React Props
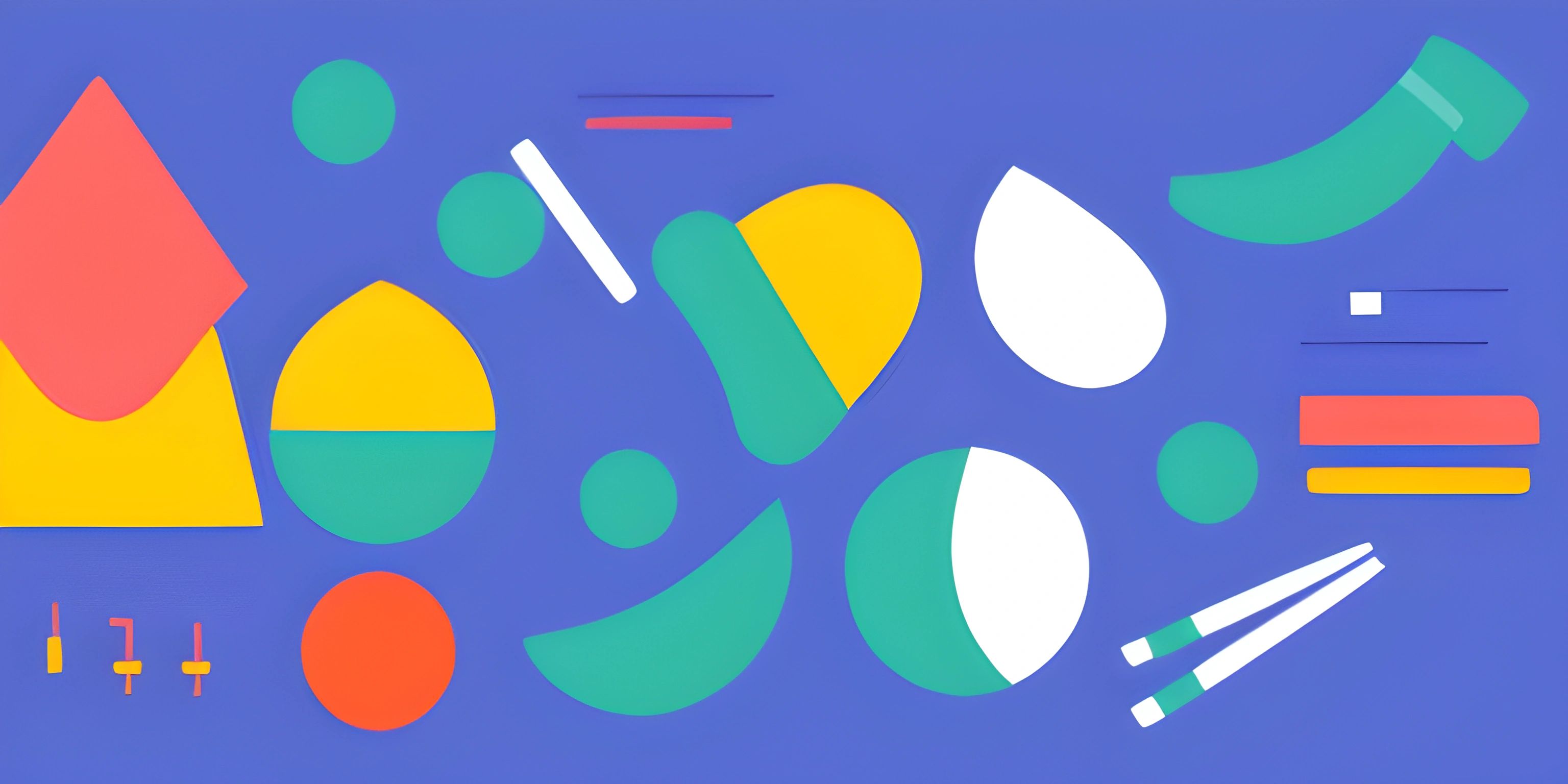
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of React, components are like the building blocks of your application. Often, you'll need to share data between these components. In React, we use props to pass data from parent to child components.
What are Props?
Props (short for properties) are the mechanism by which we pass data from a parent component to a child component in a React application. Think of props as a way of passing custom attributes to your components, similar to how you would pass attributes to HTML elements.
In a React component, props are received as an argument in the component's function. Let's see an example to understand how to use props:
import React from "react"; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return <Greeting name="John" />; } export default App;
In the example above, we have two components: Greeting
and App
. The App
component renders the Greeting
component and passes the name
prop with the value "John". The Greeting
component then receives this prop and displays the value within an <h1>
element.
Passing Multiple Props
You can pass multiple props to a child component by simply adding more attributes to the component. Here's an example:
import React from "react"; function UserInfo(props) { return ( <div> <h1>Name: {props.name}</h1> <p>Age: {props.age}</p> </div> ); } function App() { return <UserInfo name="John" age={30} />; } export default App;
In this example, the App
component renders the UserInfo
component and passes two props: name
and age
. The UserInfo
component then receives these props and displays the values within an <h1>
and a <p>
element, respectively.
Props are Read-Only
It's important to note that props should be treated as read-only. This means that you should never modify props directly within a component. Instead, if a component needs to change the value of a prop, it should use state and event handlers to manage the change.
For example, if you need to build a counter component that increments a value, you should use state to store the value and event handlers to handle the increment action.
Conclusion
Props are a fundamental concept in React, allowing you to pass data between components and build complex and dynamic applications. Remember that props are read-only, so any changes to prop values should be managed through state and event handlers. With a solid understanding of React props, you'll be well-equipped to develop flexible and reusable components that make your applications shine.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What are React props?
React props are short for "properties" and are a way to pass data from one component to another within a React application. Props allow you to share data and functionality between components, making your code more modular and maintainable.
How do I pass data to a component using props?
To pass data to a component using props, you can add attributes to the component's JSX tag, just like you would with HTML attributes. Here's an example of passing a name
prop to a Greeting
component:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return <Greeting name="John" />; } ReactDOM.render(<App />, document.getElementById('root'));
In this example, the Greeting
component receives a name
prop, which is set to "John", and displays it in the rendered output.
How can I pass multiple props to a component?
You can pass multiple props to a component by adding multiple attributes to the component's JSX tag. Here's an example of passing both a firstName
and a lastName
prop to a FullName
component:
function FullName(props) { return <h1>{props.firstName} {props.lastName}</h1>; } function App() { return <FullName firstName="John" lastName="Doe" />; } ReactDOM.render(<App />, document.getElementById('root'));
In this example, the FullName
component receives both a firstName
and a lastName
prop and displays them in the rendered output.
Can I pass functions as props?
Yes, you can pass functions as props to components, allowing you to share functionality between components. Here's an example of passing a handleClick
function as a prop to a Button
component:
function Button(props) { return <button onClick={props.handleClick}>Click me!</button>; } function App() { function handleClick() { alert("Button clicked!"); } return <Button handleClick={handleClick} />; } ReactDOM.render(<App />, document.getElementById('root'));
In this example, the Button
component receives a handleClick
function as a prop and uses it as an event handler for the onClick
event.