Fibonacci Sequence
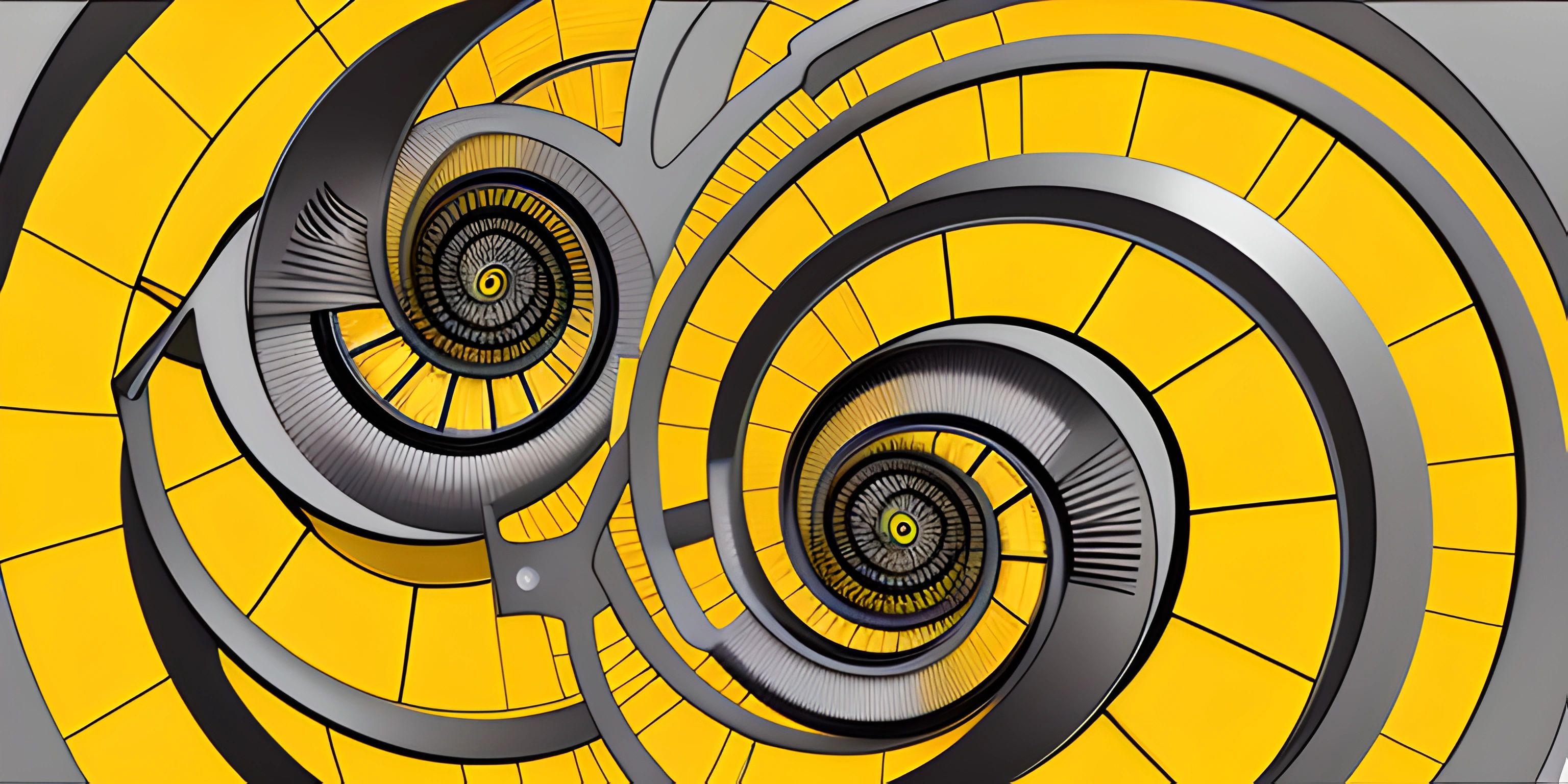
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of the Fibonacci sequence—a mesmerizing series of numbers found in nature, mathematics, and even art. In this article, we'll dive into what the Fibonacci sequence is, its significance, and how to generate it using different programming techniques.
