Understanding Pure Functions
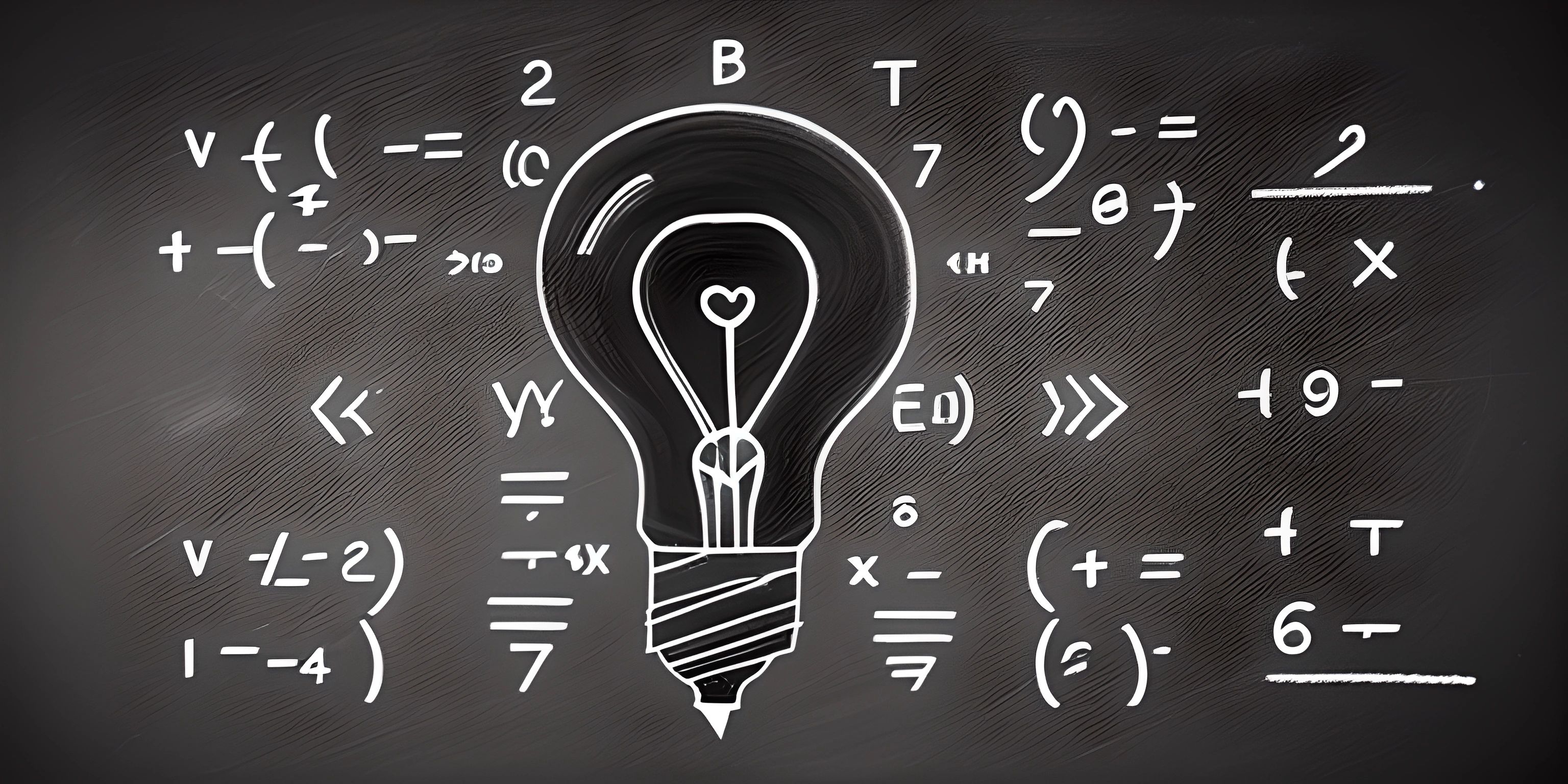
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you're diving into the world of functional programming, you'll inevitably come across the concept of pure functions. These programming gems are known for their clean and maintainable nature, making them a vital part of functional programming. So, what exactly makes a function pure, and why should we care about them? Let's find out!
