Python for Beginners
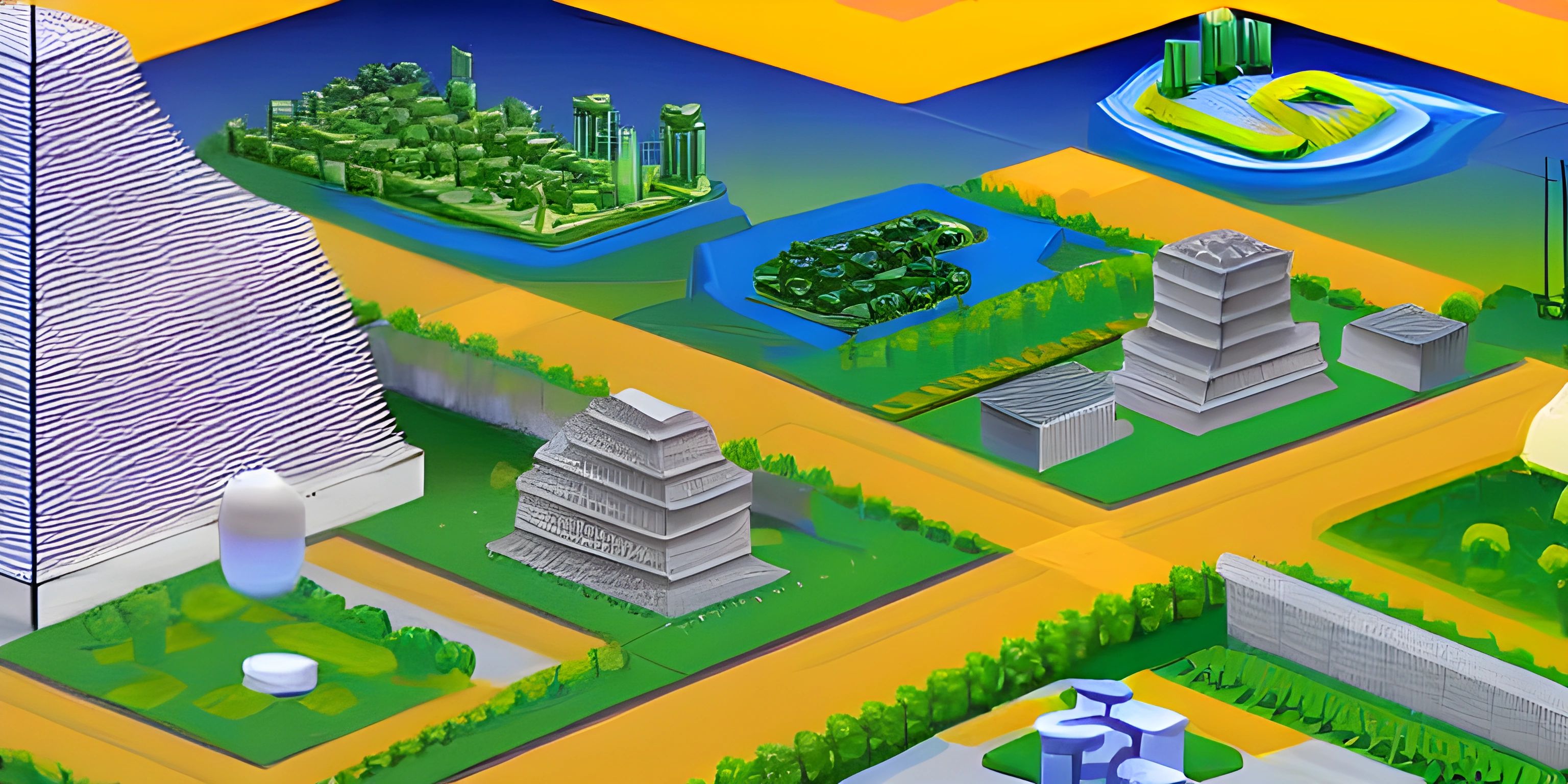
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a versatile and beginner-friendly programming language that is widely used for various applications, ranging from simple scripts to complex web applications and machine learning models. In this article, we'll introduce Python and its basic concepts, including variables, data types, control structures, and functions.
Getting Started with Python
To start programming in Python, you need to have it installed on your computer. You can download the latest version of Python from the official website. Once installed, you can access Python through the command line (terminal) or by using an Integrated Development Environment (IDE) like Visual Studio Code or PyCharm.
Hello, World!
The classic "Hello, World!" program is the simplest way to start learning a new programming language. In Python, this can be achieved in just one line:
print("Hello, World!")
This code will print the text "Hello, World!" to the console. The print()
function is a built-in Python function used for displaying output.
Variables and Data Types
In Python, variables are used to store data. You don't need to declare the data type of a variable explicitly, as Python automatically infers the type. Here are some common data types and examples of how to use them:
# Integer age = 25 # Float (floating-point number) height = 180.5 # String name = "John Doe" # Boolean is_student = True
You can also perform operations with variables, such as addition, subtraction, multiplication, and division.
result = age + 5
Control Structures
Control structures are used to control the flow of your program. They include conditional statements (if-else) and loops (for, while).
If-else
The if-else statement is used to make decisions based on conditions. Here's an example:
age = 18 if age >= 18: print("You are an adult.") else: print("You are not an adult.")
Loops
Loops are used to execute a block of code repeatedly. Python supports two types of loops: for
and while
.
For
The for
loop is commonly used to iterate over a sequence (e.g., a list, tuple, or string).
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
While
The while
loop repeats a block of code as long as a certain condition remains true.
count = 0 while count < 5: print(count) count += 1
Functions
Functions are reusable pieces of code that can be called with arguments and may return a value. Functions are defined using the def
keyword, followed by the function name and a pair of parentheses.
def greet(name): return "Hello, " + name greeting = greet("John") print(greeting)
In this example, we've defined a function called greet
that takes a single argument name
and returns a greeting string.
Now you know the basics of Python programming! Keep on exploring and learning more about this powerful and versatile language.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is Python?
Python is a versatile, high-level, and beginner-friendly programming language used for various applications, including web development, scripting, data analysis, and machine learning.
How do I install Python?
You can download the latest version of Python from the official website (https://www.python.org/downloads/) and follow the installation instructions for your operating system.
What are some common data types in Python?
Some common data types in Python include integers, floating-point numbers, strings, and booleans.
What are loops in Python?
Loops in Python are used to execute a block of code repeatedly. Python supports two types of loops: for
and while
.
How do I create a function in Python?
In Python, functions are created using the def
keyword, followed by the function name and a pair of parentheses containing the function's arguments.