Java Interfaces
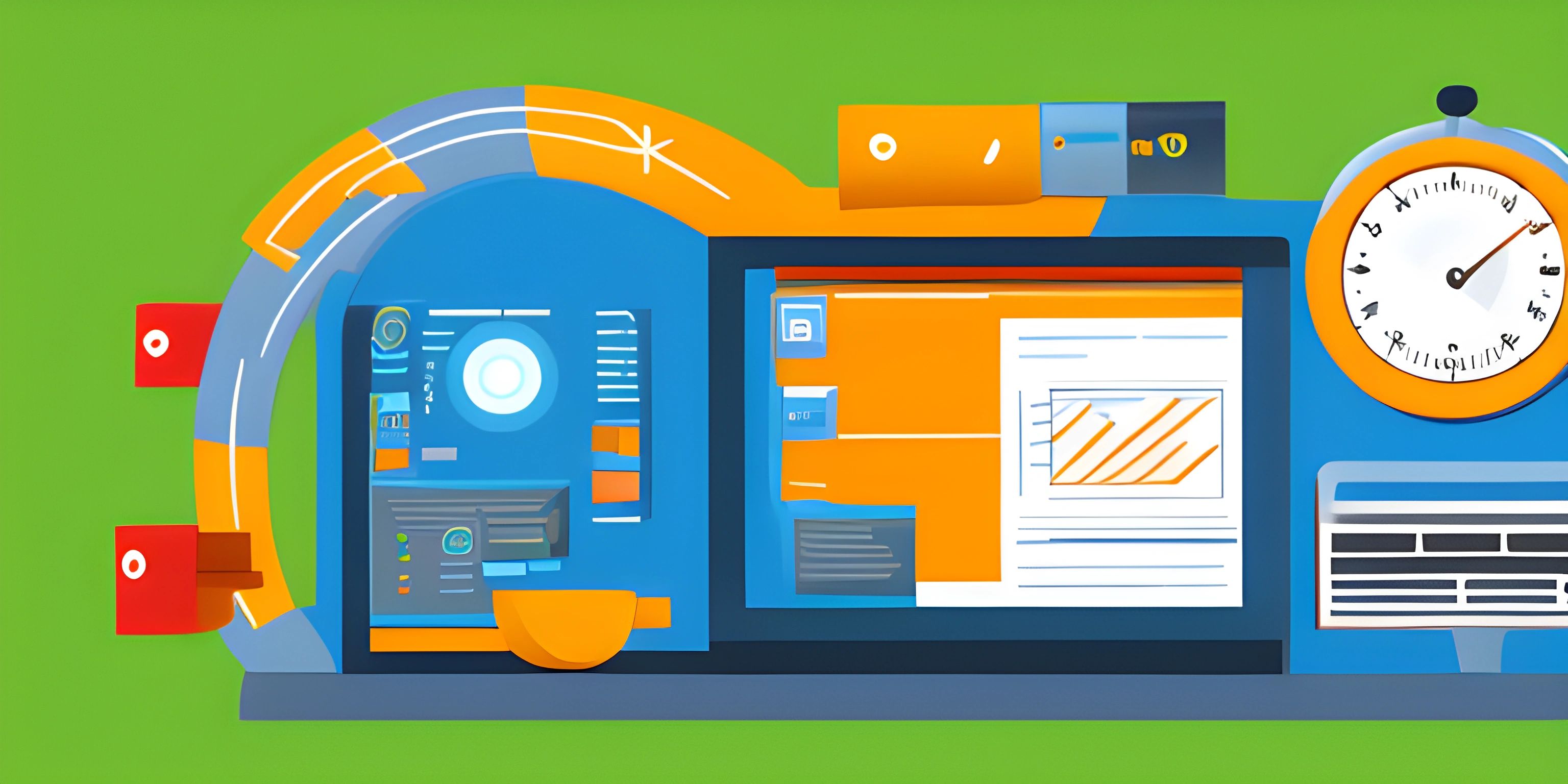
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever wondered how your TV remote communicates with your TV? Or how your smartphone pairs with your car's Bluetooth system? This is made possible through the magic of interfaces. In programming, interfaces are just as magical and versatile.
Java Interfaces
Let's dive straight into the world of Java interfaces. In Java, an interface is a blueprint of a class. It has static constants and abstract methods. Java Interface also represents the IS-A relationship.
In layman's terms, an interface is a contract for what a class should do. It's like a job description that details what tasks an employee should perform, but not how they should be done.
For instance, a "Flyable" interface might look like this:
public interface Flyable { void fly(); }
Any class implementing this Flyable
interface would be required to have a fly
method.
public class Bird implements Flyable { public void fly() { System.out.println("The bird is flying."); } }
In this example, the Bird
class is promising to adhere to the Flyable
interface's contract by defining a fly
method.
Now, the magic starts when we realize that any class, not just Bird
, can implement Flyable
.
public class Airplane implements Flyable { public void fly() { System.out.println("The airplane is flying."); } }
So, both Bird
and Airplane
are Flyable
, but each implements flying in their own way.
Why is this useful? Well, imagine you have a list of Flyable
objects. You don't care if they're birds, airplanes, or Superman – you just want them all to fly. With interfaces, you can do just that!
List<Flyable> flyingObjects = Arrays.asList(new Bird(), new Airplane()); for (Flyable object : flyingObjects) { object.fly(); }
And voila! You've just made a bird and an airplane fly without knowing or caring what they were. You just knew they were Flyable
. That's the power of interfaces!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is an interface in Java?
An interface in Java is a blueprint of a class. It can have static constants and abstract methods. It represents an IS-A relationship and acts as a contract, specifying what methods a class should implement.
Why are interfaces useful?
Interfaces are useful because they define a contract for what a class should do, allowing you to use objects without knowing their exact type. You just need to know that they fulfill the contract of the interface. This is helpful for things like allowing different types of objects to be used interchangeably.
How do I create an interface in Java?
An interface in Java is created using the interface
keyword followed by the name of the interface and a set of method signatures contained within curly braces {}
. These methods are abstract, meaning they do not have a body and must be implemented by any class that uses the interface.
Can a class implement multiple interfaces?
Yes, a class in Java can implement multiple interfaces. This is one of the ways Java circumvents the issue of not having multiple inheritance. The class has to provide implementations for all the methods in all the interfaces it implements.
Can an interface extend another interface?
Yes, just like a class, an interface can extend another interface in Java. This allows you to create a hierarchy of interfaces where a child interface inherits the methods of its parent interface. The child interface can also add its own methods.