Python: A Beginner's Guide
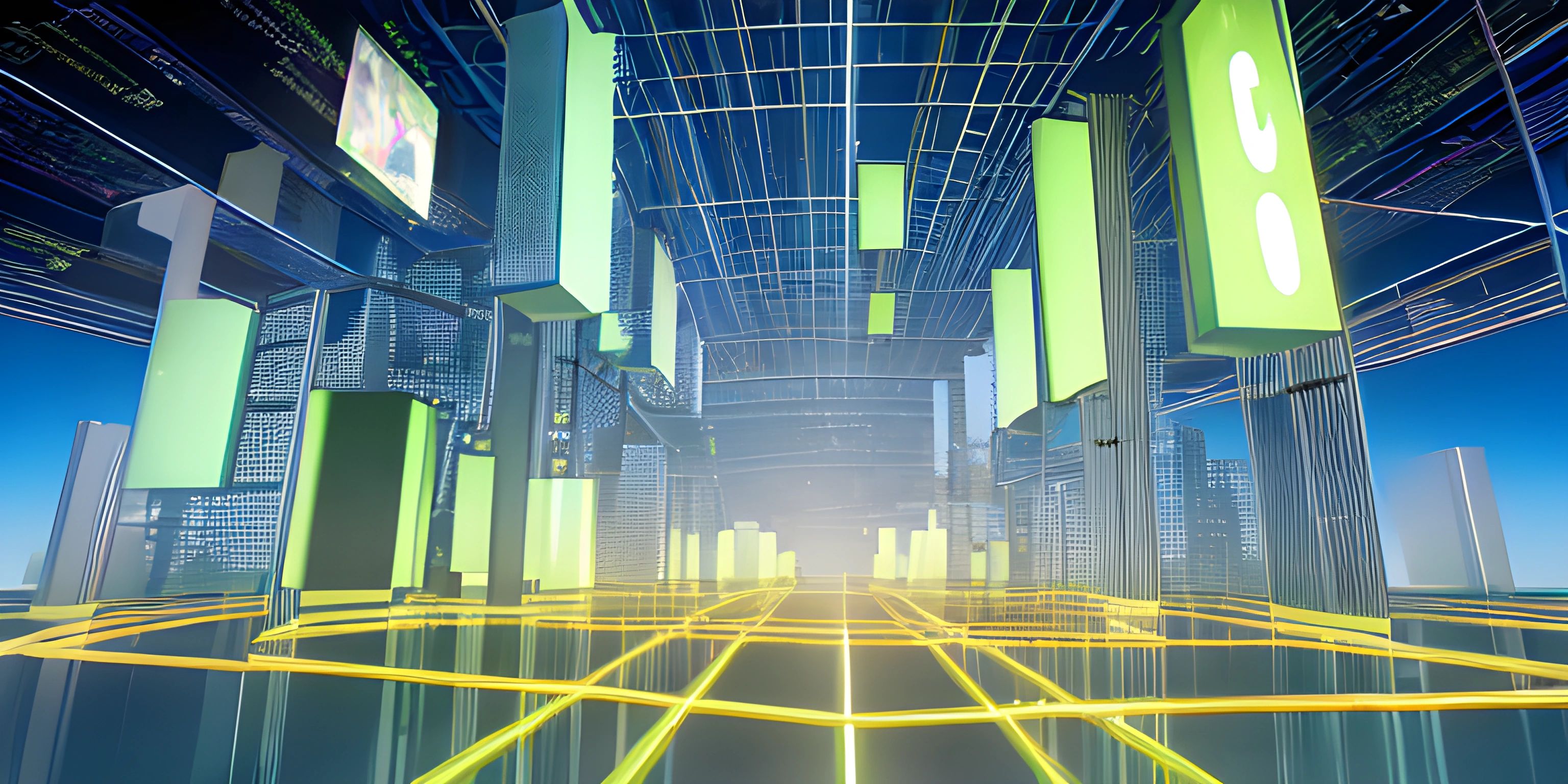
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python, a snake or a programming language? Well, for us, it's definitely the latter! Python is a popular high-level programming language known for its simplicity and readability, which makes it a great choice for beginners. So, let's dive into the wonderful world of Python coding.
Syntax
Python's syntax is one of its greatest attractions. The structure and rules of Python, or its syntax, is straightforward and easy to understand, even for beginners. Let's look at a classic "Hello World" example:
print("Hello, World!")
Just one line of code, and you've written your first Python program! The print
function puts whatever you put inside the parentheses onto the screen. In this case, it's the string "Hello, World!"
Data Types
In Python, you'll be dealing with a variety of data types. These include integers, floating point numbers, strings, and booleans. Let's look at how we can work with these different data types:
# This is an integer x = 7 # This is a floating point number y = 7.5 # This is a string z = "Hello, Python!" # This is a boolean w = True
Here, we've created four variables and assigned them different data types. Variables are containers for storing data, and we'll be using them all the time in Python.
Control Structures
Control structures help us manage the flow of our program. In Python, we have several control structures, including if
, for
, and while
statements. Here's an example of how an if
statement works:
x = 10 if x > 5: print("x is greater than 5") else: print("x is less than or equal to 5")
In this example, we're checking if the variable x
is greater than 5. If it is, we print one message; if it isn't, we print another. Control structures like these are what make our programs dynamic and interactive.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
Is Python a good language for beginners?
Yes, Python is an excellent language for beginners. Its syntax is clear and concise, it has a rich set of libraries and tools, and its community is active and supportive.
What are some of the data types in Python?
Python has several basic data types, including integers (int), floating point numbers (float), strings (str), and booleans (bool). These form the basic building blocks for storing data in Python.
What are control structures in Python?
Control structures in Python, like if
, for
, and while
statements, allow us to control the flow of our program. They allow our scripts to make decisions and repeat actions, making them dynamic and interactive.
How do I print something in Python?
In Python, you can use the print()
function to output text to the console. For example, print("Hello, World!")
will print the string "Hello, World!" to the console.
What is a variable in Python?
A variable in Python is a container for storing data values. In Python, variables are created when you assign a value to them. For example, x = 10
creates a variable named x
and assigns it the integer value 10.