Programming Paradigms
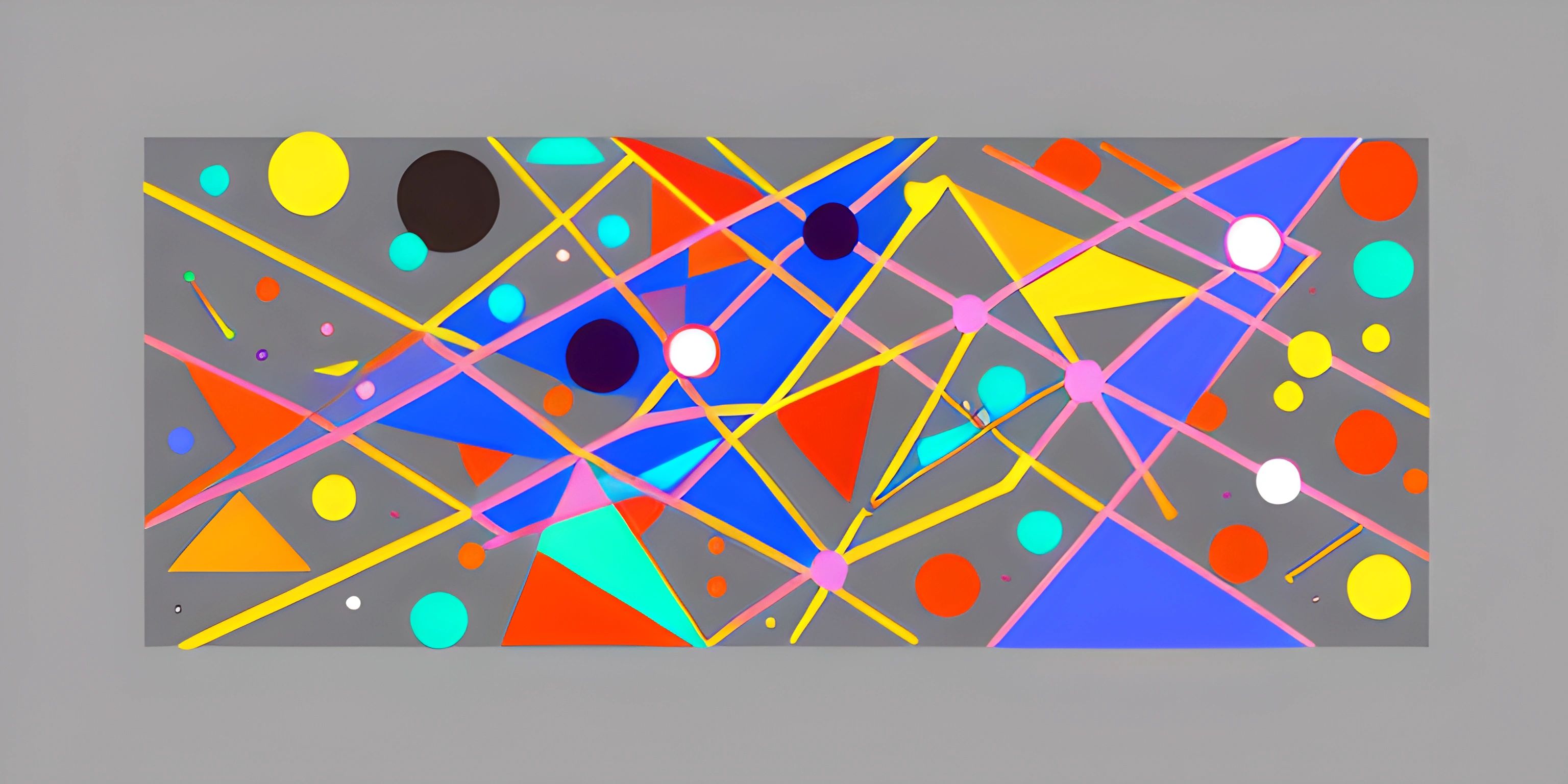
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dive into the world of programming paradigms, where different approaches to solving problems reign supreme. We'll explore the core concepts of some common paradigms, the languages that embody them, and what makes each unique.
