellipse is as simple as calling the ellipse()
function. You just need to supply the x and y coordinates of the center of the ellipse, and the width and height. If width and height are equal, you get a perfect circle! Here's a simple example:
function setup() { createCanvas(400, 400); } function draw() { background(0); ellipse(200, 200, 50, 50); // Perfect circle at the center of the canvas }
Positioning based on other Ellipses
Where the magic really happens is when you start to create ellipses based on the properties of other ellipses. Say for instance, you want to draw a smaller ellipse right next to a bigger one. You'll need to use the position and size of the first ellipse to determine where to draw the second. Let's see how that would look in code:
function setup() { createCanvas(400, 400); } function draw() { background(0); let x = 200; let y = 200; let bigEllipseSize = 100; ellipse(x, y, bigEllipseSize, bigEllipseSize); // Big ellipse ellipse(x + bigEllipseSize/2 + 20, y, 20, 20); // Small ellipse to the right of big ellipse }
In the above example, the small ellipse is drawn at a position that is half the width of the big ellipse + 20 pixels to the right of the big ellipse's center.
Sizing based on other Ellipses
Sizing an ellipse based on another ellipse takes the same kind of thinking. Let's say you want to create an ellipse that is always half the size of another. You'd use the size of the first ellipse to determine the size of the second. Here's how to do it:
function setup() { createCanvas(400, 400); } function draw() { background(0); let bigEllipseSize = 100; ellipse(200, 200, bigEllipseSize, bigEllipseSize); // Big ellipse ellipse(300, 200, bigEllipseSize/2, bigEllipseSize/2); // Small ellipse that is half the size of the big ellipse }
In this case, the small ellipse is always half the size of the big one, no matter what size the big ellipse is.
And that's it! With these techniques, you can create complex and dynamic drawings in p5.js. Remember, these examples are just the tip of the iceberg - there's a whole ocean of possibilities to explore!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
How do I draw an ellipse in p5.js?
You can draw an ellipse in p5.js by using the ellipse()
function. This function requires four parameters: the x and y coordinates for the center of the ellipse, and the width and height of the ellipse.
How do I position an ellipse based on another ellipse?
You can position an ellipse based on another ellipse by using the position and size of the first ellipse to calculate the position of the second one.
How can I size an ellipse based on another ellipse?
You can size an ellipse based on another ellipse by using the size of the first ellipse to calculate the size of the second one.
Can I draw an ellipse that is always half the size of another ellipse?
Yes, you can draw an ellipse that is always half the size of another ellipse by setting the size of the second ellipse to be half of the size of the first one when calling the ellipse()
function.
Can I create complex and dynamic drawings in p5.js?
Absolutely! With the techniques explained in this article, you can create complex and dynamic drawings in p5.js. You just need to practice and experiment with different techniques.