Unraveling the Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, starting from 0 and 1. The sequence goes like this: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on.
What makes the Fibonacci sequence so fascinating is that it appears in various aspects of our lives, from the spiral arrangement of leaves on plants to the growth patterns of rabbit populations. You might even find it in the structure of some famous artworks!
Generating the Fibonacci Sequence Using Programming
There are several ways to generate the Fibonacci sequence using programming, and we'll explore two popular methods: recursion and iteration. We'll be using Python as our programming language of choice, but you can apply these concepts to any other language you're familiar with.
Recursive Method
Recursion is a technique where a function calls itself to solve a problem. In the case of the Fibonacci sequence, we can define a recursive function that returns the nth Fibonacci number as follows:
def fibonacci_recursive(n): if n <= 1: return n else: return fibonacci_recursive(n - 1) + fibonacci_recursive(n - 2)
Now, let's say we want to find the 9th Fibonacci number. We just need to call the function with the appropriate parameter:
result = fibonacci_recursive(9) print("The 9th Fibonacci number is:", result) # Output: The 9th Fibonacci number is: 34
While the recursive method might look elegant, it's not very efficient. It ends up recalculating the same Fibonacci numbers multiple times, leading to a lot of wasted computation power.
Iterative Method
An alternative, and more efficient, approach is to use an iterative method. In this case, we'll use a loop to generate the Fibonacci sequence up to the desired nth number:
def fibonacci_iterative(n): if n <= 1: return n a, b = 0, 1 for _ in range(2, n + 1): a, b = b, a + b return b
Again, let's find the 9th Fibonacci number using this function:
result = fibonacci_iterative(9) print("The 9th Fibonacci number is:", result) # Output: The 9th Fibonacci number is: 34
The iterative method is much more efficient than the recursive one, as it doesn't need to recalculate the same numbers repeatedly.
Conclusion
The Fibonacci sequence is a captivating series of numbers that holds significance in various fields. By learning different programming techniques, such as recursion and iteration, you can efficiently generate the sequence and explore its wonder. So go ahead, dive into the mesmerizing world of Fibonacci, and unleash the beauty of mathematics and computing.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is the Fibonacci sequence?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. It is named after Leonardo Fibonacci, an Italian mathematician who introduced this sequence to Western mathematics. The sequence goes like this: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on.
Why is the Fibonacci sequence significant in mathematics and computing?
The Fibonacci sequence has many interesting properties and appears in many different areas of mathematics and nature. Some examples include the golden ratio, which is closely related to the Fibonacci sequence, and the appearance of Fibonacci numbers in the growth patterns of plants. In computing, the sequence is used in algorithms, data structures, and even as a basis for some programming exercises and coding challenges.
How can I generate the Fibonacci sequence using programming?
You can generate the Fibonacci sequence using various programming languages and techniques. Here's a simple Python example using recursion:
def fibonacci(n): if n <= 1: return n else: return fibonacci(n - 1) + fibonacci(n - 2) for i in range(10): print(fibonacci(i))
Are there more efficient ways to generate the Fibonacci sequence in programming?
Yes, there are more efficient ways to generate the Fibonacci sequence, such as using iteration or memoization. Here's an example using iteration in Python:
def fibonacci(n): a, b = 0, 1 for _ in range(n): a, b = b, a + b return a for i in range(10): print(fibonacci(i))
Can the Fibonacci sequence be generated using different programming languages?
Absolutely! The Fibonacci sequence can be generated using any programming language, such as Java, C++, JavaScript, Ruby, and many others. The implementation might differ slightly depending on the language, but the underlying logic remains the same.