Python Basics
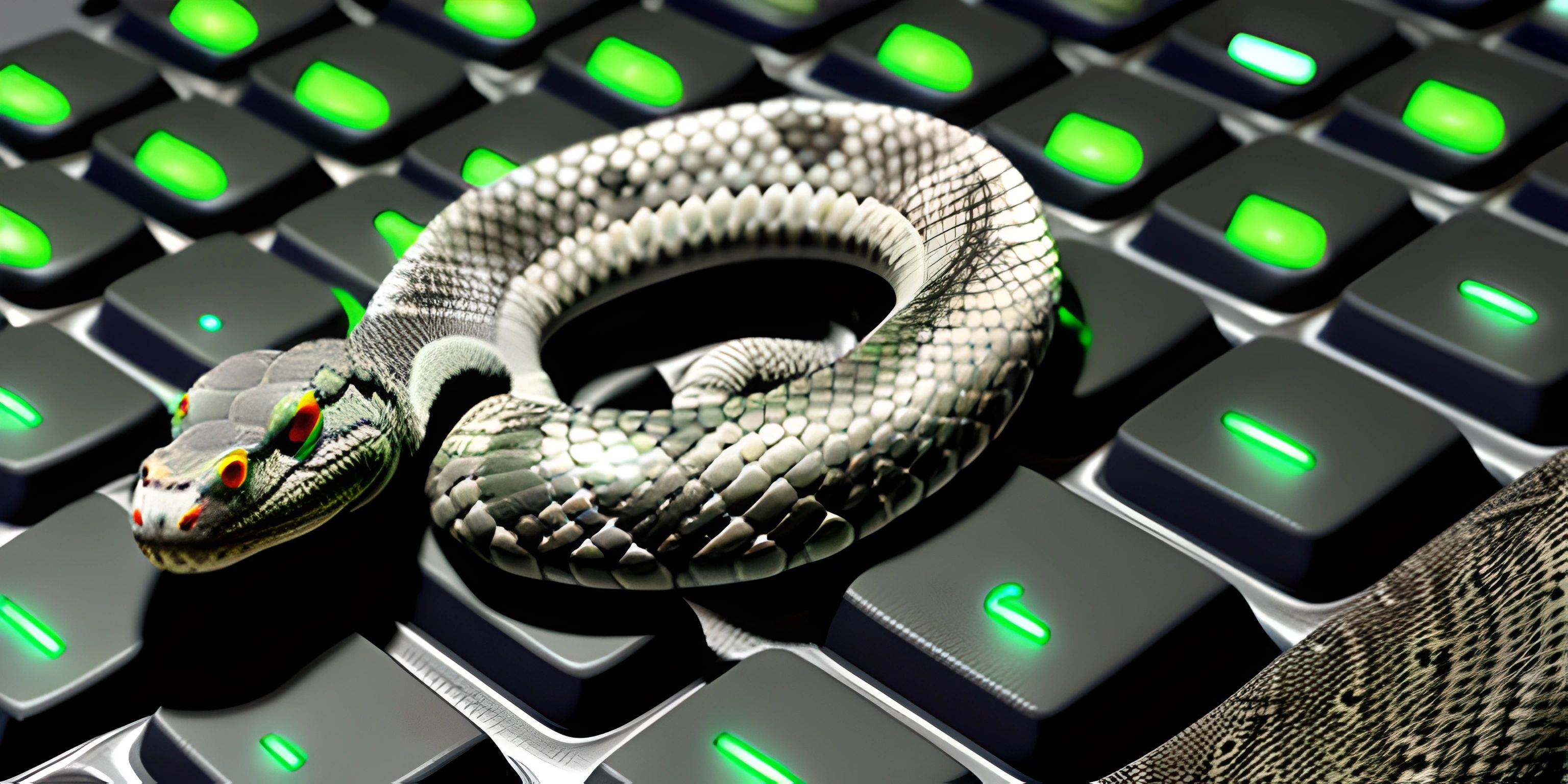
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python, the lovable programming language that doesn't bite, is known for its readability and versatility. Whether you're just starting your coding journey or you're a seasoned programmer looking to add another language to your arsenal, Python is a fantastic choice. Let's slither through the basics of Python together and learn some fundamental concepts.
