Semantic Analysis with Python and NLTK
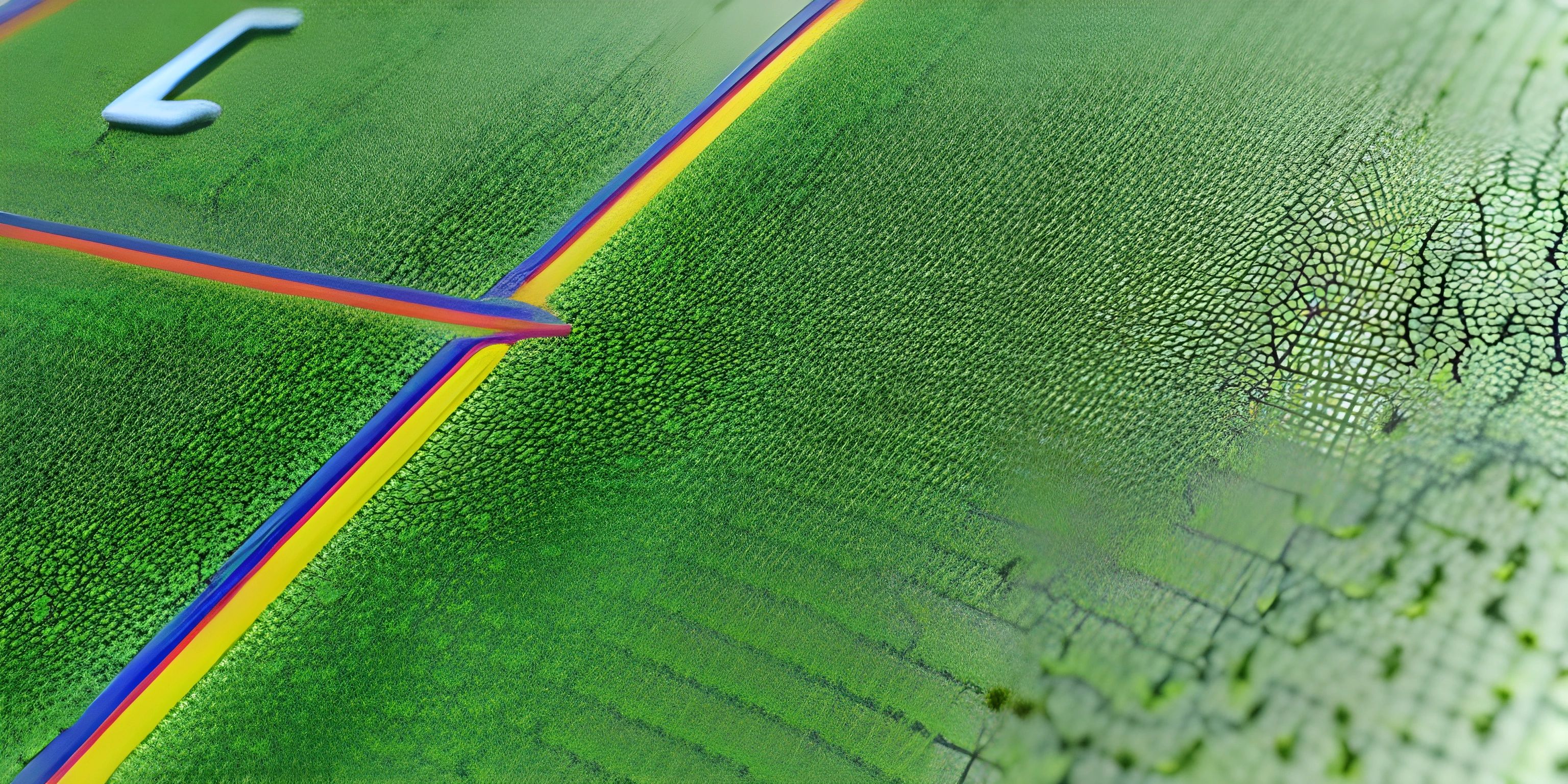
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Semantic analysis is like the Sherlock Holmes of text mining. It dives deep into text, picks up the subtle meanings, and comes back with some profound insights that even Watson (no, not the AI) would marvel at. Today, we're going to do some detective work ourselves with Python and the Natural Language Toolkit (NLTK).
Getting Started
Python and NLTK are a power duo, like Batman and Robin, perfect for semantic analysis. So we start off by installing NLTK. On your Python environment, run the following command:
pip install nltk
Once NLTK is installed, we're ready to get into the nitty-gritty.
What is Semantic Analysis?
Semantic analysis or Semantic Mining is the process of extracting meaning from text. It's like understanding the language of dolphins, except the dolphins are articles, blogs, comments, reviews, etc.
The NLTK Library
NLTK, short for Natural Language Toolkit, is like a Swiss Army knife for language processing in Python. It comes with a bunch of features like tokenization, part-of-speech tagging, and semantic reasoning wrappers. We're going to focus on semantic analysis using NLTK today.
Semantic Analysis with NLTK
Let's start by tokenizing our text. Tokenization is the process of breaking down text into tokens (words, sentences, etc.). It's like chopping up a block of cheese into bite-sized pieces. Here's how we do it:
import nltk nltk.download('punkt') text = "NLTK is a leading platform for building Python programs to work with human language data." tokens = nltk.word_tokenize(text) print(tokens)
But words alone don't convey the full meaning. We need to understand the role of each word, which is where part-of-speech tagging comes in. It's like assigning roles in a school play. Here's how to do it:
nltk.download('averaged_perceptron_tagger') tagged = nltk.pos_tag(tokens) print(tagged)
Now comes the real Sherlock Holmes stuff - semantic reasoning. This is where we decipher the meaning behind the text. We can do this by using WordNet, a large lexical database of English. In WordNet, nouns, verbs, adjectives, and adverbs are grouped into sets of cognitive synonyms (synsets), each expressing a distinct concept.
nltk.download('wordnet') from nltk.corpus import wordnet synonyms = [] for syn in wordnet.synsets("program"): for lemma in syn.lemmas(): synonyms.append(lemma.name()) print(synonyms)
This will print out a list of synonyms for the word "program", showcasing the power of semantic analysis through NLTK.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is semantic analysis?
Semantic analysis is the process of extracting meaning from text. It's like understanding the language of dolphins, except the dolphins are articles, blogs, comments, reviews, etc.
What is NLTK and what can it do?
NLTK, short for Natural Language Toolkit, is a Python library used for language processing. It comes with a bunch of features like tokenization, part-of-speech tagging, and semantic reasoning wrappers, making it a versatile tool for text mining and data analysis.
How do I perform semantic analysis using NLTK?
You would first perform tokenization and part-of-speech tagging using NLTK's built-in methods. For semantic reasoning, you can use WordNet, a large lexical database of English integrated with NLTK. This lets you find synonyms, antonyms, and more, giving you a deep insight into the text.
What is tokenization?
Tokenization is the process of breaking down text into tokens (words, sentences, etc.). It's like chopping up a block of cheese into bite-sized pieces.
What is part-of-speech tagging?
Part-of-speech tagging is the process of assigning grammatical roles (like noun, verb, adjective, etc.) to each token in the text. It's like assigning roles in a school play.