Creating Shapes with Py5
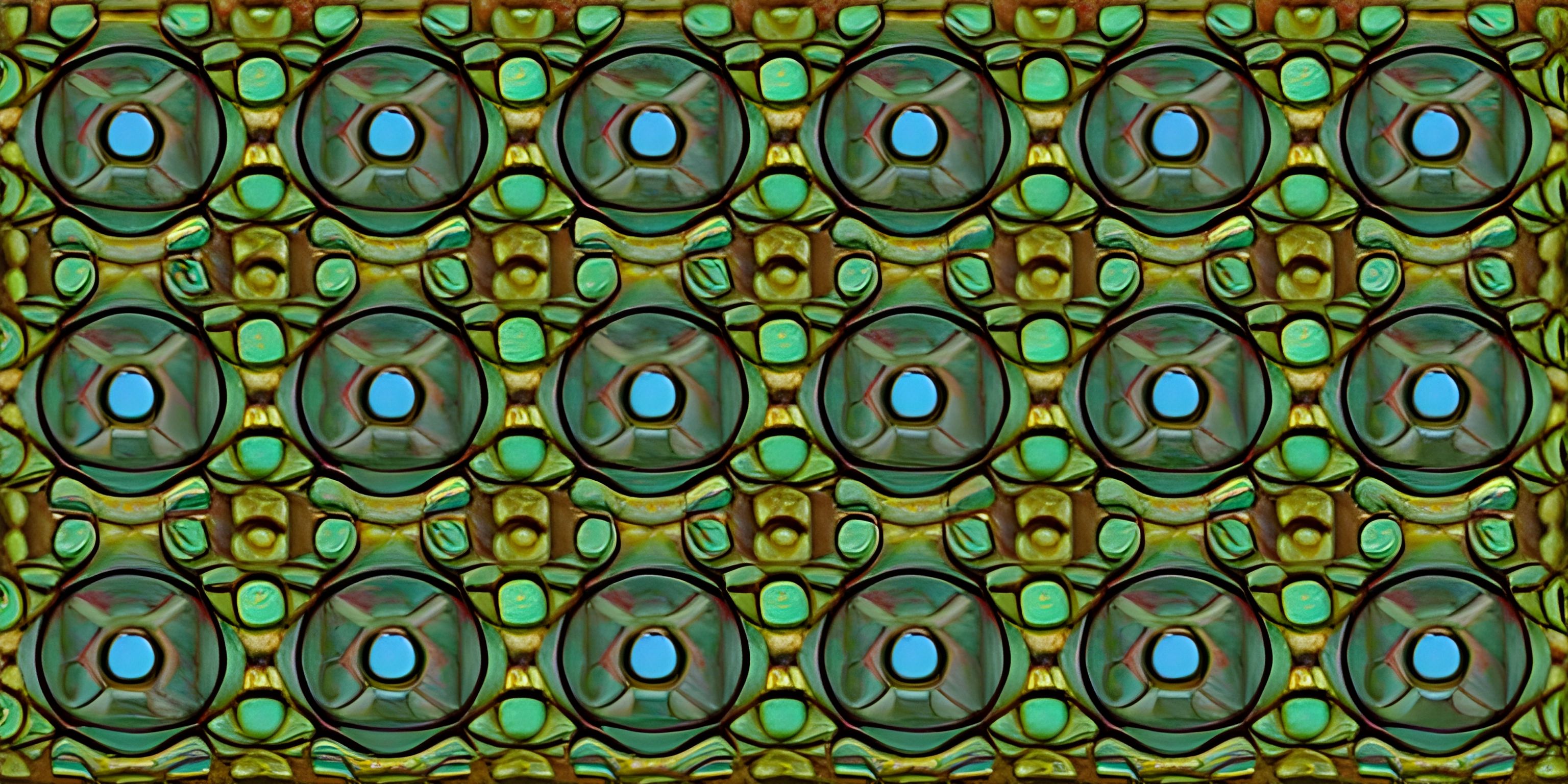
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ready to make some cool shapes using Python? Imagine wielding a magic wand that conjures up circles, squares, and intricate polygons just with a few lines of code. Well, with the Py5 library, you can do just that! Let's dive into the fascinating world of shapes and see how this library can turn your screen into a canvas.
Getting Started with Py5
First things first, we need to get Py5 installed and ready to go. You can easily install Py5 using pip:
pip install py5
Once installed, let's import it and set up a basic sketch. Think of this as setting up your canvas:
import py5 def setup(): py5.size(400, 400) # Set the size of the canvas def draw(): py5.background(255) # Set the background color to white draw_shapes() # Call our custom function to draw shapes def draw_shapes(): pass # We will fill this in soon py5.run_sketch()
Here, we have the setup
function to initialize our canvas and the draw
function, which continuously updates the display. The draw_shapes
function is where we'll be creating our magic.