Python Syntax
Python's syntax is designed to be clean and straightforward. This means that you can focus on building your awesome projects instead of getting tangled in curly braces or semicolons. To give you an idea of how Python looks, here's a simple "Hello, World!" program:
print("Hello, World!")
That's all you need! Run this code, and you'll be greeted with a friendly "Hello, World!" message.
Indentation
Python uses indentation to define blocks of code, unlike languages like C or JavaScript that use curly braces. The standard indentation is four spaces, and you should stick with that to maintain readability. Let's take a look at an example with a simple if
statement:
if 5 > 2: print("Five is greater than two!")
Notice how the print()
function is indented after the if
statement. This indentation shows that it's part of the code block that should execute when the condition is true.
Variables
In Python, you can create variables to store data. You don't need to specify the type of data a variable can hold, as Python is a dynamically-typed language. Let's create a variable called greeting
and assign it the value "Hello, Python!":
greeting = "Hello, Python!" print(greeting)
Running this code will print "Hello, Python!" to the console, showing that the variable greeting
has been assigned the string value.
Basic Data Types
Python offers a variety of data types to store your data. Some of the basic ones include:
- Integers (e.g.,
42
) - Floats (e.g.,
3.14
) - Strings (e.g.,
"Hello, World!"
) - Booleans (e.g.,
True
orFalse
)
Here's an example demonstrating different data types:
integer_example = 7 float_example = 2.5 string_example = "Python is fun!" boolean_example = True print(integer_example, float_example, string_example, boolean_example)
This code will print 7 2.5 Python is fun! True
, showing the values of the variables holding different data types.
Functions
Functions are reusable blocks of code that can be called multiple times with different arguments. You can define a function using the def
keyword, followed by the function name and a pair of parentheses. Let's create a simple function that adds two numbers and returns the result:
def add_numbers(num1, num2): result = num1 + num2 return result sum_result = add_numbers(3, 5) print(sum_result)
This code defines a function called add_numbers
that takes two arguments, num1
and num2
. It adds them together and returns the result. When called with the arguments 3
and 5
, it returns 8
.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is Python and why is it so popular?
Python is a high-level, versatile programming language known for its ease of readability and simplicity. It is widely used for web development, data analysis, artificial intelligence, machine learning, and more. Python's popularity can be attributed to its clean syntax, a vast selection of libraries, and a large, supportive community.
How do I install Python on my computer?
To install Python, follow these steps:
- Visit the official Python website at https://www.python.org/downloads/
- Download the installer for your specific operating system (Windows, macOS, or Linux)
- Run the installer and follow the on-screen instructions. Make sure to check the option "Add Python to PATH" during installation.
How do I write and run a simple Python program?
To write your first Python program, follow these steps:
- Open a text editor or an Integrated Development Environment (IDE) like Visual Studio Code or PyCharm.
- Create a new file and save it with a
.py
extension, likehello_world.py
. - Write your code inside the file. For example, write the classic "Hello, World!" program:
print("Hello, World!")
- Save the file.
- Open a terminal or command prompt, navigate to the directory where your file is saved, and run the following command:
python hello_world.py
This will execute your program and display the output "Hello, World!" in the terminal.
What are some fundamental concepts and syntax in Python?
Some fundamental concepts and syntax in Python include:
- Variables: Store values in memory. Example:
my_variable = 42
- Data types: Basic data types include integers, floats, strings, and booleans. Example:
my_string = "Hello"
- Lists: Ordered, mutable collections of items. Example:
my_list = [1, 2, 3, 4, 5]
- Tuples: Ordered, immutable collections of items. Example:
my_tuple = (1, 2, 3)
- Dictionaries: Key-value pairs for efficient data storage. Example:
my_dict = {"key": "value"}
- Control structures:
if
,else
, andelif
for conditional execution;for
andwhile
loops for iteration. - Functions: Blocks of reusable code. Example:
def greet(name): print("Hello, " + name + "!")
- Modules and libraries: Import and use external code. Example:
import math