Java Basic Syntax
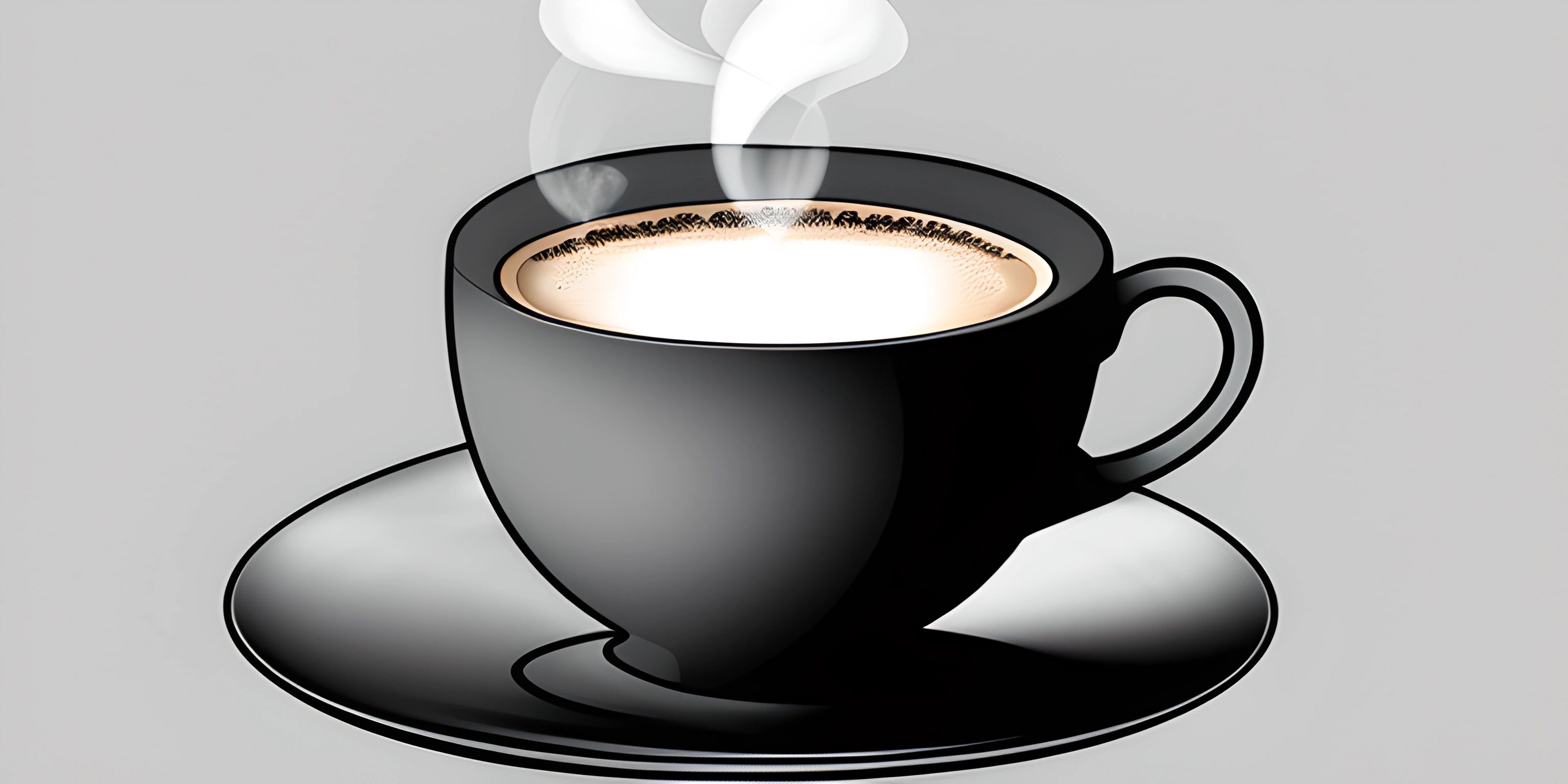
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Learning Java, or any programming language for that matter, requires getting familiar with its basic syntax and structure. Java is a popular, object-oriented, high-level programming language that is relatively easy to learn, but has some unique syntax rules. Let's dive into Java's basic syntax and structure to provide a solid foundation for your Java programming journey.
Java Program Structure
A Java program typically consists of one or more classes, each containing methods (functions) and variables (data). The most basic Java program has a single class with a main
method, which serves as the entry point for the program execution.
Classes
In Java, everything is organized within classes. A class is a blueprint for creating objects, which are instances of that class. A class can contain variables for storing data and methods for performing actions. Here's a simple example of a Java class:
public class HelloWorld { // Class content goes here }
In this example, we've created a class named HelloWorld
. The public
keyword signifies that this class is accessible from anywhere in the code.
Methods
Methods are blocks of code that perform specific tasks. In Java, every program must have a main
method, which is the starting point for its execution. The main
method has a specific signature that includes the public
, static
, and void
keywords, and accepts a single argument: a String array (String[] args
).
Here's an example of a basic main
method in Java:
public class HelloWorld { public static void main(String[] args) { // Method content goes here } }
In this example, we've added a main
method to our HelloWorld
class. The public
keyword means the method is accessible from anywhere in the code, while static
means it belongs to the class itself, rather than an instance of the class. The void
keyword indicates that the method doesn't return any value.
Statements
Java code is composed of statements. A statement is a single line of code that performs an action, such as declaring a variable or calling a method. Statements must be terminated with a semicolon (;
).
Let's add a statement to our main
method that prints "Hello, world!" to the console:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, world!"); } }
In this example, we've added a statement inside the main
method that calls the println
method of the System.out
object. This statement prints the text "Hello, world!" followed by a newline to the console.
Basic Java Syntax Rules
Here are some important syntax rules to remember when writing Java code:
-
Case sensitivity: Java is case-sensitive, meaning that
hello
andHello
are considered different identifiers. -
Class names: Class names should start with a capital letter and follow the camel-case convention (e.g.,
MyFirstClass
). -
Method names: Method names should start with a lowercase letter and also follow the camel-case convention (e.g.,
myFirstMethod
). -
File names: Java source code files must have the same name as the public class they contain, and the file extension must be
.java
(e.g.,HelloWorld.java
). -
Indentation: Although Java doesn't enforce a specific indentation style, it's recommended to use four spaces for each level of indentation for readability.
With these basic syntax rules and understanding of Java program structure, you're well on your way to becoming a proficient Java programmer. Remember, practice makes perfect, so keep coding and exploring new concepts to build your Java skills.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).