Imperative Programming
Imperative programming focuses on how to solve a problem by providing step-by-step instructions. It's the most intuitive approach for many newcomers, as it closely mimics how we think about solving problems in everyday life.
Imagine baking a cake: you follow a recipe with clear instructions, like "mix eggs and sugar" or "bake for 30 minutes." That's imperative programming in a nutshell.
In this paradigm, you'll often find loops, conditionals, and variables. Languages that support imperative programming include C, C++, and Java.
Declarative Programming
Declarative programming shifts the focus from how to solve a problem to what the desired outcome is. Instead of writing explicit steps, you define the end result, and let the language or framework figure out the best way to achieve it.
Picture a GPS: you enter your destination, and it calculates the route for you. You don't need to tell it every turn to make, it just gets you there. That's declarative programming in action.
Functional Programming
Functional programming is a subset of declarative programming that treats computation as the evaluation of mathematical functions. It avoids changing the state or mutable data, thus promoting immutability, predictability, and easier debugging.
In functional programming, you'll encounter higher-order functions, pure functions, and recursion. Languages that support functional programming include Haskell, Lisp, and Erlang.
Logic Programming
Logic programming is another subset of declarative programming, where you express the relationships between different entities using formal logic. The program then searches for a solution that satisfies these constraints.
Imagine solving a Sudoku puzzle: you don't need a specific algorithm, you just need to satisfy the rules of the game. That's logic programming at work.
Languages that support logic programming include Prolog and Mercury.
Object-Oriented Programming
Object-oriented programming (OOP) organizes code around objects that represent real-world entities, making it easier to model complex systems. Objects have attributes (data) and methods (functions) that operate on those attributes.
Think of objects as blueprints for creating instances of a class. For example, a "Car" class might have attributes like "make" and "model" and methods like "drive" and "park".
OOP emphasizes encapsulation, inheritance, and polymorphism. Languages that support OOP include Python, Java, and C#.
Conclusion
Programming paradigms offer a way to approach problem-solving from different perspectives. Each paradigm has its strengths and weaknesses, and the best choice often depends on the problem at hand. By being familiar with multiple paradigms, you'll become a more versatile programmer, ready to tackle any challenge that comes your way.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are the main programming paradigms?
The main programming paradigms are imperative, declarative, object-oriented, and functional programming. Each paradigm is based on a unique approach to organizing and structuring code, and solving problems.
How does imperative programming work?
Imperative programming is a paradigm that focuses on describing the steps a program must take to achieve its goal. It uses statements to change a program's state, which is typically represented by variables. Examples of imperative languages include C, C++, and Java.
#include <stdio.h> int main() { int a = 5; int b = 10; int sum = a + b; printf("The sum is: %d\n", sum); return 0; }
What is declarative programming and how is it different from imperative programming?
Declarative programming is a paradigm that emphasizes describing the desired outcome of a program, without explicitly detailing the steps for achieving it. It contrasts with imperative programming, which focuses on step-by-step instructions. Declarative languages include SQL, Prolog, and Haskell.
SELECT first_name, last_name FROM employees WHERE salary > 50000;
Can you explain object-oriented programming and its key principles?
Object-oriented programming (OOP) is a paradigm that uses objects, which are instances of classes, to represent and manipulate data. The key principles of OOP are encapsulation, inheritance, and polymorphism. Encapsulation involves bundling data and methods within a class. Inheritance allows classes to inherit attributes and methods from parent classes, while polymorphism enables a single function to operate on different types of objects. Examples of OOP languages include Python, Java, and C#.
class Vehicle: def __init__(self, make, model): self.make = make self.model = model def honk(self): print("Honk!") class Car(Vehicle): def honk(self): print("Beep!") my_car = Car("Toyota", "Camry") my_car.honk()
What are the main features of functional programming?
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions, focusing on immutability and avoiding mutable state and side effects. It encourages the use of higher-order functions, recursion, and pure functions. Examples of functional languages include Haskell, Lisp, and Scala.
factorial :: Integer -> Integer factorial 0 = 1 factorial n = n * factorial (n - 1) main = print (factorial 5)