Getting Started with Python Programming
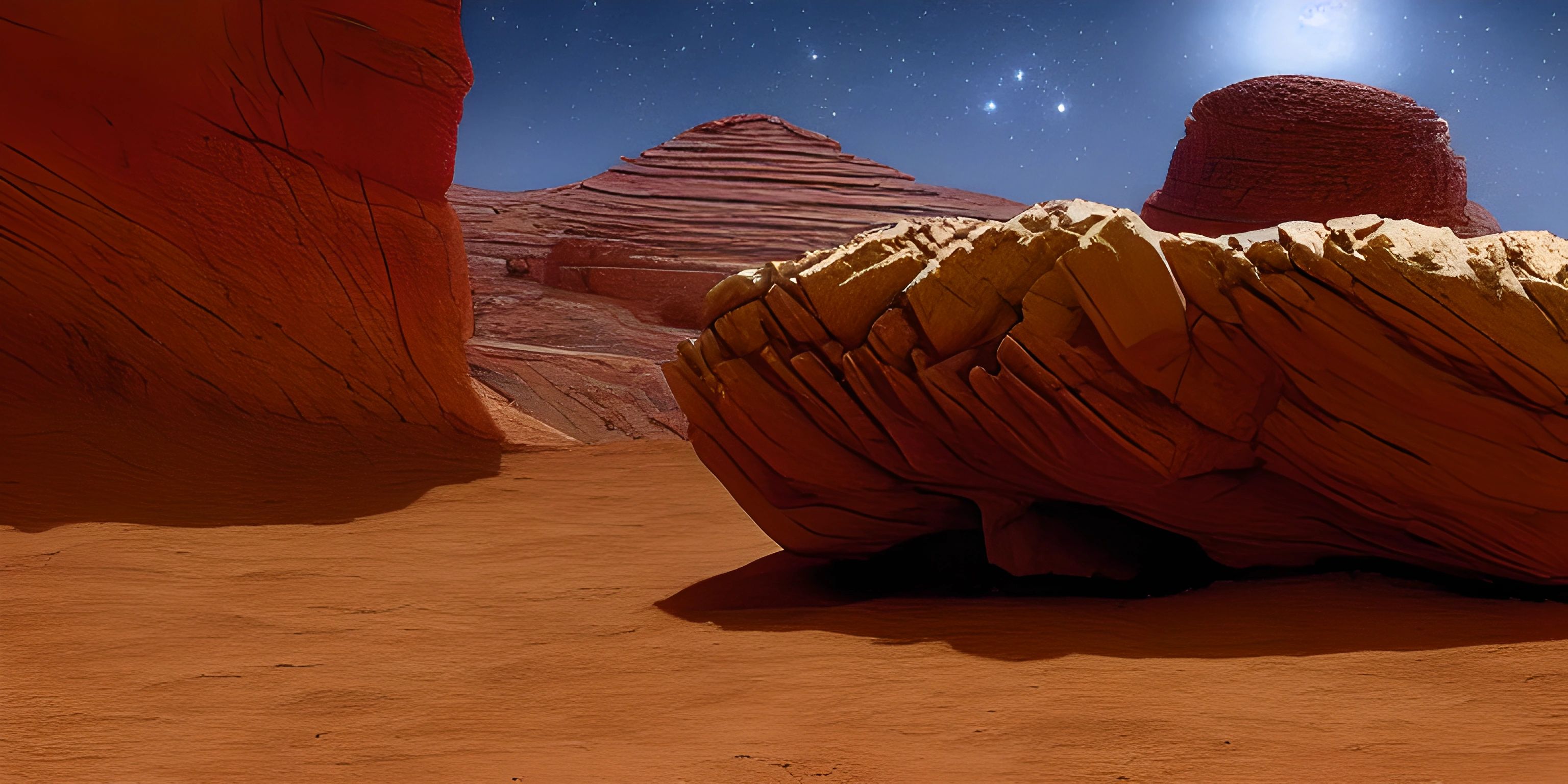
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a versatile programming language known for its simplicity and readability. It's widely used for various tasks, such as web development, data analysis, and artificial intelligence. With its beginner-friendly nature and a huge community, Python is a fantastic choice for programmers of all levels. Let's dive into the world of Python and learn the basics!
Installing Python
To begin, you'll need to install Python on your computer. Head over to the official Python website and download the latest version for your operating system. Follow the installation instructions, and you'll be ready to write your first Python program in no time!
Python Syntax
Python's syntax is designed to be clean and easy to understand. It uses indentation to define blocks of code, so you won't find curly braces {}
or semicolons ;
like in some other languages. Here's a simple example:
if 5 > 3: print("Five is greater than three.")
Notice that the print
statement is indented, indicating that it belongs to the if
block.
Writing Your First Python Program
Now that you have Python installed, it's time to write your first program. Open your favorite text editor or integrated development environment (IDE), and let's start with the classic "Hello, World!" example:
print("Hello, World!")
Save your file with a .py
extension, such as hello_world.py
. Now, open your terminal or command prompt, navigate to the directory where you saved the file, and run the following command:
python hello_world.py
You should see the message "Hello, World!" printed on the screen. Congratulations, you've just written and executed your first Python program!
Variables and Data Types
In Python, you can store values in variables, which are like containers for your data. You don't need to declare variable types explicitly, as Python automatically infers the type based on the value you assign. Here's an example:
name = "Alice" age = 30 is_student = True
In this example, we created three variables: name
is a string, age
is an integer, and is_student
is a boolean.
Basic Control Structures
Control structures in Python allow you to control the flow of your program. The most common ones are if
, elif
, and else
for conditional statements and for
and while
loops for iteration.
Conditional Statements
Here's an example using if
, elif
, and else
:
x = 42 if x < 0: print("x is negative") elif x == 0: print("x is zero") else: print("x is positive")
Loops
Python provides two loop structures: for
and while
. The for
loop is used to iterate over a sequence (such as a list or a string), and the while
loop repeats a block of code as long as a given condition is true.
Here's an example of a for
loop:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
And here's a while
loop example:
count = 1 while count <= 5: print(count) count += 1
Functions
Functions in Python are blocks of reusable code that perform a specific task. You can define your own functions using the def
keyword, followed by the function name and a pair of parentheses. Here's an example of a simple function that adds two numbers:
def add_numbers(a, b): return a + b result = add_numbers(3, 5) print(result) # This will print "8"
Now that you've learned the basics of Python programming, it's time to explore more advanced topics and start building your own projects. Python has a rich ecosystem of libraries and frameworks, so the possibilities are limitless. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
What is Python and why is it a popular programming language?
Python is a high-level, interpreted, and general-purpose programming language known for its simplicity, readability, and versatility. It is popular because it allows developers to write code faster and with fewer errors compared to other languages.
How do I install Python on my computer?
To install Python, visit the official Python website (python.org) and download the appropriate installer for your operating system. Follow the installation prompts and ensure that you add Python to your PATH during the installation process.
How do I write and run my first Python program?
To write a Python program, open a text editor or an Integrated Development Environment (IDE) like Visual Studio Code or PyCharm, and create a new file with a .py
extension. Write your code in the file, save it, and then run the program using a command prompt or terminal by typing python yourfilename.py
.
What are some basic Python programming concepts I should know?
Some fundamental Python programming concepts include variables, data types, conditional statements, loops, functions, and error handling. Familiarity with these concepts will help you write efficient and effective Python programs.
Where can I find resources to further my Python learning?
There are numerous resources available for learning Python, such as online tutorials, documentation, and video courses. A great starting point is the official Python documentation (docs.python.org) and websites like Real Python, Python.org, and Codecademy.