Java Polymorphism and Interfaces
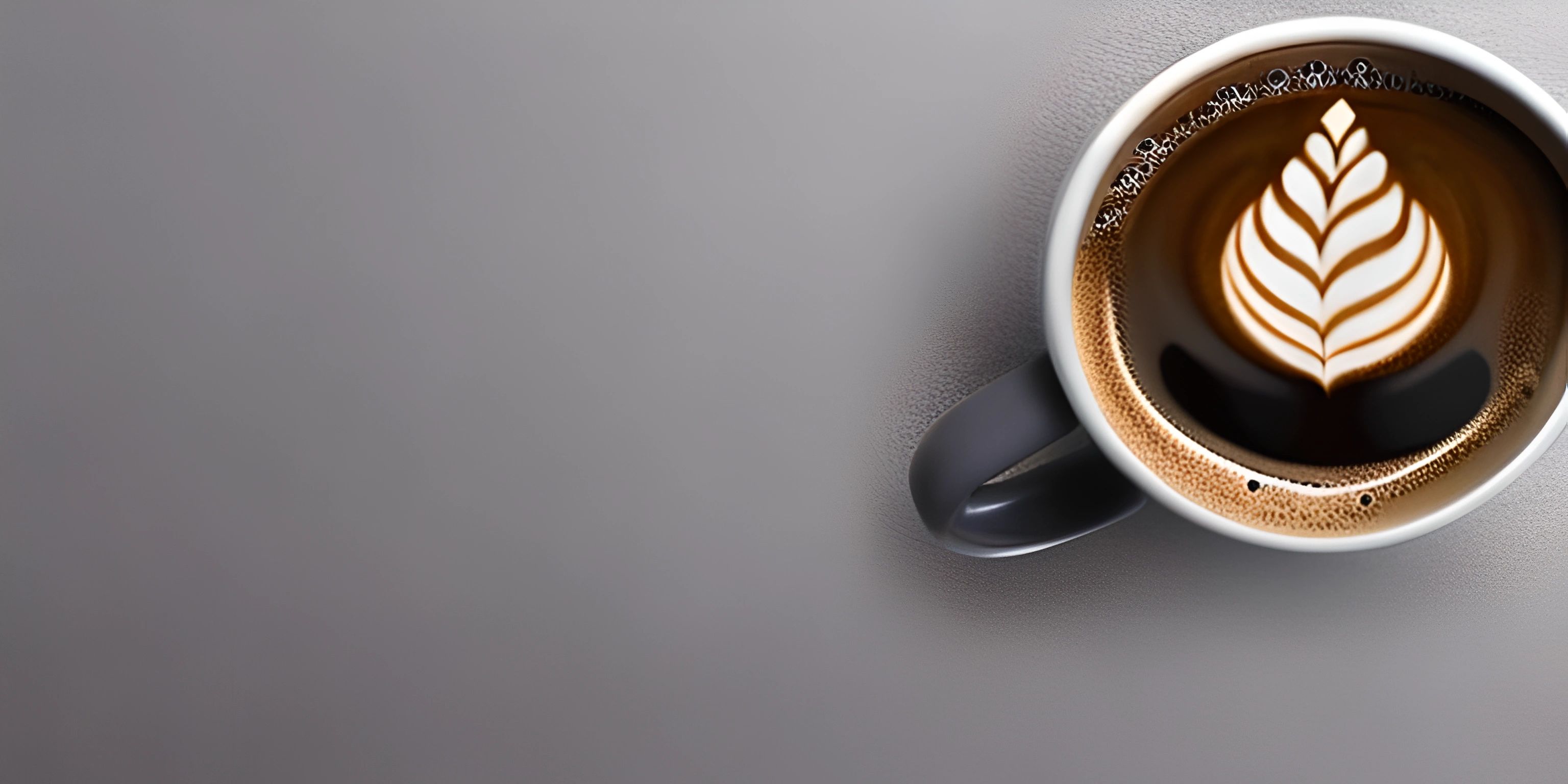
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Polymorphism is a powerful concept in object-oriented programming that allows us to effectively handle multiple data types or objects through a single interface. In Java, one way to achieve polymorphism is by using interfaces. Let's explore how interfaces work and how they can help us achieve polymorphism in our code.
