Comparing Object Oriented, Functional, and Procedural Approaches
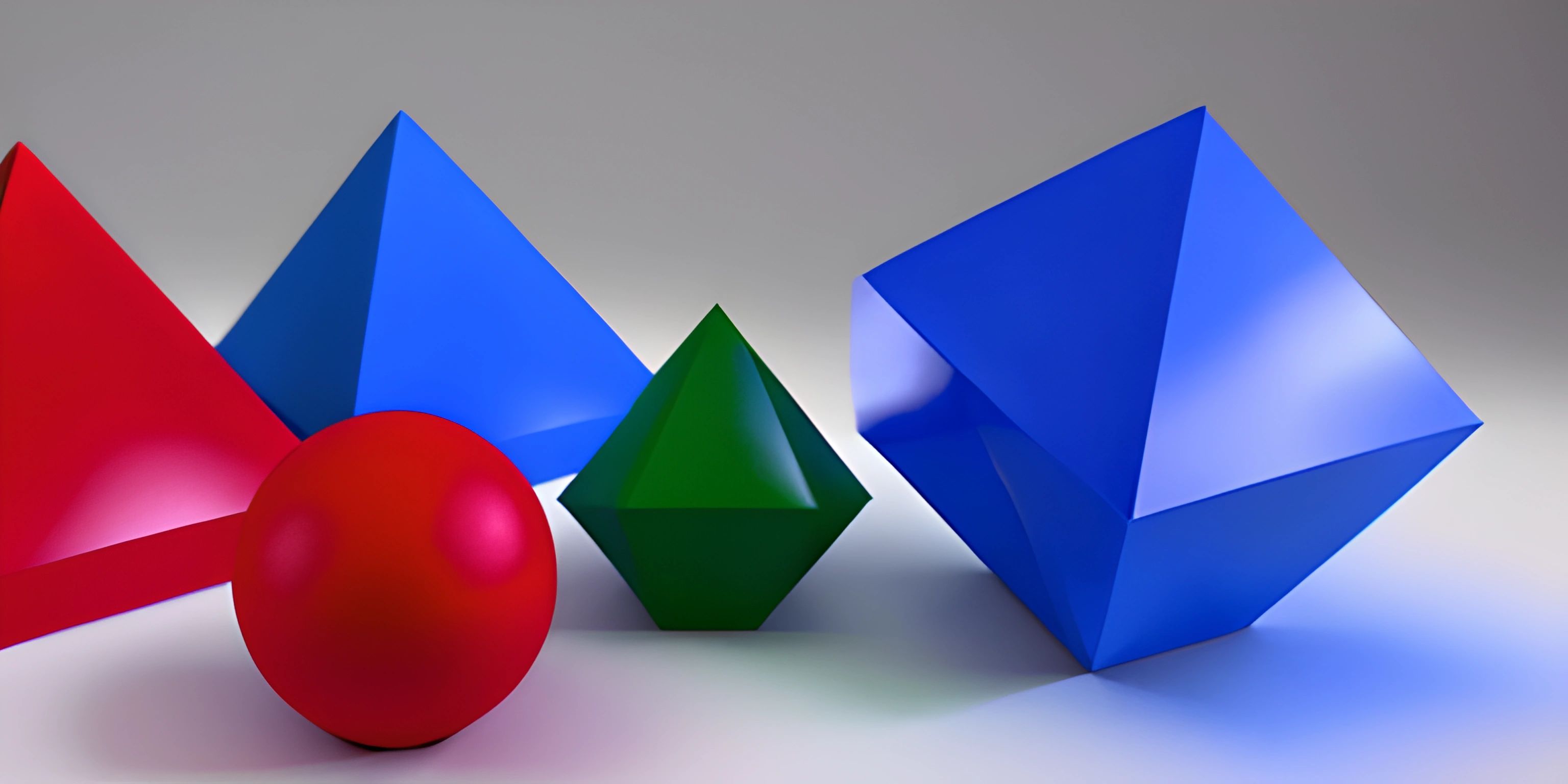
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming, there are several approaches to solving problems and structuring code. These approaches are called programming paradigms. In this article, we will compare three popular paradigms: object-oriented, functional, and procedural programming.