What is a Pure Function?
Pure functions are a fundamental concept in functional programming. A function is considered pure if it meets two specific criteria:
- Deterministic: Given the same input, a pure function always returns the same output.
- No Side Effects: A pure function does not modify any external state or produce any side effects.
This might sound abstract, so let's break it down with an example. Let's say we have a function that calculates the sum of two numbers:
def add(a, b): return a + b
This function is pure because it always produces the same output for the same input and doesn't have any side effects. In other words, it doesn't change anything outside of the function or cause any unintended consequences.
Now, let's look at an impure function:
counter = 0 def increment_counter(): global counter counter += 1 return counter
This function is impure because it has a side effect – it modifies the global variable counter
. The output of this function depends on the current value of counter
, making it non-deterministic.
The Advantages of Pure Functions
Pure functions offer several benefits that make them appealing to use in your code:
Predictability
Pure functions are predictable because their output solely depends on their input. This makes it easier to reason about your code, test it, and debug it.
Reusability and Modularity
Pure functions promote code reusability and modularity. Because they don't rely on external state or produce side effects, you can easily combine pure functions to create more complex functionality without worrying about unintended consequences.
Concurrency and Parallelism
Pure functions can be executed in parallel without any issues, as they don't access or modify shared state. This makes them particularly useful in concurrent or parallel programming scenarios, where managing shared state can be challenging.
Pure Functions in Practice
Now that we understand the concept of pure functions, let's look at how to apply them in practice. Here's an example using JavaScript:
// A pure function to calculate the price of an item with tax function calculatePrice(itemPrice, taxRate) { return itemPrice * (1 + taxRate); } // Usage const itemPrice = 100; const taxRate = 0.07; const finalPrice = calculatePrice(itemPrice, taxRate); console.log(`The final price is: ${finalPrice}`);
In this example, the calculatePrice
function is pure because it meets both criteria: it's deterministic and has no side effects. By using a pure function, we've made our code more predictable, maintainable, and easier to test.
Conclusion
Pure functions are a powerful concept in functional programming that can help you write clean, maintainable, and reusable code. By understanding their properties and incorporating them into your programming practices, you'll be well on your way to writing more efficient and robust code. So, the next time you're hacking away at your codebase, keep an eye out for opportunities to make your functions pure and reap the benefits!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is a pure function?
A pure function is a function that has two main characteristics:
- It always produces the same output for the same input.
- It doesn't have any side effects, meaning it doesn't modify any external state or data. Pure functions are crucial in functional programming, as they promote clean, maintainable, and predictable code.
Can you provide an example of a pure function?
Sure! Here's a simple example of a pure function in JavaScript:
function add(a, b) { return a + b; }
This function is pure because it always produces the same output for the same input (e.g., add(2, 3)
will always return 5
), and it doesn't have any side effects.
Why are pure functions important in functional programming?
Pure functions are important in functional programming because they make the code more predictable, easier to test, and easier to maintain. Since pure functions don't have side effects, you don't need to worry about them affecting other parts of your application. Moreover, their consistent output makes it simpler to reason about the code and spot potential bugs.
Can you give an example of an impure function and how to make it pure?
Sure! Here's an example of an impure function that adds two numbers and increments a global variable:
let counter = 0; function impureAdd(a, b) { counter++; return a + b; }
To make this function pure, we can remove the side effect (i.e., incrementing the global variable counter
). Here's the updated, pure version:
function pureAdd(a, b) { return a + b; }
Are there any downsides to using pure functions?
While pure functions have many benefits, they may not always be practical or efficient in certain situations. For example, when dealing with input/output operations, database interactions, or other side effects, it's challenging to maintain purity. Additionally, using pure functions can sometimes lead to performance overhead, as you may need to create new data structures instead of modifying existing ones in place. However, the benefits of easier testing, maintainability, and predictability generally outweigh these downsides.