PEP-8 Style Guide
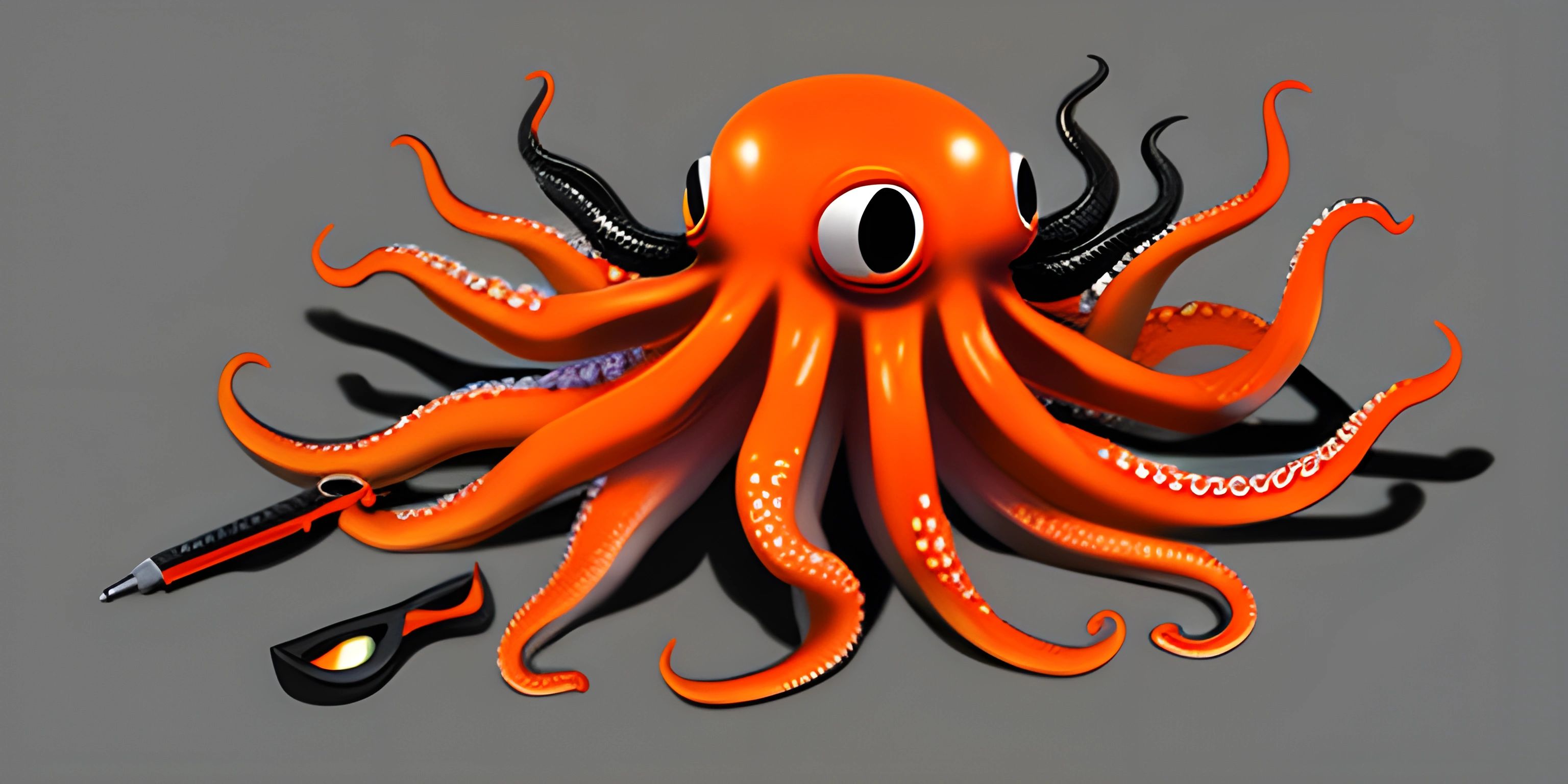
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The PEP-8 Style Guide is the official style guide for writing Python code. It's a set of rules and recommendations that help to keep your code clean, readable, and maintainable. By following these guidelines, you'll be able to write code that's easier to understand, more consistent with other Python projects, and more enjoyable for yourself and others to work with.
Importance of PEP-8
The main idea behind adhering to the PEP-8 style guide is to ensure that your code is clean and easily understood by others. This is especially important in collaborative projects, where multiple developers work on the same codebase. Consistent code style helps to reduce confusion and minimize potential errors. Plus, it's always more pleasant to read well-structured code.
Some Key PEP-8 Guidelines
Here are a few key PEP-8 guidelines to help you get started:
Indentation
Use four spaces per indentation level. No tabs! This keeps the code clean, and spaces are more consistent across different editors and platforms.
def my_function(): if some_condition: print("Four spaces for indentation")
Maximum Line Length
Limit lines to 79 characters. This makes the code more readable and reduces the need for horizontal scrolling.
# This line is within the 79 character limit short_variable = "This is a short string that easily fits within the limit."
Blank Lines
Use blank lines to separate functions and classes, and to visually group related sections of code.
def first_function(): print("Hello, world!") def second_function(): print("Goodbye, world!")
Imports
Place imports at the beginning of the file, on separate lines. Organize them by type: standard library, third-party, and local.
# Standard library imports import os import sys # Third-party imports import requests # Local imports from my_project import my_module
Naming Conventions
Use descriptive names for variables, functions, and classes, and follow the accepted naming conventions:
- Variables and functions: lowercase, words separated by underscores (e.g.,
my_variable
,my_function
). - Classes: CapWords (e.g.,
MyClass
).
class Dog: def __init__(self, name): self.name = name def bark(self): print("Woof!")
There's a lot more to PEP-8, but these guidelines should give you a good starting point for writing clean, readable Python code.
Tools for PEP-8 Compliance
There are several tools available, such as flake8 and autopep8, that can help you automatically check and enforce PEP-8 compliance in your code.
Conclusion
By following the PEP-8 style guide, you'll be creating Python code that's more consistent, easier to read, and enjoyable to work with. It's a small investment that brings significant long-term benefits to the quality of your code and the experience of working with it.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Lifetimes (psst, it's free!).
FAQ
What is the PEP-8 Style Guide?
The PEP-8 Style Guide is the official style guide for writing Python code. It sets rules and recommendations for code formatting, naming conventions, and other aspects of coding style to ensure that code is consistent, clean, and maintainable.
Why is it important to follow the PEP-8 style guide?
Following the PEP-8 style guide ensures that your code is more readable, maintainable, and consistent with other Python projects. It also makes it easier for you and others to collaborate and understand the code.
What are some key PEP-8 guidelines?
Some of the key PEP-8 guidelines include using four spaces for indentation, limiting line length to 79 characters, separating code with blank lines, organizing imports, and following naming conventions for variables, functions, and classes.
What tools can help me enforce PEP-8 compliance in my code?
Tools like flake8 and autopep8 can help you automatically check and enforce PEP-8 compliance in your code by identifying and, in some cases, automatically fixing violations.