The Power of Functions in Programming
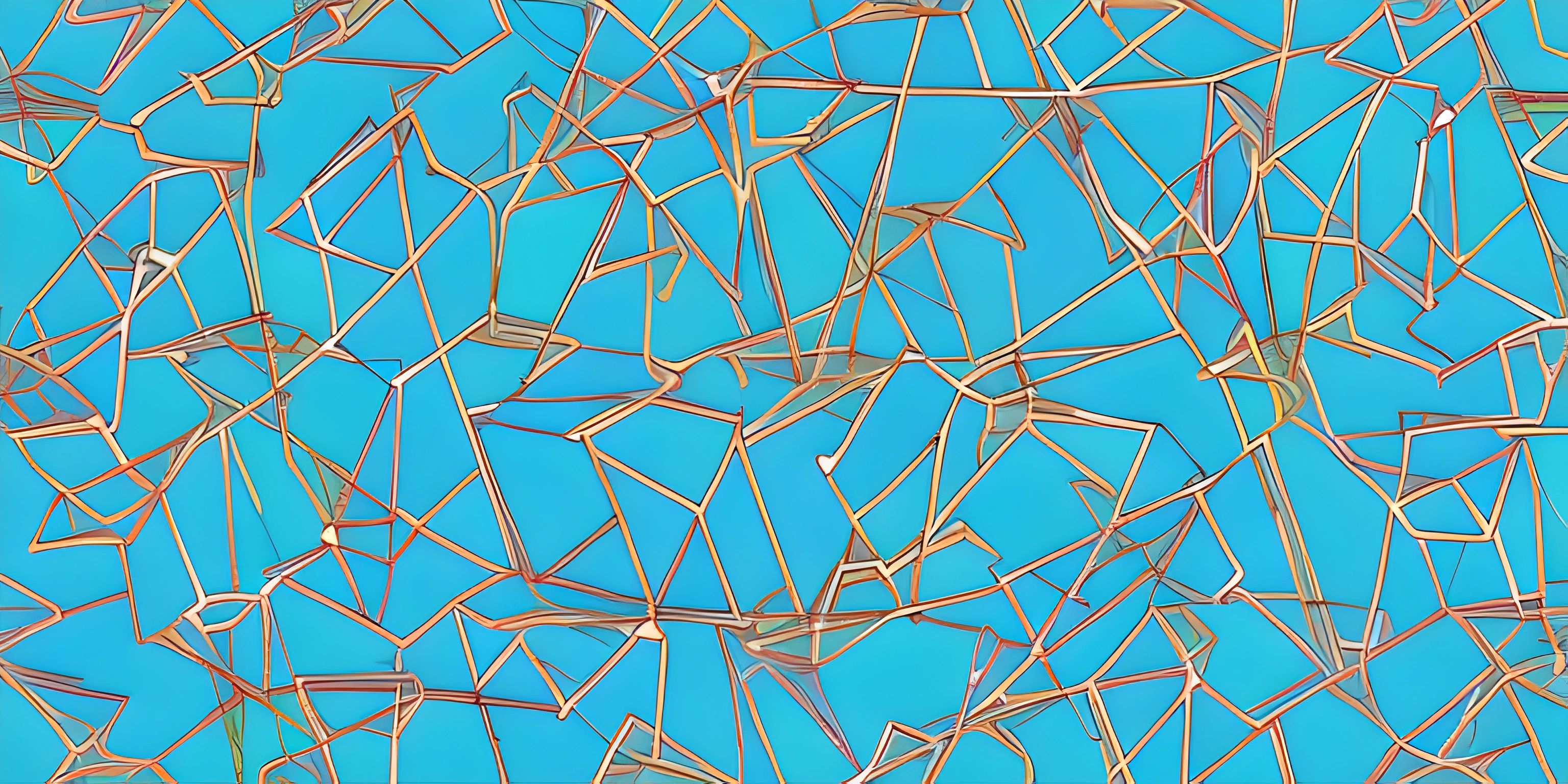
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Functions are like the Swiss Army knives of programming, versatile and essential. They are the building blocks that make your code more organized, efficient, and reusable. Functions help to break down complex tasks into smaller, manageable parts, making your code easier to understand and maintain.
What are Functions?
In programming, a function is a group of statements that perform a specific task. Functions take input, process it, and return an output. Just like a well-trained dog, you give a function a command (and maybe a treat), and it will do its magic and return the result.
Why Functions are Important
Imagine you're trying to build a house without any tools. You might be able to do it, but it'll take a lot of time and effort. Functions are the tools that help you construct your code more quickly and efficiently. Here's why:
-
Organization: Functions help you organize your code into logical sections, making it easier to understand and maintain. They allow you to separate concerns and focus on one task at a time.
-
Reusability: Functions can be called multiple times with different inputs, reducing the need for duplicate code. This makes your code shorter, cleaner, and more efficient.
-
Abstraction: Functions hide complexity by encapsulating code, allowing you to focus on the higher-level logic. This promotes better overall design and helps to reduce errors.
-
Modularity: Functions can be easily combined and reused in different parts of your code, as well as in different projects. This creates modular code that's flexible and easy to expand.
Functions in Action
Let's take a look at a simple example using Python:
def greet(name): return f"Hello, {name}!" print(greet("Alice")) print(greet("Bob"))
Here, we define a function called greet
that takes one parameter, name
. The function returns a greeting string that includes the given name. We can now use this function to greet different people by calling it with different arguments.
Functions as Building Blocks
Now, let's say we want to build a more complex program that sends personalized emails to a list of recipients. We can use our greet
function as a building block:
def send_email(email, subject, body): # Code to send the email pass recipients = [ {"name": "Alice", "email": "[email protected]"}, {"name": "Bob", "email": "[email protected]"}, ] for recipient in recipients: name = recipient["name"] email = recipient["email"] greeting = greet(name) email_body = f"{greeting}\n\nWe have a special offer for you!" send_email(email, "Special Offer", email_body)
Our program now consists of multiple functions, each responsible for a specific task. The greet
function generates a greeting, and the send_email
function sends an email. By combining these functions, we can create a more complex and organized program.
Conclusion
Functions are a powerful tool in programming, allowing you to create organized, reusable, and modular code. They help you break down complex tasks into smaller, more manageable parts, making your code more efficient and easier to maintain. So, the next time you write code, remember to harness the power of functions and unlock their full potential!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using Constants (psst, it's free!).