Introduction to React and Its Components
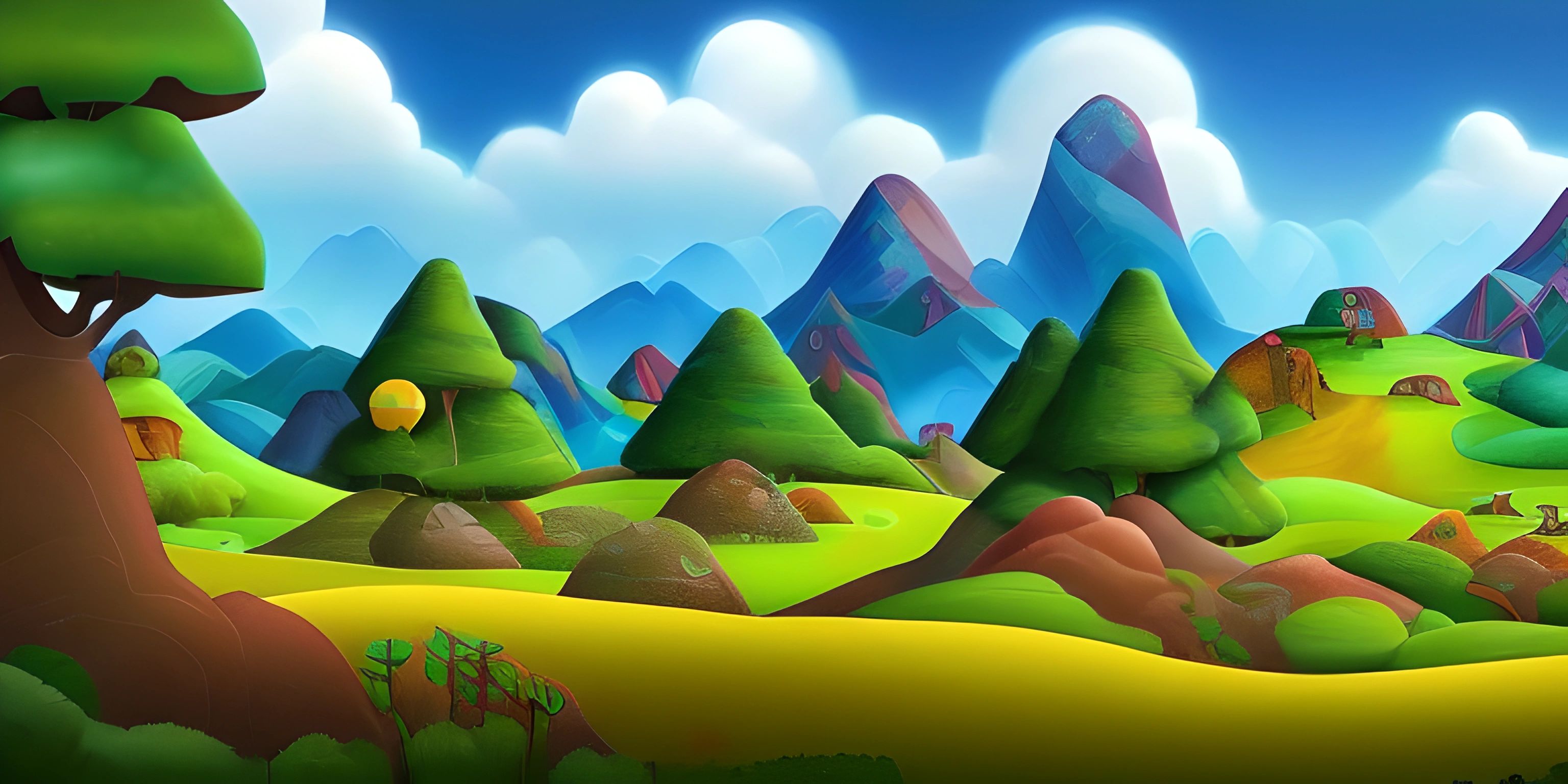
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React is a popular JavaScript library that makes it easy to build interactive user interfaces (UIs). Developed by Facebook, React is often used in combination with other technologies to create powerful Single Page Applications (SPAs). In the wonderful world of React, components are the building blocks that help you construct a sleek and efficient UI.