Object-Oriented Programming (OOP)
Object-oriented programming is a paradigm that revolves around the concept of "objects." Objects are instances of classes, which are templates that define the properties and methods of the objects. OOP focuses on encapsulation, inheritance, and polymorphism.
Pros of OOP
- Encourages code reuse through inheritance.
- Easier to model real-world systems.
- Encapsulation helps to maintain and organize code.
Cons of OOP
- Can lead to complex class hierarchies.
- Inheritance can sometimes cause tight coupling between classes.
Popular OOP languages include Java, C++, and Python.
Functional Programming (FP)
Functional programming treats computation as the evaluation of mathematical functions, and it avoids changing state and mutable data. It emphasizes immutability, higher-order functions, and function composition.
Pros of FP
- Easier to reason about and debug due to immutability.
- Encourages modular and reusable code.
- Can lead to more efficient and parallelizable code.
Cons of FP
- Can be difficult to learn for programmers used to imperative programming.
- Can be less efficient due to the creation of new objects instead of modifying existing ones.
Popular FP languages include Haskell, Lisp, and Erlang.
Procedural Programming
Procedural programming is a paradigm that focuses on procedures (also known as functions or routines) to control the flow of the program. It is a subset of imperative programming, which provides a series of steps to execute a task.
Pros of Procedural Programming
- Straightforward and easy to understand.
- Suitable for small-scale projects.
- Can be more efficient than OOP and FP in certain cases.
Cons of Procedural Programming
- Can become difficult to manage and maintain as codebase grows.
- Lacks the advantages of OOP and FP, such as inheritance and immutability.
Popular procedural languages include C, Pascal, and Fortran.
Conclusion
Each programming paradigm has its own strengths and weaknesses, and the choice of which one to use depends on the specific problem you are trying to solve and your personal preferences. It is not uncommon for modern programming languages to support multiple paradigms, allowing you to mix and match the best aspects of each. By understanding the differences between these paradigms, you can make informed decisions about how to structure your code and improve your programming skills.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are the key differences between object-oriented, functional, and procedural programming paradigms?
Each programming paradigm has its unique characteristics:
- Object-oriented programming (OOP) focuses on grouping related data and behavior into classes and objects, promoting code reusability and modularity. It uses concepts like inheritance, encapsulation, and polymorphism.
- Functional programming (FP) emphasizes immutability, statelessness, and the use of functions as first-class citizens. It promotes writing code with fewer side-effects, making it easier to reason about and test.
- Procedural programming is a step-by-step approach to problem-solving, organizing code into procedures or subroutines. It's generally easier to learn and understand but can lead to less modular and maintainable code compared to OOP and FP.
Can I combine different programming paradigms in a single project?
Yes, it's possible to combine different paradigms in a single project. Many modern programming languages support multiple paradigms, allowing developers to leverage the strengths of each approach when appropriate. This is known as multi-paradigm programming.
Which programming paradigm is best for my project?
The "best" programming paradigm depends on your project's requirements, your team's expertise, and the language you're using. Each paradigm has its strengths and weaknesses:
- OOP is well-suited for complex, large-scale applications with many interacting components.
- FP is ideal for applications with a strong focus on concurrency, parallelism, or when working with large datasets.
- Procedural programming is a good choice for smaller projects or when simplicity and ease of understanding are essential.
How do I choose a programming language based on the desired paradigm?
When choosing a programming language, consider its support for the programming paradigm(s) you want to use. For example:
- For OOP, popular choices include Java, C++, and Python.
- For FP, languages like Haskell, Lisp, and Scala are commonly used.
- For procedural programming, you might consider C, Pascal, or Fortran.
Are there any real-world examples of projects using different programming paradigms?
Yes, many real-world projects utilize different programming paradigms to solve various problems. For example:
- Most web applications use a combination of OOP (for back-end server logic) and functional programming (for front-end JavaScript code).
- Game engines often use a mix of OOP (for game objects and their interactions) and procedural programming (for rendering or physics calculations).
- Data analysis pipelines may rely on functional programming for data transformation, combined with procedural or OOP for the overall structure and execution.