Interfaces in Java
In Java, an interface is a collection of abstract methods (methods without a body). It represents a contract that any implementing class must fulfill. A class can implement an interface by using the implements
keyword, followed by the interface name.
Here's a simple example of an interface:
public interface Movable { void move(); }
And this is how a class implements the interface:
public class Car implements Movable { public void move() { System.out.println("The car is moving."); } }
Polymorphism through Interfaces
Polymorphism allows us to treat different objects as if they were of the same type. By using interfaces, we can create a single reference variable of the interface type, which can hold objects of any class that implements the interface. This way, we can call the methods declared in the interface without knowing the actual class of the object.
Let's see an example of polymorphism in action:
public class Main { public static void main(String[] args) { Movable car = new Car(); Movable bicycle = new Bicycle(); moveVehicle(car); moveVehicle(bicycle); } public static void moveVehicle(Movable movable) { movable.move(); } } public class Bicycle implements Movable { public void move() { System.out.println("The bicycle is moving."); } }
In the example above, we have two classes (Car
and Bicycle
) that implement the Movable
interface. In our main
method, we create instances of these classes and treat them as Movable
objects. This enables us to pass them to the moveVehicle
method, which calls the move
method on the passed object without knowing its actual class.
Benefits of Polymorphism with Interfaces
Using polymorphism through interfaces offers numerous advantages:
- Flexibility: We can easily add or modify classes that implement the interface, without changing the code that uses the interface.
- Reusability: We can reuse the same code with different object types, promoting code reuse and reducing duplication.
- Abstraction: By using interfaces, we can hide the implementation details of classes and focus on their behavior, making our code more comprehensible.
By leveraging polymorphism and interfaces in Java, we can create more flexible, reusable, and maintainable code. So, next time you're designing a Java application, don't forget to consider the power of interfaces and polymorphism!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is polymorphism in Java and why is it important?
Polymorphism is one of the four fundamental principles of object-oriented programming (OOP) and allows objects of different classes to be treated as objects of a common superclass. In Java, polymorphism is achieved through inheritance, interfaces, and method overriding. It promotes code reusability, makes the code more flexible, and allows for easier maintenance.
How do interfaces in Java help achieve polymorphism?
Interfaces in Java provide a way to achieve polymorphism by defining a set of abstract methods that any class implementing the interface must provide. This allows objects of different classes, which implement the same interface, to be treated as objects of a common type. When a method is called on an object of the common type, the appropriate implementation of the method is invoked based on the actual object's class.
Can you provide an example of polymorphism using interface in Java?
Sure! Let's consider an interface Drawable
that has an abstract method draw()
:
public interface Drawable { void draw(); }
Now, we'll create two classes Circle
and Square
that implement the Drawable
interface:
public class Circle implements Drawable { @Override public void draw() { System.out.println("Drawing a circle."); } } public class Square implements Drawable { @Override public void draw() { System.out.println("Drawing a square."); } }
Now, we can create an array of Drawable
objects and call the draw()
method on each element, showcasing polymorphism:
public class Main { public static void main(String[] args) { Drawable[] drawables = new Drawable[2]; drawables[0] = new Circle(); drawables[1] = new Square(); for (Drawable drawable : drawables) { drawable.draw(); } } }
Can a class implement multiple interfaces in Java?
Yes, a class in Java can implement multiple interfaces by separating the interface names with a comma. This feature facilitates the concept of multiple inheritance in Java, which allows a class to inherit behaviors from more than one parent.
public interface InterfaceA { void methodA(); } public interface InterfaceB { void methodB(); } public class MyClass implements InterfaceA, InterfaceB { @Override public void methodA() { System.out.println("Method A implementation"); } @Override public void methodB() { System.out.println("Method B implementation"); } }
Can an interface extend another interface in Java?
Yes, an interface in Java can extend one or more other interfaces using the extends
keyword. This allows the extending (child) interface to inherit the abstract methods from the parent interface(s), and any class implementing the child interface must provide implementations for all inherited methods.
public interface Shape { void draw(); } public interface ColoredShape extends Shape { void setColor(String color); } public class Circle implements ColoredShape { @Override public void draw() { System.out.println("Drawing a circle."); } @Override public void setColor(String color) { System.out.println("Setting circle color to " + color + "."); } }