Components
In React, components are self-contained, reusable pieces of code that return a portion of the UI. Components can be as simple as a button or as complex as an entire page layout. They can be written as plain JavaScript functions or using the more advanced ES6 class syntax.
JSX
React components are written using a special syntax called JSX, which allows you to mix HTML and JavaScript in a single file. JSX might look strange at first, but it's actually quite intuitive once you get the hang of it. Here's a simple example:
function WelcomeMessage() { return ( <div> <h1>Hello, World!</h1> <p>Welcome to React.</p> </div> ); }
This WelcomeMessage
component, when rendered, will display a heading and a paragraph with a welcome message. You can think of JSX as a way of describing the UI structure with a syntax that closely resembles HTML.
State and Props
Two key concepts in React are state and props. State is the internal data of a component, while props are the external data passed to it from its parent component.
When a component's state or props change, React automatically re-renders the component, ensuring the UI is always up to date. This makes it easy to create dynamic and interactive UIs without having to manually update the DOM.
State
State is managed within a component and can be initialized and updated using the useState
hook in functional components or the setState
method in class components. Here's a simple example using the useState
hook:
import React, { useState } from "react"; function Counter() { const [count, setCount] = useState(0); return ( <div> <button onClick={() => setCount(count + 1)}>Click me</button> <p>Clicked {count} times</p> </div> ); }
In this example, we've created a Counter
component that manages its own state, allowing it to keep track of how many times the button has been clicked.
Props
Props, short for properties, are used to pass data from a parent component to its children. Here's a simple example of passing props:
function Greeting({ name }) { return <p>Hello, {name}!</p>; } function App() { return ( <div> <Greeting name="Alice" /> <Greeting name="Bob" /> </div> ); }
In this example, the App
component renders two Greeting
components, each with a different name
prop. The Greeting
component then displays a personalized message based on the name
prop it receives.
Conclusion
React is a powerful and versatile library that has taken the web development world by storm. By understanding its core concepts, such as components, JSX, state, and props, you can unlock the full potential of React to create dynamic and interactive user interfaces. Now that you've been introduced to React, it's time to dive deeper and start building awesome web applications!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What is React and why is it popular?
React is an open-source JavaScript library developed by Facebook for building modern, interactive web applications. It's popular due to its robust component-based architecture, which promotes reusability and maintainable code. Additionally, React efficiently updates and renders components, resulting in improved performance and a smooth user experience.
Can you explain the basic structure of a React component?
A React component is a reusable piece of UI that can contain its own state and logic. The basic structure of a React component is a JavaScript class or function that extends React.Component
. It usually has a render
method that returns JSX (a syntax extension that looks similar to HTML) to describe what the component should look like. Here's a simple example of a functional React component:
import React from 'react'; const HelloWorld = () => { return ( <div> <h1>Hello, World!</h1> </div> ); }; export default HelloWorld;
How do I use a React component in another component?
To use a React component in another component, first import the desired component and then include it as a JSX tag within the parent component's JSX. Here's an example of how to use the HelloWorld
component we defined earlier inside another component:
import React from 'react'; import HelloWorld from './HelloWorld'; const App = () => { return ( <div> <HelloWorld /> </div> ); }; export default App;
What are props in React and how do I use them?
Props (short for "properties") are a way to pass data from a parent component to a child component. To use props, define the desired attributes on the child component JSX tag in the parent component, and then access those attributes as properties of the props
object in the child component. Here's an example:
import React from 'react'; const Greeting = (props) => { return <h1>Hello, {props.name}!</h1>; }; const App = () => { return ( <div> <Greeting name="John" /> </div> ); }; export default App;
In this example, we're passing a name
prop from the App
component to the Greeting
component. The Greeting
component then uses the name
prop to display a personalized greeting.