React Context API
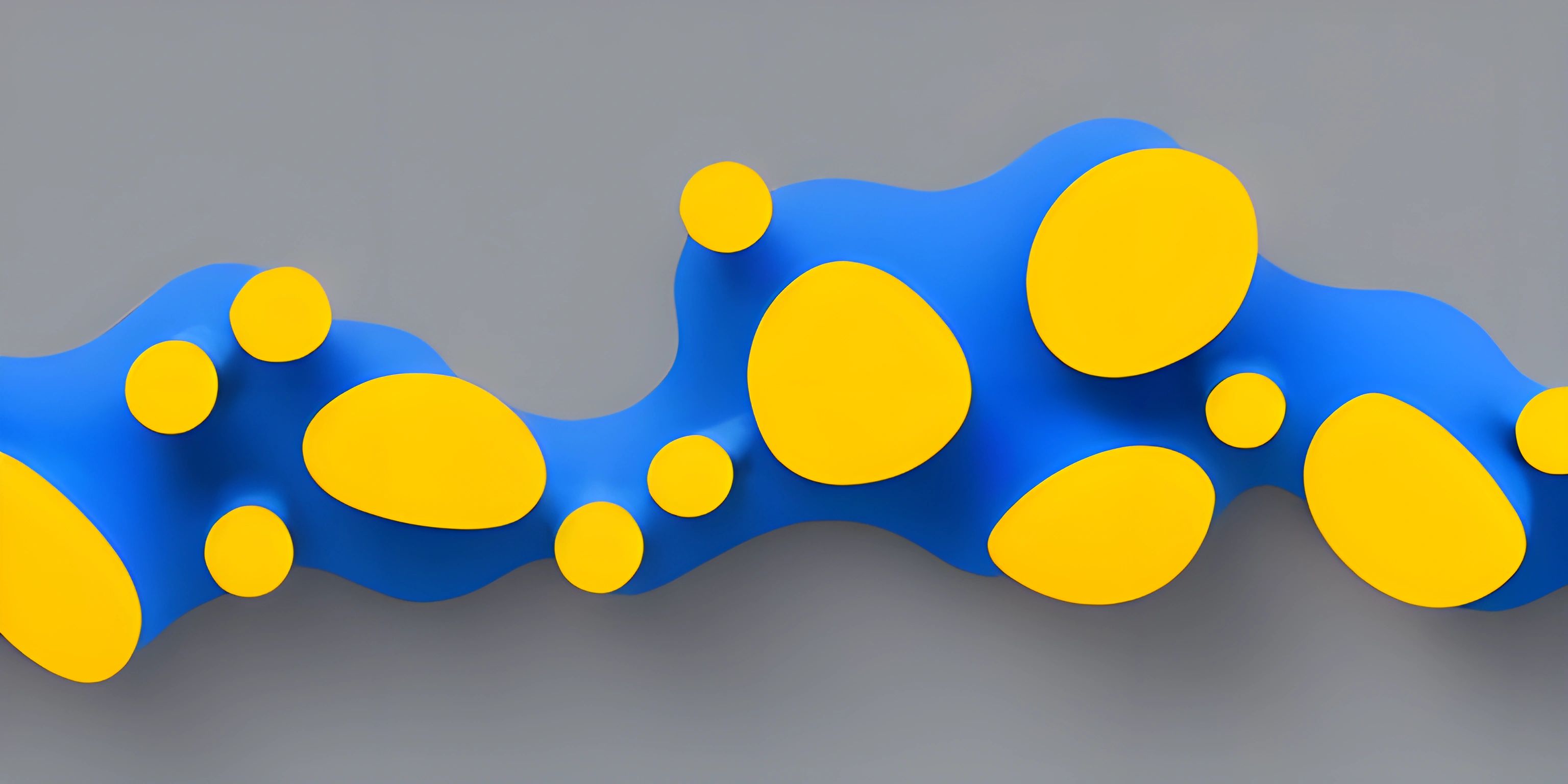
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Managing state in a React application can sometimes feel like a game of pass-the-parcel. You pass data down through layers of components, and in turn, pass callbacks to update the data back up. But worry not! The React Context API offers a way to simplify state management and avoid prop drilling in your application.
What is the React Context API?
The React Context API is a built-in feature that allows you to create a global state and share it with components that need access to it. By creating a context, you can avoid passing props through intermediate components that don't care about them.
Creating a Context
To create a context, you'll use the React.createContext()
function. This function returns an object with two components: a Provider
and a Consumer
. The Provider
is responsible for defining the value of the context, while the Consumer
is used to access the value within child components.
Here's an example of creating a context:
import React from "react"; const ThemeContext = React.createContext("light"); // Default value is "light" export default ThemeContext;
In this example, we've created a ThemeContext
with a default value of "light"
.
Using the Provider
The Provider
component is used to wrap the part of your app where you want to provide the context value. It takes a single prop, value
, which is the data you want to share with the components inside the provider.
Here's an example of using the Provider
:
import React from "react"; import ThemeContext from "./ThemeContext"; import AppContent from "./AppContent"; function App() { const [theme, setTheme] = React.useState("light"); return ( <ThemeContext.Provider value={theme}> <AppContent /> </ThemeContext.Provider> ); } export default App;
In this example, we're using the ThemeContext.Provider
to wrap our AppContent
component and provide the theme
value.
Accessing the Context Value
There are two ways to consume the context value in a child component: using the Consumer
component or the useContext()
hook.
Using the Consumer Component
The Consumer
component is a render prop component that takes a child function with the context value as its argument. Here's an example:
import React from "react"; import ThemeContext from "./ThemeContext"; function ThemedButton() { return ( <ThemeContext.Consumer> {(theme) => <button className={`btn-${theme}`}>Click me!</button>} </ThemeContext.Consumer> ); } export default ThemedButton;
Using the useContext() Hook
If you prefer using hooks, you can access the context value using the useContext()
hook. It takes the context as an argument and returns the context value:
import React, { useContext } from "react"; import ThemeContext from "./ThemeContext"; function ThemedButton() { const theme = useContext(ThemeContext); return <button className={`btn-${theme}`}>Click me!</button>; } export default ThemedButton;
And there you have it! You've now learned how to use the React Context API to simplify state management in your React applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the React Context API?
The React Context API is a built-in feature in React that allows you to create a global state and share it with components that need access to it. This helps to avoid prop drilling and simplifies state management in React applications.
How do you create a context?
To create a context, you use the React.createContext()
function. This function returns an object with two components: a Provider
and a Consumer
. The Provider
is responsible for defining the value of the context, while the Consumer
is used to access the value within child components.
How do you access the context value in a child component?
There are two ways to consume the context value in a child component: using the Consumer
component, which takes a child function with the context value as its argument, or using the useContext()
hook, which takes the context as an argument and returns the context value.