Drawing Basic Shapes
Let's start with some basic shapes. Py5 makes it easy to draw shapes like rectangles, ellipses, and lines.
Rectangles
To draw a rectangle, use the rect
function. Here's how to draw a simple rectangle at position (50, 50)
with a width of 100
and height of 50
:
def draw_shapes(): py5.rect(50, 50, 100, 50)
Ellipses
Drawing an ellipse is equally straightforward. Use the ellipse
function to draw an ellipse at position (200, 200)
with a width and height of 80
:
def draw_shapes(): py5.ellipse(200, 200, 80, 80)
Lines
How about a line? The line
function draws a line from one point to another. Here's a line from (300, 300)
to (350, 350)
:
def draw_shapes(): py5.line(300, 300, 350, 350)
Adding Colors
Shapes look more interesting with colors. You can set the fill color for shapes and the stroke color for their outlines.
Fill Color
Use the fill
function to set the color inside the shapes. Here's a red rectangle:
def draw_shapes(): py5.fill(255, 0, 0) py5.rect(50, 50, 100, 50)
Stroke Color
To set the outline color, use the stroke
function. Let's set a blue outline for our ellipse:
def draw_shapes(): py5.stroke(0, 0, 255) py5.ellipse(200, 200, 80, 80)
Creating Complex Shapes
Now that we have the basics down, let's move on to more complex shapes like polygons. Py5 provides a beginShape
and endShape
function to define vertices of polygons.
Polygons
Here's an example of how to draw a triangle:
def draw_shapes(): py5.begin_shape() py5.vertex(150, 100) py5.vertex(200, 200) py5.vertex(100, 200) py5.end_shape(py5.CLOSE)
In this example, begin_shape
starts the shape, vertex
adds points, and end_shape(py5.CLOSE)
closes the shape by connecting the last point to the first.
Stars
How about a star? By strategically placing vertices, we can create a star shape:
def draw_shapes(): py5.begin_shape() py5.vertex(200, 50) py5.vertex(217, 90) py5.vertex(260, 90) py5.vertex(227, 120) py5.vertex(240, 160) py5.vertex(200, 140) py5.vertex(160, 160) py5.vertex(173, 120) py5.vertex(140, 90) py5.vertex(183, 90) py5.end_shape(py5.CLOSE)
Play around with the vertex positions to create different star shapes and sizes.
Rotating and Scaling Shapes
To add more dynamic effects, you can rotate and scale shapes. Py5 offers rotate
and scale
functions for this purpose.
Rotating
To rotate a shape, use the rotate
function. This rotates the shape by a specified angle (in radians):
def draw_shapes(): py5.push_matrix() # Save the current transformation state py5.translate(200, 200) # Move the origin to the center of the ellipse py5.rotate(py5.PI / 4) # Rotate by 45 degrees py5.ellipse(0, 0, 80, 80) # Draw the ellipse py5.pop_matrix() # Restore the original transformation state
Scaling
Similarly, use the scale
function to resize shapes:
def draw_shapes(): py5.push_matrix() py5.translate(200, 200) py5.scale(1.5) # Scale by 150% py5.ellipse(0, 0, 80, 80) py5.pop_matrix()
Conclusion
With Py5, creating basic and complex shapes is a breeze. From simple rectangles and circles to intricate stars and polygons, the library provides a set of intuitive functions that make drawing fun and easy. Experiment with different shapes, colors, rotations, and scales to create your unique artwork!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Getting Complex (psst, it's free!).
FAQ
How do I install Py5?
You can easily install Py5 using pip with the command pip install py5
.
What is the function to draw a rectangle in Py5?
Use the rect
function to draw a rectangle. For example, py5.rect(50, 50, 100, 50)
draws a rectangle at position (50, 50)
with a width of 100
and height of 50
.
How can I add color to my shapes in Py5?
Use the fill
function to set the fill color and the stroke
function to set the outline color. For example, py5.fill(255, 0, 0)
sets the fill color to red.
How do I draw a polygon in Py5?
Use begin_shape
to start the shape, vertex
to add points, and end_shape(py5.CLOSE)
to close the shape. For example, a triangle can be drawn using three vertex
calls.
Can I rotate and scale shapes in Py5?
Yes, you can use the rotate
function to rotate shapes and the scale
function to resize them. Use push_matrix
and pop_matrix
to save and restore the transformation state.