Breaking Down React Components
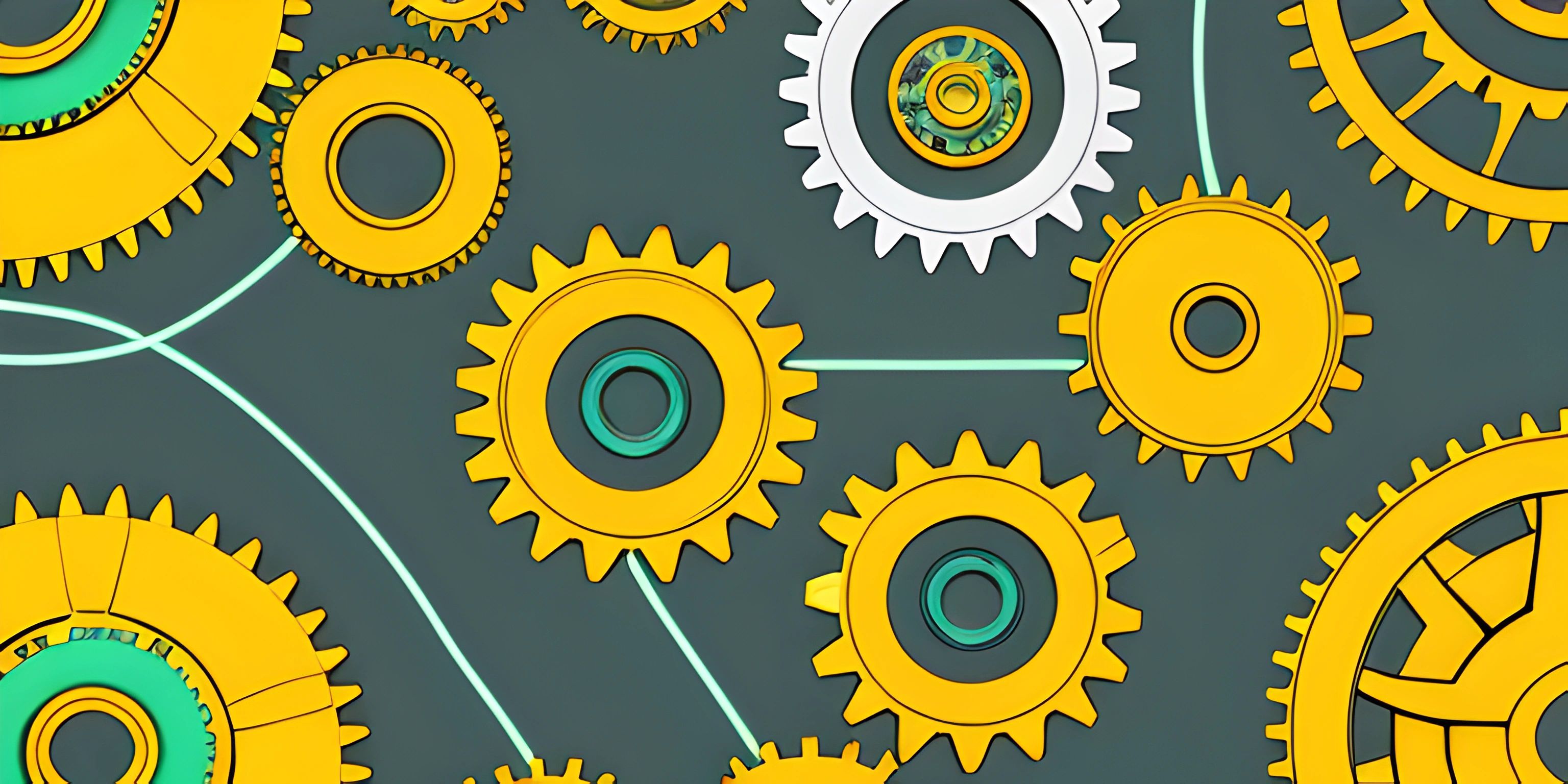
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Are you ready to get your hands dirty with some React components? Let's do it! React components are the building blocks of a React application, and understanding them is crucial for any aspiring React developer. Here's what we'll cover:
What is a React Component?
React components are reusable, self-contained pieces of code that represent a part of the user interface (UI). Each component is responsible for rendering a specific part of the UI and managing its own state and behavior.
Think of React components as LEGO bricks. You can snap them together to build complex structures (your application's UI), but each brick is a self-contained unit with a specific purpose.
Types of React Components
There are two main types of React components: class components and functional components.
Class Components
Class components are defined using ES6 classes and extend the React.Component
class. They are capable of managing their own state and have access to React lifecycle methods.
Here's a simple example of a class component:
import React, { Component } from "react"; class Greeting extends Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
Functional Components
Functional components, on the other hand, are simple JavaScript functions that receive props as an argument and return a React element. They are stateless by nature but can use React Hooks to manage state and side effects.
Here's the same Greeting component as a functional component:
import React from "react"; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
State and Props
React components can receive data from their parent components through props (short for properties). Props are read-only and cannot be modified by the component. If a component needs to manage its own data, it can use state.
State is mutable, and changes to the state trigger a re-render of the component. In class components, state is managed using the setState
method, while functional components use the useState
hook.
Component Lifecycle and Hooks
React components go through a series of phases, such as mounting, updating, and unmounting. Class components have access to lifecycle methods, like componentDidMount
, componentDidUpdate
, and componentWillUnmount
, allowing you to perform side effects at specific points in the component's life.
Functional components can achieve the same effect using React Hooks, such as useEffect
, which is more flexible and can cover multiple lifecycle events.
Best Practices
When working with React components, it's essential to follow best practices for better maintainability, readability, and performance. Some of these best practices include:
- Favor functional components over class components, as they are generally more concise and easier to read.
- Keep components small and focused on a single responsibility.
- Use React.memo to optimize functional components that re-render unnecessarily.
- Be mindful of performance and use techniques like lazy loading and code splitting when needed.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What are React components?
React components are reusable, self-contained pieces of code that represent a part of the user interface (UI) in a React application. They are responsible for rendering specific UI elements and managing their own state and behavior.
What are the two main types of React components?
The two main types of React components are class components and functional components. Class components are defined using ES6 classes and extend the React.Component class, while functional components are simple JavaScript functions that receive props as an argument and return a React element.
How do React components receive data from their parent components?
React components receive data from their parent components through props (short for properties). Props are read-only and cannot be modified by the component.
What is the difference between state and props in React components?
Props are read-only data passed down from parent components, while state is mutable data managed within the component. Changes to state trigger a re-render of the component.
What are some best practices when working with React components?
Best practices when working with React components include favoring functional components over class components, keeping components small and focused, optimizing functional components with React.memo, and being mindful of performance by using techniques like lazy loading and code splitting when needed.