Java Loops
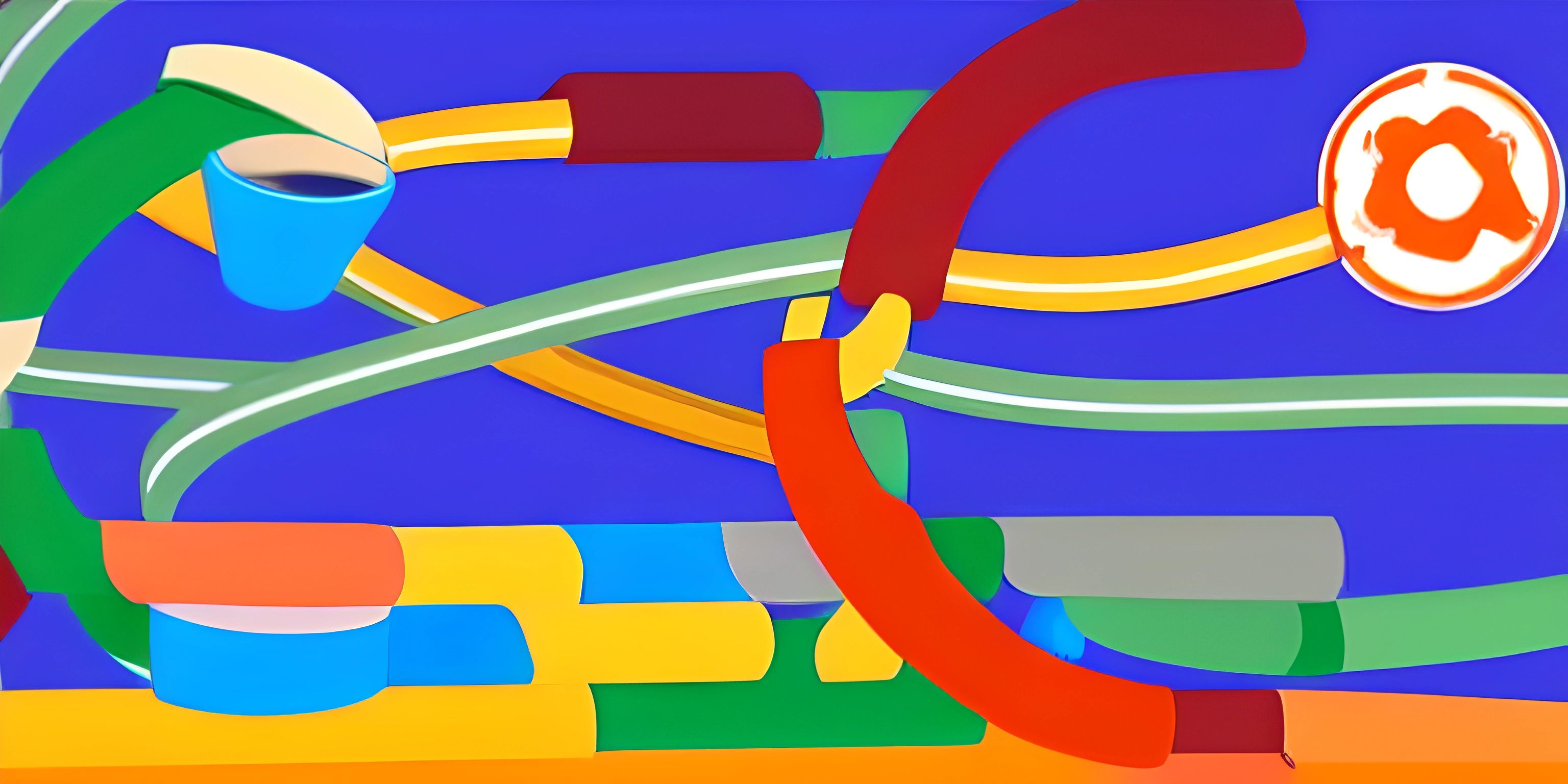
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to repeating tasks in Java, loops are your best friend. They allow you to execute a block of code multiple times, which can save you from writing repetitive code and make your program more efficient. In Java, there are three main types of loops: for loops, while loops, and do-while loops. Let's dive into each one and see how they work.
For Loops
For loops are the most common type of loop in Java. They are great for situations where you know how many times you want to repeat a task. The basic syntax of a for loop is as follows:
for (initialization; condition; increment) { // Code to be executed }
- Initialization: This is where you set the starting value of a loop control variable, which is usually an integer.
- Condition: This is a boolean expression that will be checked before each iteration of the loop. If the condition is true, the loop will continue; if it's false, the loop will break.
- Increment: This is where you update the loop control variable after each iteration.
Here's an example of a for loop that prints the numbers 1 to 5:
for (int i = 1; i <= 5; i++) { System.out.println(i); }
While Loops
While loops are useful when you don't know how many times you need to repeat a task, but you know the condition that must be met for the loop to continue. The basic syntax of a while loop is:
while (condition) { // Code to be executed }
The loop will continue to execute as long as the condition remains true. It's important to ensure that the condition will eventually become false, otherwise, you'll end up with an infinite loop. Here's an example of a while loop that prints the numbers 1 to 5:
int i = 1; while (i <= 5) { System.out.println(i); i++; }
Do-While Loops
Do-while loops are similar to while loops, but they guarantee that the loop will execute at least once, even if the condition is false from the beginning. The basic syntax of a do-while loop is:
do { // Code to be executed } while (condition);
Like with while loops, it's important to ensure that the condition will eventually become false to avoid infinite loops. Here's an example of a do-while loop that prints the numbers 1 to 5:
int i = 1; do { System.out.println(i); i++; } while (i <= 5);
Choosing the Right Loop
Now that you know the different types of loops in Java, you might wonder which one to use in a given situation. Here's a simple guideline:
- Use for loops when you know the exact number of times you want to repeat a task.
- Use while loops when you don't know how many times you'll repeat the task, but you know the condition that must be met for the loop to continue.
- Use do-while loops when you want to guarantee that the loop will execute at least once, regardless of the initial condition.
With a solid understanding of loops in Java, you're well on your way to writing more efficient and dynamic code. Just remember to always keep an eye out for infinite loops and ensure that your conditions will eventually become false. Happy looping!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the different types of loops available in Java?
Java provides three main types of loops:
- For loop: This loop is used when you know the number of iterations in advance. It follows the format
for (initialization; condition; increment/decrement) { // statements }
. - While loop: This loop is used when you don't know the number of iterations in advance, but have a condition to check. It follows the format
while (condition) { // statements }
. - Do-while loop: This loop is similar to the while loop, but it executes the statements at least once before checking the condition. It follows the format
do { // statements } while (condition);
.
How do I create a simple for loop in Java?
To create a simple for loop in Java, follow the below format:
for (int i = 0; i < 10; i++) { System.out.println("Iteration: " + i); }
This loop will iterate 10 times, printing the value of i
at each iteration.
How do I create a while loop in Java?
To create a while loop in Java, follow the below format:
int counter = 0; while (counter < 10) { System.out.println("Counter value: " + counter); counter++; }
This loop will iterate until the counter
variable reaches 10, printing the value of counter
at each iteration.
How do I create a do-while loop in Java?
To create a do-while loop in Java, follow the below format:
int counter = 0; do { System.out.println("Counter value: " + counter); counter++; } while (counter < 10);
This loop will execute at least once, even if the condition is false from the beginning, and will repeat until the counter
variable reaches 10, printing the value of counter
at each iteration.